一、思维导图
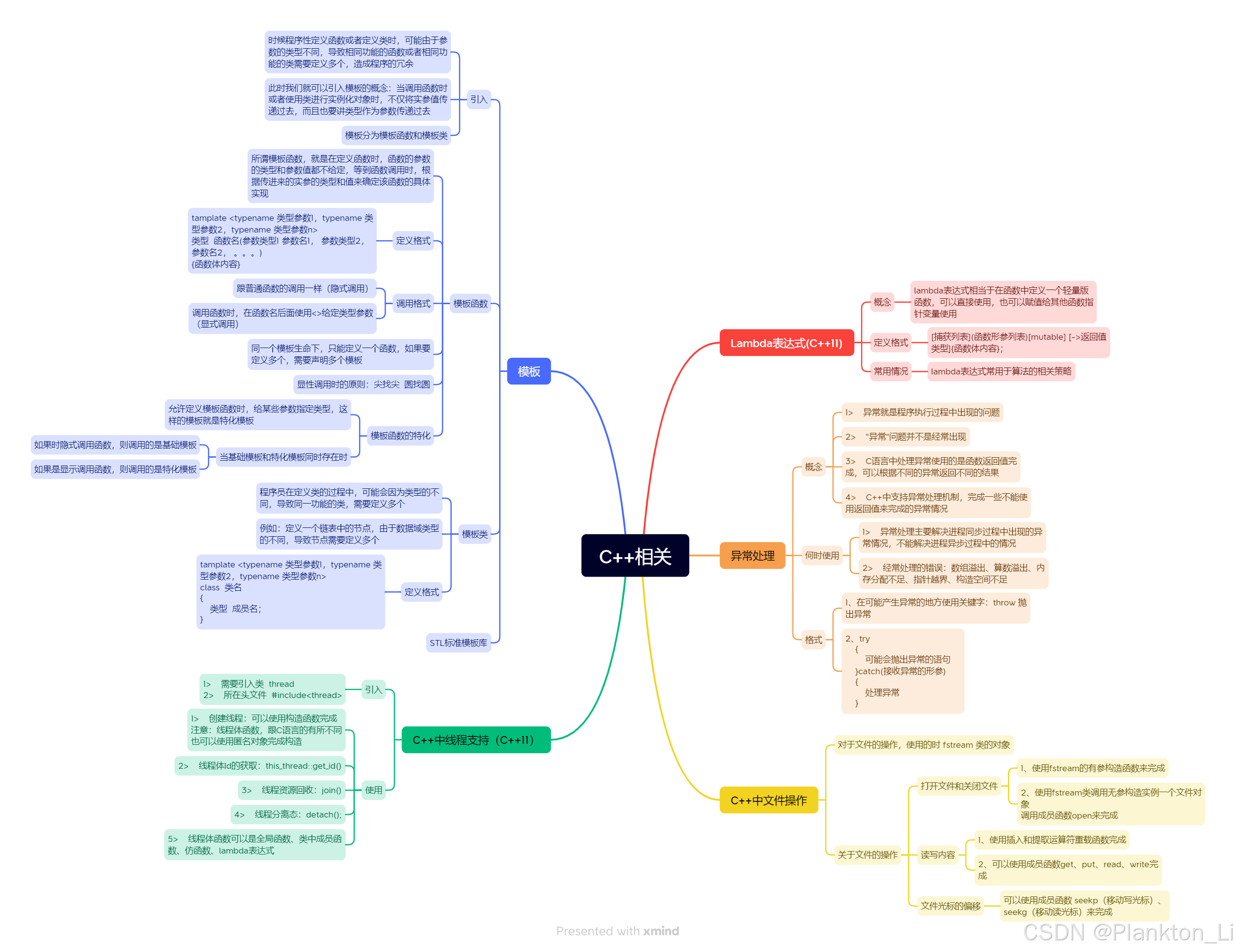
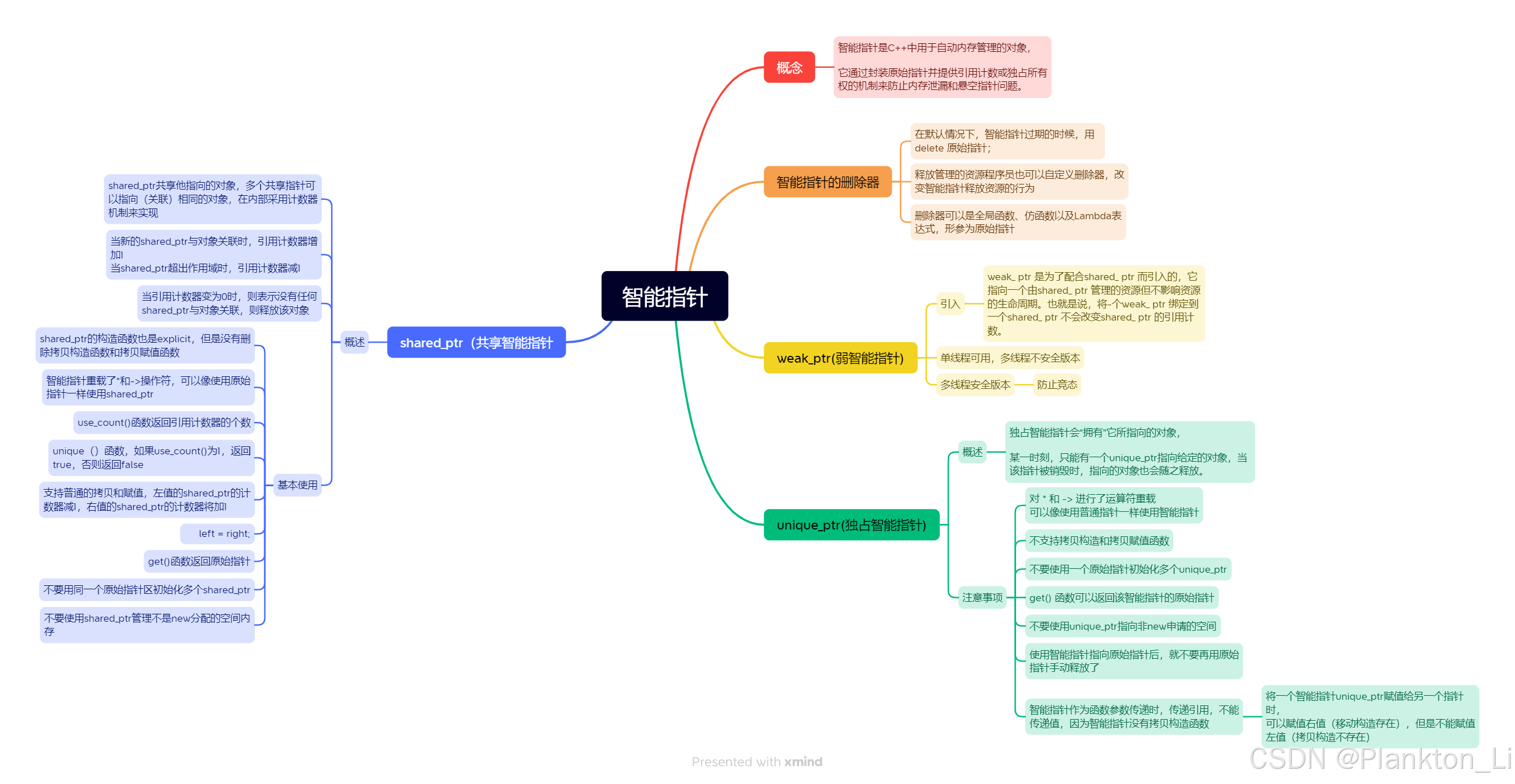
二、模板类实现myStack和myQueue
#include <iostream>
using namespace std;
template <typename T>
class MyStack {
private:
T* arr;
int capacity;
int topIndex;
public:
MyStack(int size);
~MyStack();
void push(const T& value);
void pop();
T top() const;
bool empty() const;
size_t size() const;
};
template <typename T>
MyStack<T>::MyStack(int size) {
capacity = size;
arr = new T[capacity];
topIndex = -1;
}
template <typename T>
MyStack<T>::~MyStack() {
delete[] arr;
}
template <typename T>
void MyStack<T>::push(const T& value) {
if (topIndex == capacity - 1) {
cout << "栈已满,无法添加元素" << endl;
return;
}
arr[++topIndex] = value;
}
template <typename T>
void MyStack<T>::pop() {
if (topIndex == -1) {
cout << "栈为空,无法弹出元素" << endl;
return;
}
topIndex--;
}
template <typename T>
T MyStack<T>::top() const {
if (topIndex == -1) {
cout << "栈为空,无法获取顶部元素" << endl;
return T(); // 返回默认构造的元素
}
return arr[topIndex];
}
template <typename T>
bool MyStack<T>::empty() const {
return topIndex == -1;
}
template <typename T>
size_t MyStack<T>::size() const {
return topIndex + 1;
}
int main() {
MyStack<int> myStack1(5);
myStack1.push(1);
myStack1.push(2);
myStack1.push(3);
cout << "栈顶元素: " << myStack1.top() << endl;
myStack1.pop();
cout << "栈顶元素: " << myStack1.top() << endl;
MyStack<string> myStack2(5);
myStack2.push("A");
