目录
Mybatis
MyBatis是一款优秀的 持久层 框架,用于简化JDBC的开发。Mybatis框架,就是对原始的JDBC程序的封装。
JDBC: ( Java DataBase Connectivity ),就是使用Java语言操作关系型数据库的一套API。
MyBatis本是 Apache的一个开源项目iBatis,2010年这个项目由apache迁移到了google code,并
且改名为MyBatis 。2013年11月迁移到Github。
持久层:指的是就是数据访问层(dao),是用来操作数据库的。
框架:是一个半成品软件,是一套可重用的、通用的、软件基础代码模型。在框架的基础上进行软
件开发更加高效、规范、通用、可拓展。
使用Mybatis操作数据库,就是在Mybatis中编写SQL查询代码,发送给数据库执行,数据库执行后
返回结果。
Mybatis会把数据库执行的查询结果,使用实体类封装起来(一行记录对应一个实体类对象)
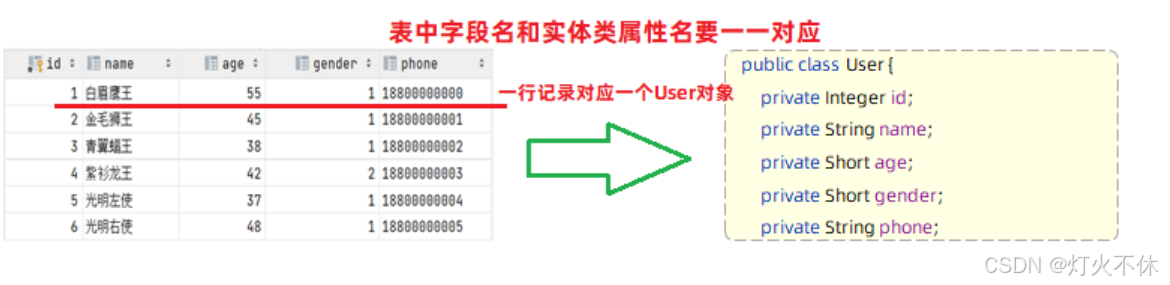
Mybatis入门操作步骤
1. 准备工作(创建springboot工程、数据库表user、实体类User)
2. 引入Mybatis的相关依赖,配置Mybatis(数据库连接信息)
3. 编写SQL语句(注解/XML)
1.准备工作
创建springboot工程
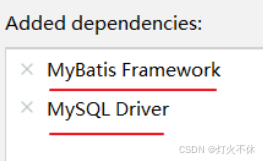
创建springboot时引入MyBatis Framework和MySQL Driver
在pom.xml文件中,自动导入了依赖
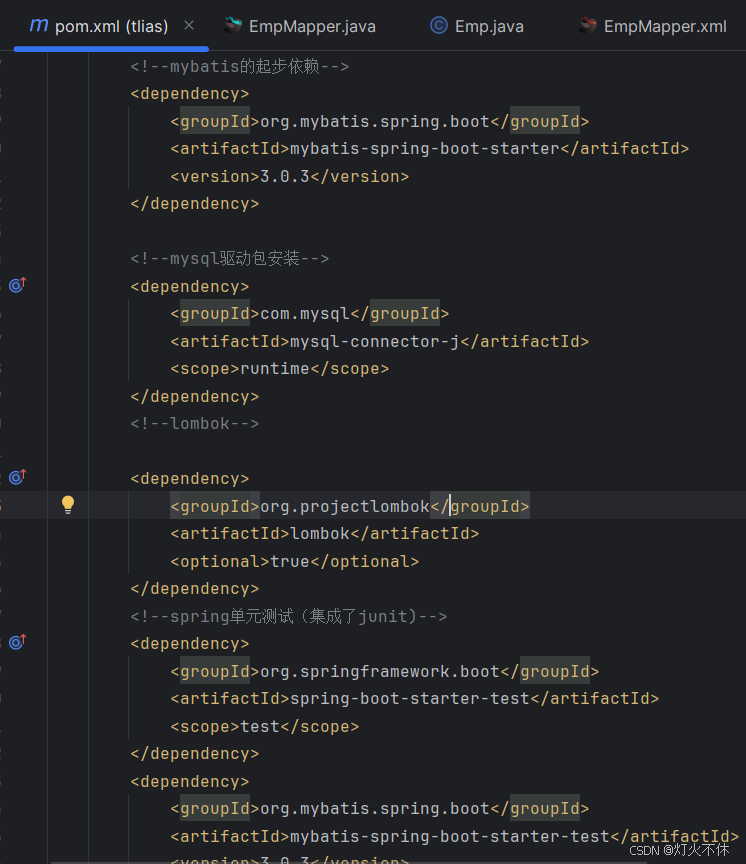
创建数据库表和实体类
在数据库中创建表user,并创建对应的实体类User
连接数据库
在文件application.properties中配置增加代码
全部都是 spring.datasource.xxxx 开头。
#驱动类名称
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
#数据库连接的url
spring.datasource.url=jdbc:mysql://localhost:3306/mybatis
#连接数据库的用户名
spring.datasource.username=root
#连接数据库的密码
spring.datasource.password=1234
记得修改url,使用自己数据库的用户名和密码
创建接口XxxMapper
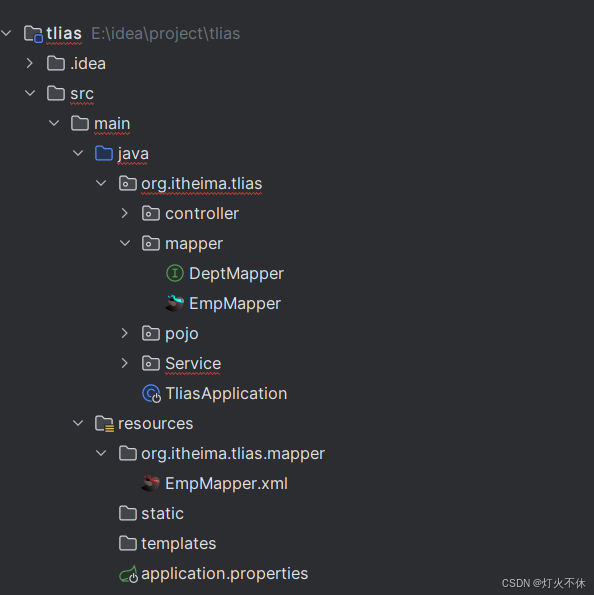
在接口文件中加入代码
@Mapper
public interface UserMapper {
//查询所有用户数据
@Select("select id, name, age, gender, phone from user")
public List<User> list();
}
@Mapper注解:表示是mybatis中的Mapper接口
程序运行时,框架会自动生成接口的实现类对象(代理对象),并给交Spring的IOC容器管理
@Select注解:代表的就是select查询,用于书写select查询语句
1. application.properties
#驱动类名称
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
#数据库连接的url
spring.datasource.url=jdbc:mysql://localhost:3306/mybatis
#连接数据库的用户名
spring.datasource.username=root
#连接数据库的密码
spring.datasource.password=1234
2. Mapper接口(编写SQL语句)
@Mapper
public interface UserMapper {
@Select("select id, name, age, gender, phone from user")
public List<User> list();
}
2.数据库连接池
数据库连接池是个容器,负责分配、管理数据库连接(Connection)
没有使用数据库连接池:
客户端执行SQL语句:要先创建一个新的连接对象,然后执行SQL语句,SQL语句执行后又需
要关闭连接对象从而释放资源,每次执行SQL时都需要创建连接、销毁链接,这种频繁的重复
创建销毁的过程是比较耗费计算机的性能。
使用数据库连接池
程序在启动时,会在数据库连接池(容器)中,创建一定数量的Connection对象
