一 Mybatis_springboot
MyBatis 是一个流行的持久层框架,可以与 Spring Boot 无缝集成。下面是如何在 Spring Boot 项目中使用 MyBatis 的基本步骤。
1. 创建 Spring Boot 项目
你可以使用 Spring Initializr 创建一个新的 Spring Boot 项目。选择以下依赖项:
- Spring Web
- MyBatis Framework
- 数据库(如 H2、MySQL、PostgreSQL 等)
2. 添加依赖
如果你已经创建了项目,可以在 pom.xml
(对于 Maven 项目)中添加 MyBatis 和数据库的依赖。例如对于 MySQL:
<dependencies>
<!-- mybatis坐标 -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.2</version>
</dependency>
<!-- mysql -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.28</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
3. 数据库配置
在 application.yml
或 application.properties
文件中配置 数据库连接信息。例如,如果使用 MySQL,可以这么配置:
#数据源
spring:
datasource:
username: root
password: 123456
url: jdbc:mysql://localhost:3306/myschool?serverTimezone=GMT
driver-class-name: com.mysql.cj.jdbc.Driver
4. 创建数据模型
创建一个 Java 类,表示数据库表的结构。例如:
package com.xn.mybatis_springboot.pojo;
/**
* @author 许娜
* @version 1.0
* @since 2024/8/13
*/
public class Account {
private int aid;
private String aname;
private int amoney;
@Override
public String toString() {
return "Account{" +
"aid=" + aid +
", aname='" + aname + '\'' +
", amoney=" + amoney +
'}';
}
public Account(int aid, String aname, int amoney) {
this.aid = aid;
this.aname = aname;
this.amoney = amoney;
}
public Account() {
}
public int getAid() {
return aid;
}
public void setAid(int aid) {
this.aid = aid;
}
public String getAname() {
return aname;
}
public void setAname(String aname) {
this.aname = aname;
}
public int getAmoney() {
return amoney;
}
public void setAmoney(int amoney) {
this.amoney = amoney;
}
}
5. 创建 Mapper 接口
创建 MyBatis 的 Mapper 接口,用于定义 SQL 操作。例如:
//@Mapper//注册注入一个mapper
public interface AccountMapper {
@Select("select * from account")
public List<Account> findAll();
}
或
@SpringBootApplication
@MapperScan(basePackages="com.xn.mybatis_springboot.mapper")//注册注入多个mapper(以包为单位)
public class MybatisSpringbootApplication {
public static void main(String[] args) {
SpringApplication.run(MybatisSpringbootApplication.class, args);
}
}
6.测试
@SpringBootTest
class MybatisSpringbootApplicationTests {
@Autowired(required=false)
AccountMapper accountMapper;
@Test
void contextLoads() {
List<Account> all=accountMapper.findAll();
for (int i=0;i<all.size();i++){
Account account=all.get(i);
System.out.println(account);
}
}
}
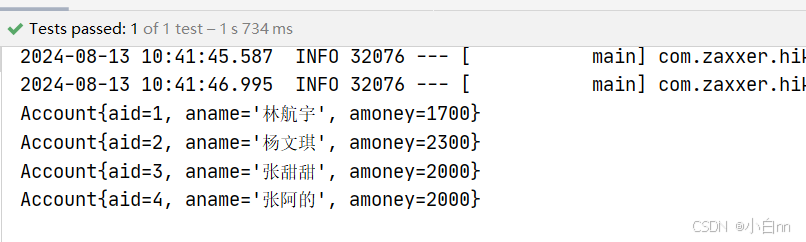
二 mybatis-plus
1.坐标
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.1.1</version>
</dependency>
注意:mp坐标添加后,mybatis坐标移除
<!-- mybatisPlus坐标 -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.1.1</version>
</dependency>
<!-- mybatis坐标 -->
<!-- <dependency>-->
<!-- <groupId>org.mybatis.spring.boot</groupId>-->
<!-- <artifactId>mybatis-spring-boot-starter</artifactId>-->
<!-- <version>2.2.2</version>-->
<!-- </dependency>-->
<!-- mysql -->
2.编写注解配置实体类与关系表映射关系(truncate清空表以及主键)
@TableName(value = "关联表名称")=========================》修饰在类
