一、TCP通讯(服务端)
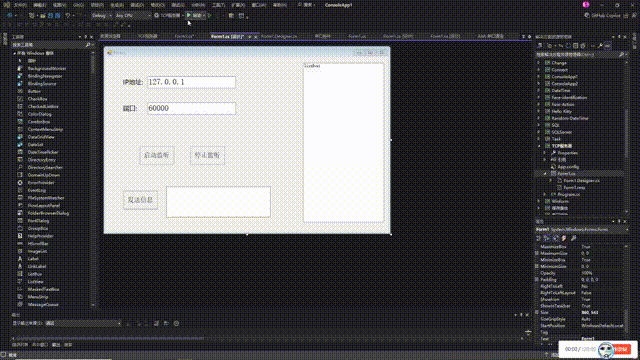
二、控件展示
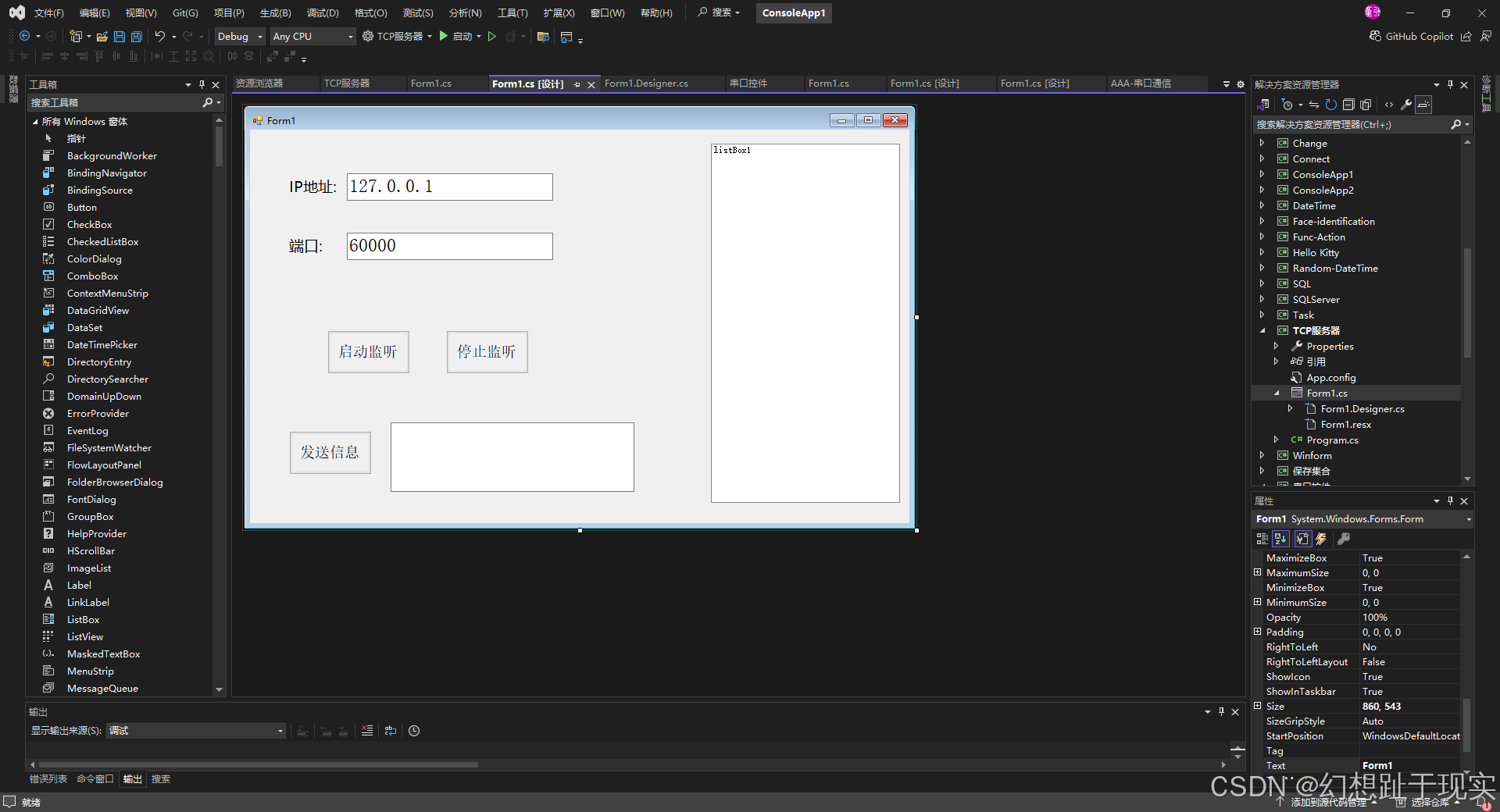
三、TCP 服务端 客户端 分辨
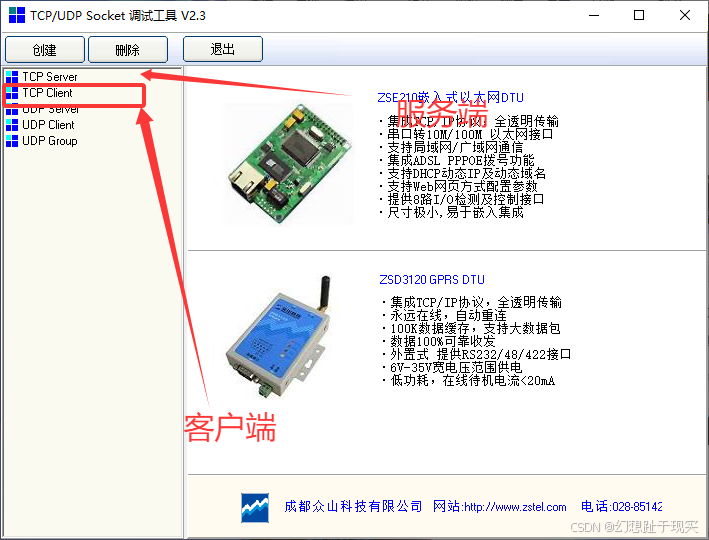
四、控件解析
一般IP地址本机都是 : 127.0.0.1
端口号会有显示: 60000
服务端: 启动监听 停止监听 都会有发送信息
服务端对接的是客户端 可以理解为是理解
控件:ListBox 是为了更好展示运行的过程
五、链接控件实施
1.实例化三个Socket
public Socket ServerSocket { get; set; }
public Socket SocketAccept { get; set; }
public Socket socket;
ServerSocket 是用来套接字 用来监听的套子节
SocketAccept 是用来接收绑定客户端的套接字
2.过程运行结果,展示
private void Showmsg(string s)
{
listBox1.BeginInvoke(new Action<string>(str =>
{
listBox1.Items.Add(str);
}), s);
}
注意: 由于在线程中,分线程不能操作ui界面,所以需要用Invoke来调用
或者: CheckForIllegalCrossThreadCalls=false; 对非法线程调用不进行检测
3.线程,开始监听事件
Thread t1;
Thread t2;
4.按钮一:启动监听实施
private void btnstart_Click(object sender, EventArgs e)
{
btnstart.Enabled=false;
btncancel.Enabled=true;
ServerSocket=new Socket(AddressFamily.InterNetwork,SocketType.Stream, ProtocolType.Tcp);
//绑定ip地址
IPAddress iPAddress=IPAddress.Parse(textBox1.Text);
//绑定端口号
int port=int.Parse(textBox2.Text);
//建立连接
IPEndPoint iPEndPoint=new IPEndPoint(iPAddress, port);
try
{
//绑定连接点
ServerSocket.Bind(iPEndPoint);
//开始监听
ServerSocket.Listen(5);
t1 = new Thread(ListenT1);
t1.IsBackground=true;
t1.Start(ServerSocket);
}
catch (Exception ex)
{
throw;
}
}
private void ListenT1(object o)
{
SocketAccept = o as Socket;
try
{
while(true)
{
//线程阻塞,直到有客户连接,再去执行后续代码
socket = SocketAccept.Accept();
