自定义创建项目
ESlint 代码规范
代码规范错误 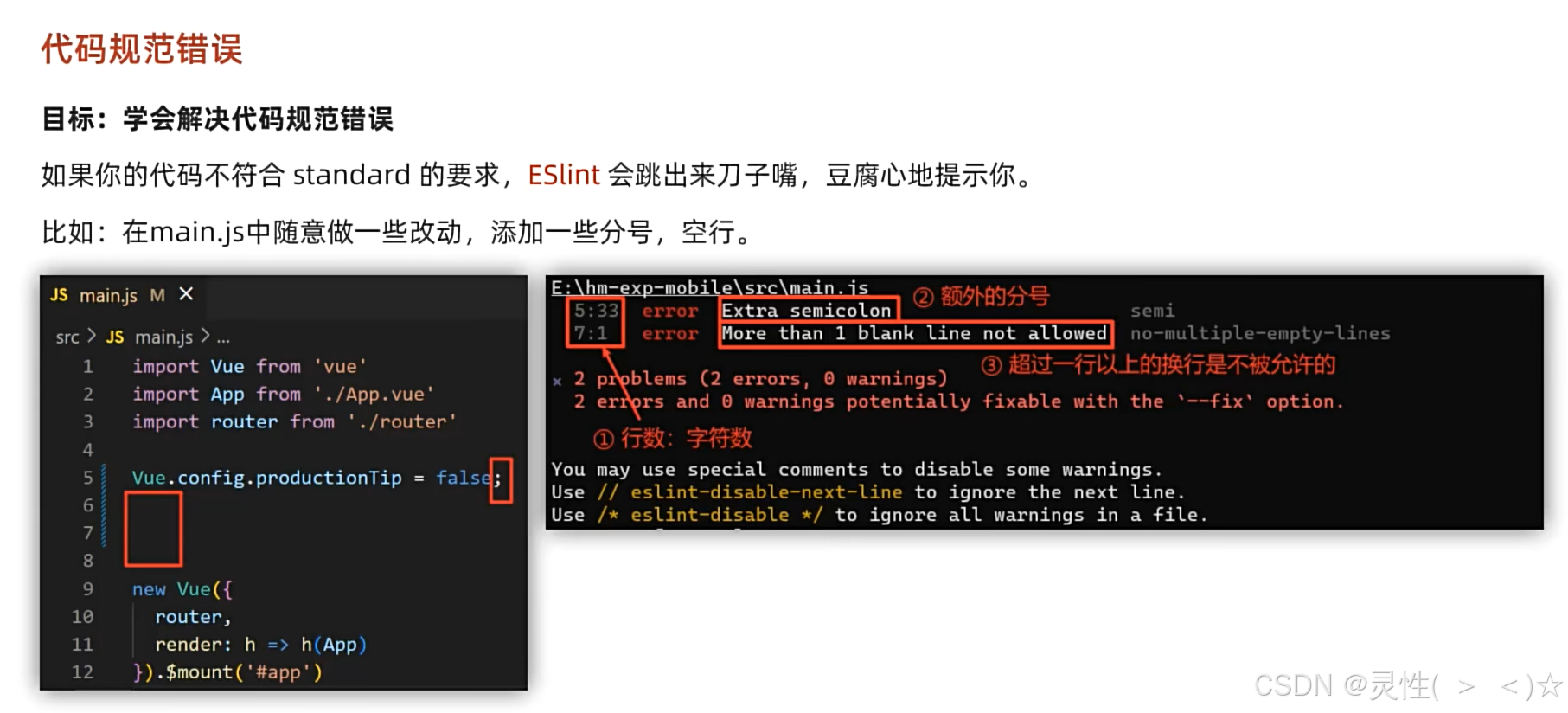
手动修正
自动修正
settings.json
{
"emmet.triggerExpansionOnTab": true,
"editor.fontSize": 25,
// 当保存的时候,eslint自动帮我们修复错误
"editor.codeActionsOnSave": {
"source.fixAll": true
},
// 保存代码,不自动格式化
"editor.formatOnSave": false
}
vuex概述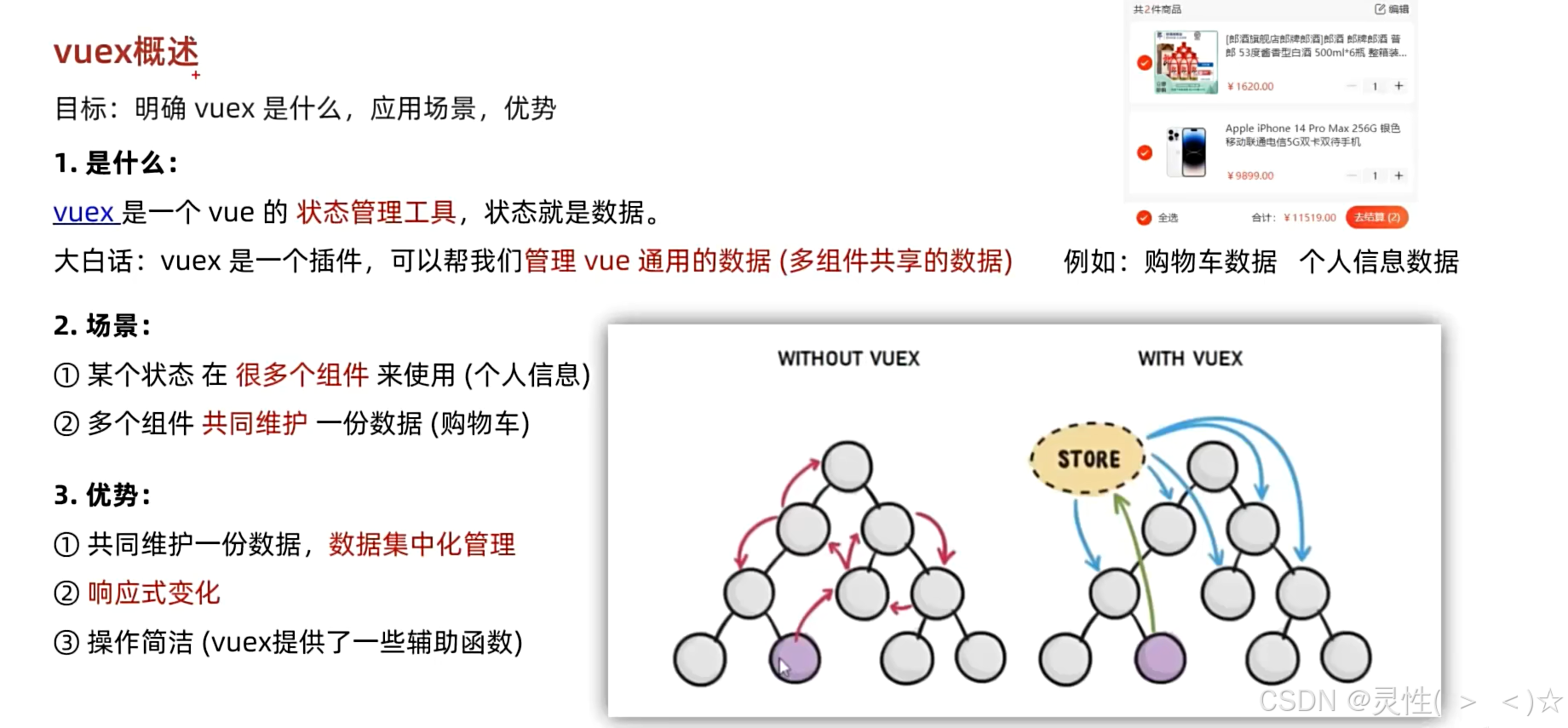
构建vuex[多组件数据共享]环境
创建一个空仓库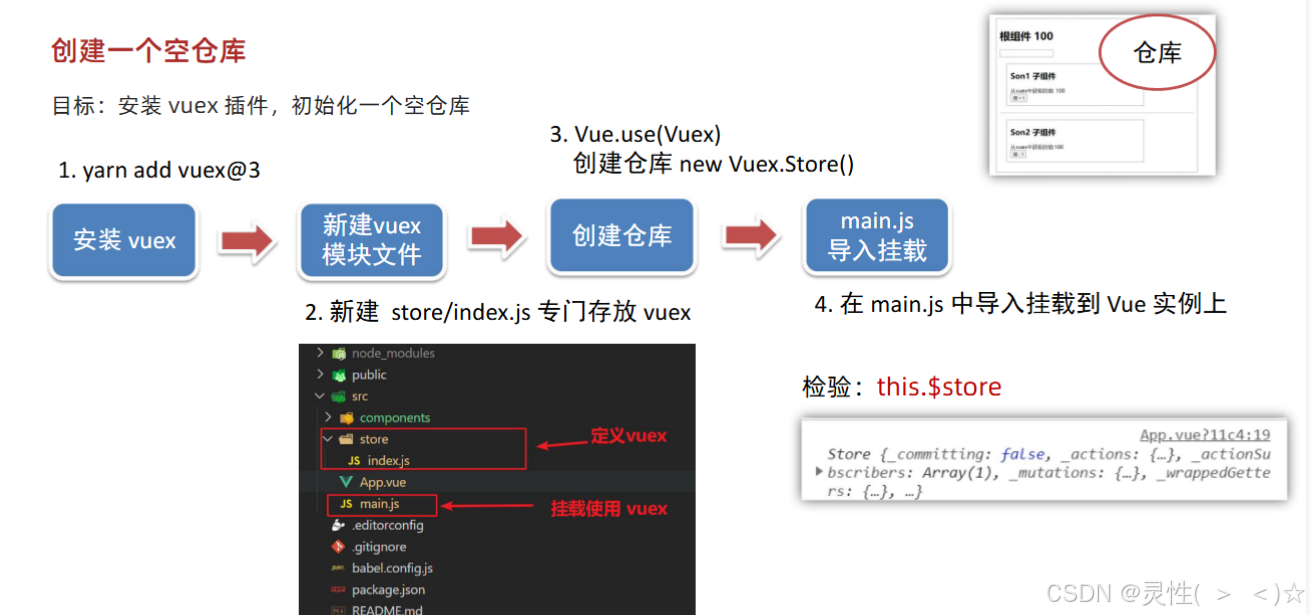
index.js
// 这里面存放的就是 vuex 相关的核心代码
import Vue from 'vue'
import Vuex from 'vuex'
import user from './modules/user'
import setting from './modules/setting'
// 插件安装
Vue.use(Vuex)
// 创建仓库(空仓库)
const store = new Vuex.Store()
// 导出给main.js使用
export default store
main.js
import Vue from 'vue'
import App from './App.vue'
import store from '@/store/index' // 导入
console.log(store.state.count)
Vue.config.productionTip = false
new Vue({
render: h => h(App),