允许应用程序重复使用一个现有的数据库连接,而不是再重新建立一个
客户端在执行SQL时,先从连接池中获取一个Connection对象,然后在执行SQL语句,SQL语句执
行完之后,释放Connection时就会把Connection对象归还给连接池(Connection对象可以复用)
释放空闲时间超过最大空闲时间的连接,来避免因为没有释放连接而引起的数据库连接遗漏
客户端获取到Connection对象了,但是Connection对象并没有去访问数据库(处于空闲),数据库连
接池发现Connection对象的空闲时间 > 连接池中预设的最大空闲时间,此时数据库连接池就会自
动释放掉这个连接对象
数据库连接池的好处:
1. 资源重用
2. 提升系统响应速度
3. 避免数据库连接遗漏
常见的数据库连接池:
C3P0 、DBCP 、Druid 、Hikari (springboot默认)
现在使用更多的是:Hikari、Druid (性能更优越) 、Hikari(追光者) [默认的连接池]
如果想把默认的数据库连接池切换为Druid数据库连接池,只需要完成以下两步操作即可:
1. 在pom.xml文件中引入依赖
<dependency>
<!-- Druid连接池依赖 -->
<groupId>com.alibaba</groupId>
<artifactId>druid-spring-boot-starter</artifactId>
<version>1.2.8</version>
</dependency>
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/mybatis
spring.datasource.username=root
spring.datasource.password=1234
优点
1. 数据库连接四要素(驱动、链接、用户名、密码),都配置在springboot默认的配置文件
application.properties中
2. 查询结果的解析及封装,由mybatis自动完成映射封装,我们无需关注
3. 在mybatis中使用了数据库连接池技术,从而避免了频繁的创建连接、销毁连接而带来的资源浪
费。
使用SpringBoot+Mybatis的方式操作数据库,能够提升开发效率、降低资源浪费
1. application.properties
2. Mapper接口(编写SQL语句)
3. 数据库连接池
在前面我们所讲解的mybatis中,使用了数据库连接池技术,避免频繁的创建连接、销毁连接而带来的资源浪费。
Lombok
Lombok是一个实用的Java类库,可以通过简单的注解来简化和消除一些必须有但显得很臃肿的Java代码。
通过注解的形式自动生成构造器、getter/setter、equals、hashcode、toString等方法,并可以自 动化生成日志变量,简化java开发、提高效率。
使用
<!-- 在springboot的父工程中,已经集成了lombok并指定了版本号,故当前引入依赖时不需要指定 version -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
第2步:在实体类上添加注解
import lombok.Data;
@Data //getter方法、setter方法、toString方法、hashCode方法、equals方法
@NoArgsConstructor //无参构造
@AllArgsConstructor//全参构造
public class User {
private Integer id;
private String name;
private Short age;
private Short gender;
private String phone;
}
Mybatis
准备工作
1. 准备数据库表
2. 创建一个新的springboot工程,选择引入对应的起步依赖(mybatis、mysql驱动、lombok)
3. application.properties中引入数据库连接信息
4. 创建对应的实体类 Emp(实体类属性采用驼峰命名)
5. 准备Mapper接口 EmpMapper
准备好后,项目工程结构目录如下
删除
SQL语句
-- 删除 id=17 的数据delete from emp where id = 17 ;
接口方法实现删除操作
@Mapper
public interface EmpMapper {
@Delete("delete from emp where id = #{id}")//使用#{key}方式获取方法中的参数值