Showmasg($"{DateTime.Now.ToString("HH-mm-ss")}{socket.RemoteEndPoint}连接成功");
t2 = new Thread(Received);
t2.IsBackground=true;
t2.Start(socket);
}
}catch (Exception ex)
{
throw;
}
}
private void Received(object o)
{
socket=o as Socket;
//传递的数据大小为2M
byte[]buffer=new byte[1024*1024*2];
int r=socket.Receive(buffer);
if (r==0)
{
return;
}
string s=Encoding.UTF8.GetString(buffer);
Showmasg(s);
}
代码解释:
实例化一个Socket
参数1:设置协议的类型 参数2:套接字的双向数据流 参数3:使用tcp通信协议
5.停止监听按钮
private void btncancel_Click(object sender, EventArgs e)
{
btncancel.Enabled = false;
if (socket != null)
{
socket.Shutdown(SocketShutdown.Both);
socket.Close();
}
ServerSocket.Close();
t1.Abort();
btnstart.Enabled = true;
}
6.发送信息效果展示
private void btnsend_Click(object sender, EventArgs e)
{
string s=textBox3.Text;
byte[]buffer=Encoding.UTF8.GetBytes(s);
try
{
int r = socket.Send(buffer);
Showmasg("发送成功");
textBox3.Clear();
}
catch (Exception ex)
{
throw;
}
}
六、清目了然的CV吧
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace TCP服务器
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
//创建Socket
public Socket ServerSocket { get; set; }
public Socket SocketAccept { get; set; }
public Socket socket;
private void Showmasg(string s)
{
listBox1.BeginInvoke(new Action<string>(str =>
{
listBox1.Items.Add(str);
}), s);
}
Thread t1;
Thread t2;
private void btnstart_Click(object sender, EventArgs e)
{
btnstart.Enabled=false;
btncancel.Enabled=true;