store // 挂载
}).$mount('#app')
核心概念:state 状态
提供数据
index.js
// 这里面存放的就是 vuex 相关的核心代码
import Vue from 'vue'
import Vuex from 'vuex'
import user from './modules/user'
import setting from './modules/setting'
// 插件安装
Vue.use(Vuex)
// 创建仓库(空仓库)
const store = new Vuex.Store({
// 1. 通过 state 可以提供数据 (所有组件共享的数据)
state: {
title: '仓库大标题',
count: 100,
list: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
}
})
// 导出给main.js使用
export default store
使用数据
通过 store 直接访问
通过辅助函数 (简化)
App.vue
<template>
<div id="app">
<h1>
根组件
- {{ title }}
- {{ count }}
</h1>
<input :value="count" @input="handleInput" type="text">
<Son1></Son1>
<hr>
<Son2></Son2>
</div>
</template>
<script>
import Son1 from './components/son1'
import Son2 from './components/son2'
// 1.导入 mapState
import { mapState } from 'vuex'
// console.log(mapState(['count', 'title']))
export default {
name: 'app',
created () {
// console.log(this.$router) // 没配
console.log(this.$store.state.count)
},
computed: { // 3.展开运算符映射
...mapState(['count', 'title']) // 2.数组方式引入state
},
</script>
<style>
#app {
width: 600px;
margin: 20px auto;
border: 3px solid #ccc;
border-radius: 3px;
padding: 10px;
}
</style>
核心概念:mutations
mutations操作流程
index.js
son1.vue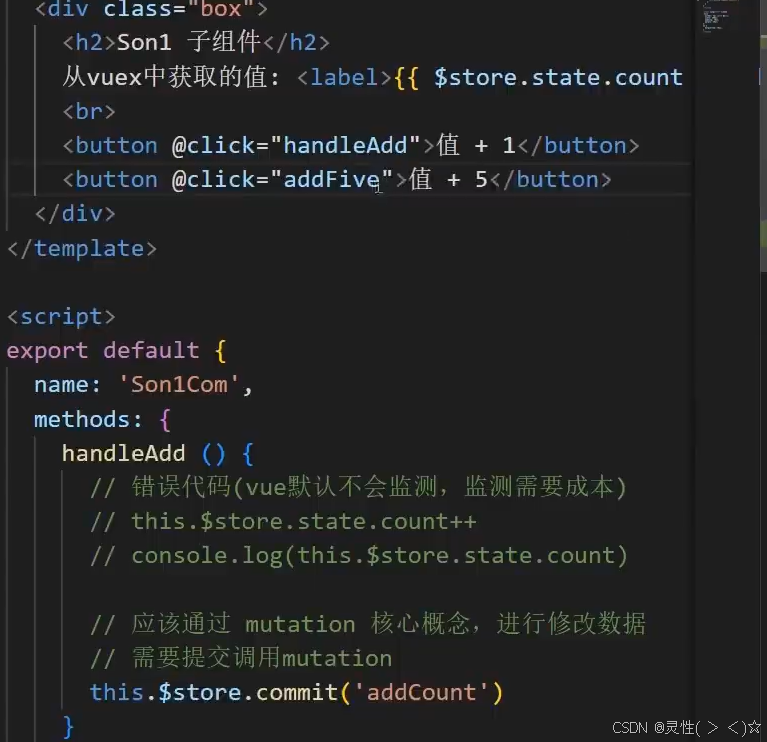
mutations传参语法
index.js
// 这里面存放的就是 vuex 相关的核心代码
import Vue from 'vue'
import Vuex from 'vuex'
import user from './modules/user'
import setting from './modules/setting'
// 插件安装
Vue.use(Vuex)
// 创建仓库(空仓库)
const store = new Vuex.Store({
// 严格模式 (有利于初学者,检测不规范的代码 => 上线时需要关闭)
strict: true,
// 1. 通过 state 可以提供数据 (所有组件共享的数据)
state: {
title: '仓库大标题',
count: 100,
list: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
},
// 2. 通过 mutations 可以提供修改数据的方法