myStack2.push("B");
myStack2.push("C");
cout << "栈顶元素: " << myStack2.top() <<endl;
myStack2.pop();
cout << "栈顶元素: " << myStack2.top() << endl;
return 0;
}
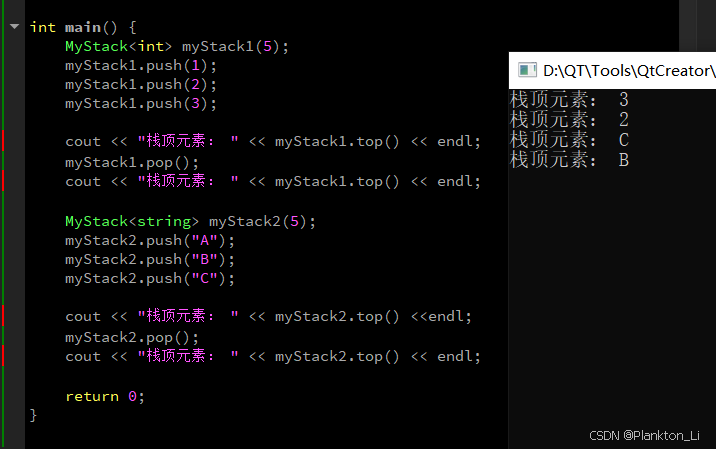
#include <iostream>
using namespace std;
#ifndef MYQUEUE_H
#define MYQUEUE_H
template <typename T>
class myQueue
{
private:
T *data;
size_t frontIndex;
size_t backIndex;
size_t capacity;
public:
myQueue();
myQueue(size_t cap);
myQueue(const myQueue& other);
virtual ~myQueue();
myQueue& operator=(const myQueue other);
void expend();
T& front();
T& back();
bool empty();
size_t get_size();
myQueue& push(T element);
myQueue& pop();
};
#endif // MYQUEUE_H
template <typename T>
myQueue<T>::myQueue():frontIndex(0),backIndex(0),capacity(5) {
data = new T [5];
}
template <typename T>
myQueue<T>::myQueue(size_t cap):frontIndex(0),backIndex(0) {
data = new T[cap];
capacity = cap;
}
template <typename T>
myQueue<T>::myQueue(const myQueue& other) {
if(this != &other) {
capacity = other.capacity;
data = new T[capacity];
frontIndex = other.frontIndex;
backIndex = other.backIndex;
copy(other.data, other.data + capacity, data);
}
}
template <typename T>
myQueue<T>::~myQueue() {
delete []data;
}
template <typename T>
myQueue<T>& myQueue<T>::operator=(const myQueue other) {
if (this == &other) {
return *this;
}
delete[] data;
capacity = other.capacity;
frontIndex = other.frontIndex;
backIndex = other.backIndex;