@TableField(value = "关联字段名称")======================》修饰在属性
exist = "忽略字段"
@TableId(type="指定主键生成策略,默认雪花算法")=============》修饰在属性
AUTO(0),
NONE(1),
INPUT(2),
ASSIGN_ID(3),
ASSIGN_UUID(4);
@TableName("account")
public class Account {
@TableId(value = "aid",type= IdType.AUTO)
private int aid;
@TableField("aname")
private String aname;
@TableField("amoney")
private int amoney;
}
3.使用
BaseMapper===========》公共的数据访问层
IService/ServiceImp==》公共的业务层
4.配置yml文件
#数据源
spring:
datasource:
username: root
password: 123456
url: jdbc:mysql://localhost:3306/myschool?serverTimezone=GMT
driver-class-name: com.mysql.cj.jdbc.Driver
mybatis:
mapper-locations: mappers/*.xml
mybatis-plus:
configuration:
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
5.测试代码使用
(1)新增
@SpringBootTest
public class Test01 {
@Autowired(required = false)
AccountMapper accountMapper;
//新增
@Test
public void show1(){
Account account = new Account("夕夕",2000);
int row = accountMapper.insert(account);
System.out.println("主键回填id:"+account.getAid());
System.out.println("影响行数:"+row);
}
}
(2) 修改
@SpringBootTest
public class Test01 {
@Autowired(required = false)
AccountMapper mapper;
//修改ID
@Test
public void test02()throws Exception{
Account account = new Account(3,"发发",5000,1,"干饭");
int row = mapper.updateById(account);
System.out.println("影响行数:"+row);
}
//
// //修改Name
@Test
public void test03()throws Exception{
//1.修改数据
Account account = new Account();
account.setAhobby("打架");
//2.创建条件
QueryWrapper<Account> wrapper = new QueryWrapper<Account>();
wrapper.eq("aname","毛毛");
mapper.update(account,wrapper);
}
}
(3) mp分页使用
注意:
1.page.setCurrent(2);当前页码从1开始
2.分页需要配置插件
3.mp坐标版本3.1.1不能使用过高版本
<!-- mybatisPlus坐标 -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.3</version>
</dependency>
@Configuration
public class MyBatisPlusConfig {
//注入mp拦截器
@Bean
public MybatisPlusInterceptor mybatisPlusInterceptor(){
//1.实例化拦截器
MybatisPlusInterceptor mybatisPlusInterceptor = new MybatisPlusInterceptor();
//2.分页拦截器
mybatisPlusInterceptor.addInnerInterceptor(new PaginationInnerInterceptor());
return mybatisPlusInterceptor;
}
}
/**
* mp分页使用
* 注意:
* 1.page.setCurrent(2);当前页码从1开始
* 2.分页需要配置插件
* 3.mp坐标版本3.1.1不能使用过高版本
* */
@Test
public void test08()throws Exception{
//1.定义分页规则
Page<Account> page = new Page<Account>();
page.setSize(3);//每页记录数
page.setCurrent(2);//当前页码
//2.查询条件(可选)
QueryWrapper queryWrapper = new QueryWrapper();
queryWrapper.eq("ahobby","唱歌");
IPage<Account> iPage = mapper.selectPage(page,null);
List<Account> list = iPage.getRecords();//分页结果
System.out.println("总记录数:"+iPage.getTotal());
System.out.println("总记页数:"+iPage.getPages());
for (int i = 0; i < list.size(); i++) {
Account account = list.get(i);
System.out.println(account);
}
}
(4)查询
普通查询
//查询ID
@Test
public void test04()throws Exception{
Account account = mapper.selectById(5);
System.out.println(account);
}
//
//查询IDS
@Test
public void test05()throws Exception{