mutations: {
// 所有mutation函数,第一个参数,都是 state
// 注意点:mutation参数有且只能有一个,如果需要多个参数,包装成一个对象
addCount (state, obj) {
console.log(obj)
// 修改数据
state.count += obj.count
},
subCount (state, n) {
state.count -= n
},
changeCount (state, newCount) {
state.count = newCount
},
changeTitle (state, newTitle) {
state.title = newTitle
}
}
})
// 导出给main.js使用
export default store
son1.vue
<template>
<div class="box">
<h2>Son1 子组件</h2>
从vuex中获取的值: <label>{{ $store.state.count }}</label>
<br>
<button @click="handleAdd(1)">值 + 1</button>
<button @click="handleAdd(5)">值 + 5</button>
<button @click="handleAdd(10)">值 + 10</button>
<button @click="handleChange">一秒后修改成666</button>
<button @click="changeFn">改标题</button>
<hr>
<!-- 计算属性getters -->
<div>{{ $store.state.list }}</div>
<div>{{ $store.getters.filterList }}</div>
<hr>
<!-- 测试访问模块中的state - 原生 -->
<div>{{ $store.state.user.userInfo.name }}</div>
<button @click="updateUser">更新个人信息</button>
<button @click="updateUser2">一秒后更新信息</button>
<div>{{ $store.state.setting.theme }}</div>
<button @click="updateTheme">更新主题色</button>
<hr>
<!-- 测试访问模块中的getters - 原生 -->
<div>{{ $store.getters['user/UpperCaseName'] }}</div>
</div>
</template>
<script>
export default {
name: 'Son1Com',
created () {
console.log(this.$store.getters)
},
methods: {
updateUser () {
// $store.commit('模块名/mutation名', 额外传参)
this.$store.commit('user/setUser', {
name: 'xiaowang',
age: 25
})
},
updateUser2 () {
// 调用action dispatch
this.$store.dispatch('user/setUserSecond', {
name: 'xiaohong',
age: 28
})
},
updateTheme () {
this.$store.commit('setting/setTheme', 'pink')
},
handleAdd (n) {
// 错误代码(vue默认不会监测,监测需要成本)
// this.$store.state.count++ // 单向数据流不可直接修改,需要申请