public void delete(Integer id);
}
日志输入
在Mybatis当中我们可以借助日志,查看到sql语句的执行、执行传递的参数以及执行结果。具体操作如下:
1. 打开application.properties文件
2. 开启mybatis的日志,并指定输出到控制台
#指定mybatis输出日志的位置, 输出控制台
mybatis.configuration.log-impl=org.apache.ibatis.logging.stdout.StdOutImpl
预编译SQL的两个优势:
性能更高:预编译SQL,编译一次之后会将编译后的SQL语句缓存起来,后面再次执行这条语句
时,不会再次编译。(只是输入的参数不同)
更安全(防止SQL注入):将敏感字进行转义,保障SQL的安全性。
参数占位符
在Mybatis中提供的参数占位符有两种:${...} 、#{...}
#{...}
执行SQL时,会将#{…}替换为?,生成预编译SQL,会自动设置参数值
使用时机:参数传递,都使用#{…}
${...}
拼接SQL。直接将参数拼接在SQL语句中,存在SQL注入问题
使用时机:如果对表名、列表进行动态设置时使用
注意事项:在项目开发中,建议使用#{...},生成预编译SQL,防止SQL注入安全
新增
SQL语句
insert into emp(username, name, gender, image, job, entrydate, dept_id,
create_time, update_time) values ('songyuanqiao','宋远桥',1,'1.jpg',2,'2012-10-
09',2,'2022-10-01 10:00:00','2022-10-01 10:00:00');
接口方法实现删除操作
@Mapper
public interface EmpMapper {
@Insert("insert into emp(username, name, gender, image, job, entrydate,
dept_id, create_time, update_time) values (#{username}, #{name}, #{gender}, #
{image}, #{job}, #{entrydate}, #{deptId}, #{createTime}, #{updateTime})")
public void insert(Emp emp);
}
更新
SQL语句
update emp set username = 'linghushaoxia' , name = ' 令狐少侠 ' , gender = 1 , image ='1.jpg' , job = 2 , entrydate = '2012-01-01' , dept_id = 2 , update_time = '2022-10-01 12:12:12' where id = 18 ;
接口方法实现更新操作
@Mapper
public interface EmpMapper {
/**
* 根据id修改员工信息
* @param emp
*/
@Update("update emp set username=#{username}, name=#{name}, gender=#{gender},
image=#{image}, job=#{job}, entrydate=#{entrydate}, dept_id=#{deptId},
update_time=#{updateTime} where id=#{id}")
public void update(Emp emp);
}
查询
SQL语句
select id, username, password , name, gender, image, job, entrydate, dept_id,create_time, update_time from emp;
接口方法事项查询操作
@Mapperpublic interface EmpMapper {@Select ( "select id, username, password, name, gender, image, job, entrydate,dept_id, create_time, update_time from emp where id=#{id}" )public Emp getById ( Integer id );}
驼峰命名
# 在 application.properties 中添加:mybatis.configuration.map-underscore-to-camel-case = true
条件查询