List<Account> list = mapper.selectBatchIds(Arrays.asList(5,1,3));
for (int i = 0; i < list.size(); i++) {
Account account = list.get(i);
System.out.println(account);
}
}
QueryWrapper
QueryWrapper
是 MyBatis-Plus 中常用的一个工具类,用于构建 SQL 查询条件的方式。它提供了链式调用的方法来设置查询条件,使得代码更加简洁、易读。
//查询count
@Test
public void test06()throws Exception{
int count = mapper.selectCount(null);
System.out.println(count);
}
//查询list
@Test
public void test07()throws Exception{
QueryWrapper<Account> queryWrapper = new QueryWrapper();
// queryWrapper.eq("aage","11");
// queryWrapper.eq("ahobby","唱歌");
queryWrapper.eq("ahobby","唱歌").or().eq("aage","11");
List<Account> list = mapper.selectList(queryWrapper);
for (int i = 0; i < list.size(); i++) {
Account account = list.get(i);
System.out.println(account);
}
}
LambdaQueryWrapper
@Test
public void show1(){
//1.查询条件
LambdaQueryWrapper<Account> lambdaQueryWrapper = new LambdaQueryWrapper<Account>();
lambdaQueryWrapper.gt(Account::getAage,12);
//2.查询
List<Account> list = mapper.selectList(lambdaQueryWrapper);
for (int i = 0; i < list.size(); i++) {
Account account = list.get(i);
System.out.println(account);
}
}
模拟动态查询
//模拟动态查询1
@Test
public void show2(){
//1.前端发送来的数据
Integer num1 = null;
Integer num2 = 15;
//1.查询条件
LambdaQueryWrapper<Account> lambdaQueryWrapper = new LambdaQueryWrapper<Account>();
//2.判断
if(null != num2){
lambdaQueryWrapper.lt(Account::getAage,num2);
}
if(null != num1){
lambdaQueryWrapper.gt(Account::getAage,num1);
}
//3.查询
List<Account> list = mapper.selectList(lambdaQueryWrapper);
for (int i = 0; i < list.size(); i++) {
Account account = list.get(i);
System.out.println(account);
}
}
//模拟动态查询2
@Test
public void show3(){
//1.前端发送来的数据
Integer num1 = null;
Integer num2 = 15;
//1.查询条件
LambdaQueryWrapper<Account> lambdaQueryWrapper = new LambdaQueryWrapper<Account>();
//2.判断
lambdaQueryWrapper.lt(null != num2,Account::getAage,num2);
lambdaQueryWrapper.gt(null != num1,Account::getAage,num1);
//3.查询
List<Account> list = mapper.selectList(lambdaQueryWrapper);
for (int i = 0; i < list.size(); i++) {
Account account = list.get(i);
System.out.println(account);
}
}
投影查询-字段查询
//投影查询-字段查询
@Test
public void show4() {
//1.条件
LambdaQueryWrapper<Account> lambdaQueryWrapper = new LambdaQueryWrapper<Account>();
lambdaQueryWrapper.select(Account::getAname,Account::getAhobby);
//2.查询
List<Account> list = mapper.selectList(lambdaQueryWrapper);
//4.遍历
for (int i = 0; i < list.size(); i++) {
Account account = list.get(i);
System.out.println(account);
}
}
(5)删除
逻辑删除:
为数据设置是否可用状态字段,删除时设置状态字段为不可用状态, 数据保留在数据库中,执行的是update操作
实现步骤:
步骤1:修改数据库表添加`deleted`列,比如`0`代表正常,`1`代表删除,可以在添加列的同时 设置其默认值为`0`正常。
步骤2:实体类添加属性以及注解 @TableLogic(value="0",delval="1") private Integer deleted; value为正常数据的值,delval为删除数据的值 逻辑删除
//查询delete
@Test
public void test09()throws Exception{
mapper.deleteById(4);
}
物理删除:
业务数据从数据库中丢弃,执行的是delete操作
//查询delete
@Test
public void test09()throws Exception{
mapper.deleteById(3);
}