// console.log(this.$store.state.count)
// 应该通过 mutation 核心概念,进行修改数据
// 需要提交调用mutation
// this.$store.commit('addCount')
// console.log(n)
// 调用带参数的mutation函数
this.$store.commit('addCount', {
count: n,
msg: '哈哈'
})
},
changeFn () {
this.$store.commit('changeTitle', '传智教育')
},
handleChange () {
// 调用action
// this.$store.dispatch('action名字', 额外参数)
this.$store.dispatch('changeCountAction', 666)
}
}
}
</script>
<style lang="css" scoped>
.box{
border: 3px solid #ccc;
width: 400px;
padding: 10px;
margin: 20px;
}
h2 {
margin-top: 10px;
}
</style>
mutations 练习 (减法功能)
index.js
mutations 练习(实时)
输入框渲染
监听输入,获取内容
封装mutation处理函数
调用传参
辅助函数:mapMutations
核心概念:actions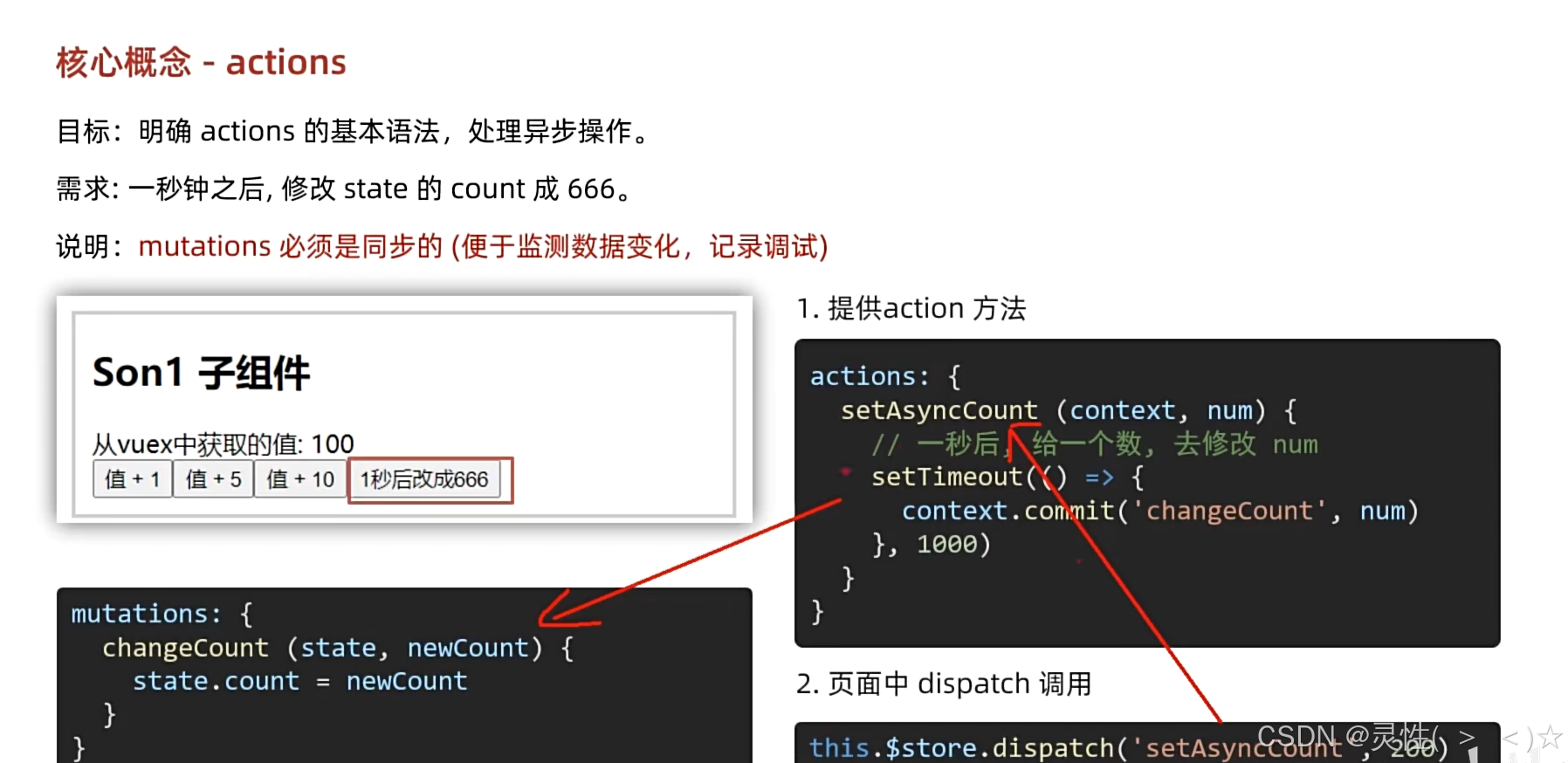
辅助函数:mapActions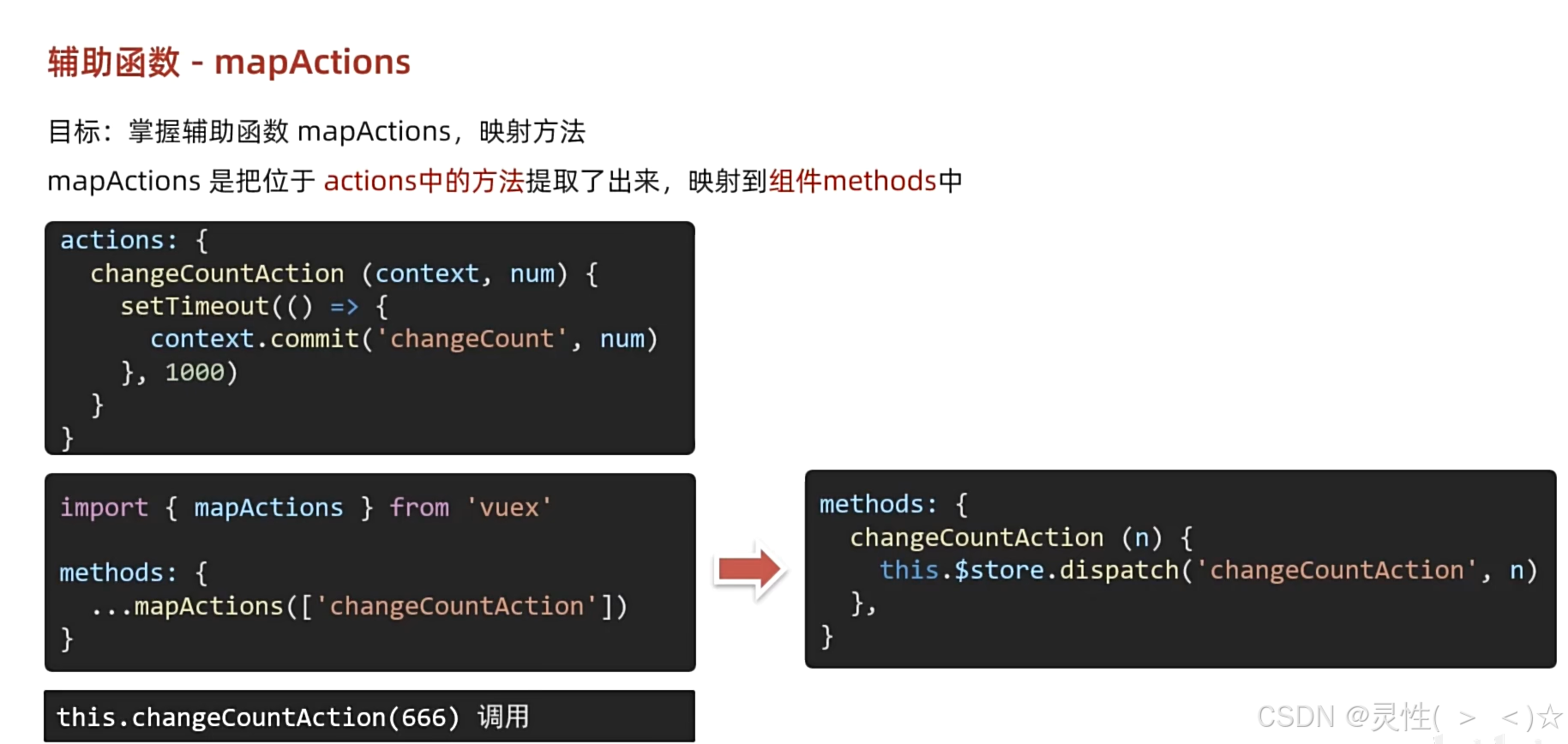
核心概念:getters
核心概念:模块module(进阶语法)
模块拆分
settings.js(store/modules/)
index.js(store)