ServerSocket=new Socket(AddressFamily.InterNetwork,SocketType.Stream, ProtocolType.Tcp);
IPAddress iPAddress=IPAddress.Parse(textBox1.Text);
int port=int.Parse(textBox2.Text);
IPEndPoint iPEndPoint=new IPEndPoint(iPAddress, port);
try
{
ServerSocket.Bind(iPEndPoint);
ServerSocket.Listen(5);
t1 = new Thread(ListenT1);
t1.IsBackground=true;
t1.Start(ServerSocket);
}
catch (Exception ex)
{
throw;
}
}
private void ListenT1(object o)
{
SocketAccept = o as Socket;
try
{
while(true)
{
socket = SocketAccept.Accept();
Showmasg($"{DateTime.Now.ToString("HH-mm-ss")}{socket.RemoteEndPoint}连接成功");
t2 = new Thread(Received);
t2.IsBackground=true;
t2.Start(socket);
}
}catch (Exception ex)
{
throw;
}
}
private void Received(object o)
{
socket=o as Socket;
byte[]buffer=new byte[1024*1024*2];
int r=socket.Receive(buffer);
if (r==0)
{
return;
}
string s=Encoding.UTF8.GetString(buffer);
Showmasg(s);
}
private void btncancel_Click(object sender, EventArgs e)
{
btncancel.Enabled = false;
if (socket != null)
{
socket.Shutdown(SocketShutdown.Both);
socket.Close();
}
ServerSocket.Close();
t1.Abort();
btnstart.Enabled = true;
}
private void btnsend_Click(object sender, EventArgs e)
{
string s=textBox3.Text;
byte[]buffer=Encoding.UTF8.GetBytes(s);
try
{
int r = socket.Send(buffer);
Showmasg("发送成功");
textBox3.Clear();
}
catch (Exception ex)
{
throw;
}
}
private void Form1_Load(object sender, EventArgs e)
{
System.Diagnostics.Process.Start(@"D:\17_SocketTool\SocketTool.exe");
}
}
}