SQL语句
select id, username, password , name, gender, image, job, entrydate, dept_id,create_time, update_timefrom empwhere name like '% 张 %'and gender = 1and entrydate between '2010-01-01' and '2020-01-01 'order by update_time desc ;
接口方法实现条件查询
方法一
@Mapperpublic interface EmpMapper {
@Select("select * from emp " +
"where name like '%${name}%' " +
"and gender = #{gender} " +
"and entrydate between #{begin} and #{end} " +
"order by update_time desc")
public List<Emp> list(String name, Short gender, LocalDate begin, LocalDate
end);
}
1. 方法中的形参名和SQL语句中的参数占位符名保持一致
2. 模糊查询使用${...}进行字符串拼接,这种方式呢,由于是字符串拼接,并不是预编译的形
式,所以效率不高、且存在sql注入风险。
性能低、不安全、存在SQL注入风险
方法二
@Mapper
public interface EmpMapper {
@Select("select * from emp " +
"where name like concat('%',#{name},'%') " +
"and gender = #{gender} " +
"and entrydate between #{begin} and #{end} " +
"order by update_time desc")
public List<Emp> list(String name, Short gender, LocalDate begin, LocalDate
end);
}
性能高、安全
XML
Mybatis的开发有两种方式
1. 注解
2. XML
使用Mybatis的注解方式,主要是来完成一些简单的增删改查功能。如果需要实现复杂的SQL功能,建议
使用XML来配置映射语句,也就是将SQL语句写在XML配置文件中。
在Mybatis中使用XML映射文件方式开发,需要符合一定的规范:
1. XML映射文件的名称与Mapper接口名称一致,并且将XML映射文件和Mapper接口放置在相同包下(同包同名)
2. XML映射文件的namespace属性为Mapper接口全限定名一致
3. XML映射文件中sql语句的id与Mapper接口中的方法名一致,并保持返回类型一致。
<select>标签:就是用于编写select查询语句的。
resultType属性,指的是查询返回的单条记录所封装的类型。
创建XML文件
编写XML映射文件
在XML映射文件中写入
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="">
</mapper>
Mybatis动态SQL
<if> :用于判断条件是否成立。使用test属性进行条件判断,如果条件为true,则拼接SQL
<if test="条件表达式">
要拼接的sql语句
</if>
条件查询
原SQL语句
<select id="list" resultType="com.itheima.pojo.Emp">
select * from emp
where name like concat('%',#{name},'%')
and gender = #{gender}
and entrydate between #{begin} and #{end}
order by update_time desc
</select>
动态SQL语句
<select id="list" resultType="com.itheima.pojo.Emp">
select * from emp
where
<if test="name != null">
name like concat('%',#{name},'%')
</if>
<if test="gender != null">
and gender = #{gender}
</if>
<if test="begin != null and end != null">