data = new T[capacity];
copy(other.data, other.data + capacity, data);
return *this;
}
template <typename T>
void myQueue<T>::expend() {
size_t newCapacity = capacity * 2;
T* newData = new T[newCapacity];
copy(data, data + capacity, newData);
delete[] data;
data = newData;
capacity = newCapacity;
}
template <typename T>
T& myQueue<T>::front() {
return data[frontIndex];
}
template <typename T>
T& myQueue<T>::back() {
return data[backIndex-1];
}
template <typename T>
bool myQueue<T>::empty() {
return (frontIndex == backIndex);
}
template <typename T>
size_t myQueue<T>::get_size() {
return (backIndex - frontIndex);
}
template <typename T>
myQueue<T>& myQueue<T>::push(T element) {
if(capacity == (backIndex+1)) {
this->expend();
}
this->data[backIndex] = element;
this->backIndex++;
return *this;
}
template <typename T>
myQueue<T>& myQueue<T>::pop() {
if (empty()) {
throw runtime_error("Queue is empty");
}
frontIndex++;
return *this;
}
int main() {
myQueue<int> myQueue1(5);
myQueue1.push(1);
myQueue1.push(2);
myQueue1.push(3);
cout << "队列首元素: " << myQueue1.front() << endl;
myQueue1.pop();
cout << "队列首元素: " << myQueue1.front() << endl;
myQueue<string> myQueue2(5);
myQueue2.push("A");
myQueue2.push("B");
myQueue2.push("C");
cout << "队列首元素: " << myQueue2.front() <<endl;
myQueue2.pop();
cout << "队列首元素: " << myQueue2.front() << endl;
return 0;
}
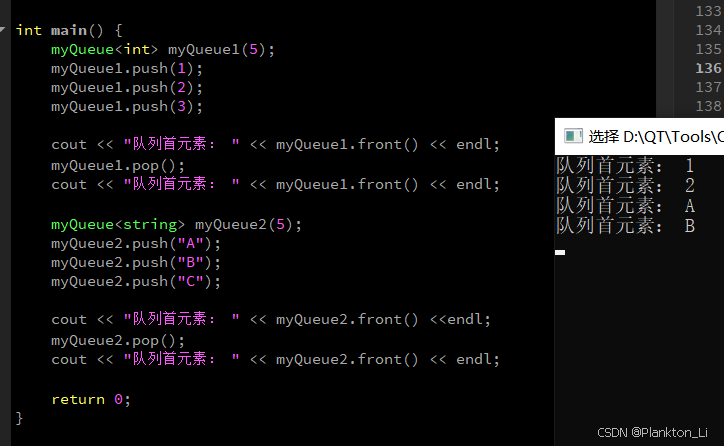