and entrydate between #{begin} and #{end}
</if>
order by update_time desc
</select>
使用 <where> 标签代替SQL语句中的where关键字
<where> 只会在子元素有内容的情况下才插入where子句,而且会自动去除子句的开头的AND或
OR
动态SQL语句
<select id="list" resultType="com.itheima.pojo.Emp">
select * from emp
<where>
<!-- if做为where标签的子元素 -->
<if test="name != null">
and name like concat('%',#{name},'%')
</if>
<if test="gender != null">
and gender = #{gender}
</if>
<if test="begin != null and end != null">
and entrydate between #{begin} and #{end}
</if>
</where>
order by update_time desc
</select>
更新员工
修改Maper接口
@Mapper
public interface EmpMapper {
//删除@Update注解编写的SQL语句
//update操作的SQL语句编写在Mapper映射文件中
public void update(Emp emp);
}
修改XML映射文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.itheima.mapper.EmpMapper">
<!--更新操作-->
<update id="update">
update emp
set
<if test="username != null">
username=#{username},
</if>
<if test="name != null">
name=#{name},
</if>
<if test="gender != null">
gender=#{gender},
</if>
<if test="image != null">
image=#{image},
</if>
<if test="job != null">
job=#{job},
</if>
<if test="entrydate != null">
entrydate=#{entrydate},
</if>
<if test="deptId != null">
dept_id=#{deptId},
</if>
<if test="updateTime != null">
update_time=#{updateTime}
</if>
where id=#{id}
</update>
</mapper>
使用 <set> 标签代替SQL语句中的set关键字
<set> :动态的在SQL语句中插入set关键字,并会删掉额外的逗号。(用于update语句中)
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.itheima.mapper.EmpMapper">
<!--更新操作-->
<update id="update">
update emp
<!-- 使用set标签,代替update语句中的set关键字 -->
<set>
<if test="username != null">
username=#{username},
</if>
<if test="name != null">
name=#{name},
</if>
<if test="gender != null">
gender=#{gender},
</if>
<if test="image != null">
image=#{image},
</if>
<if test="job != null">
job=#{job},
</if>
<if test="entrydate != null">
entrydate=#{entrydate},
</if>
<if test="deptId != null">
dept_id=#{deptId},
</if>
<if test="updateTime != null">
update_time=#{updateTime}
</if>
</set>
where id=#{id}
</update>
</mapper>
文中部分内容及图片来自黑马程序员