1.开通POP和IMAP
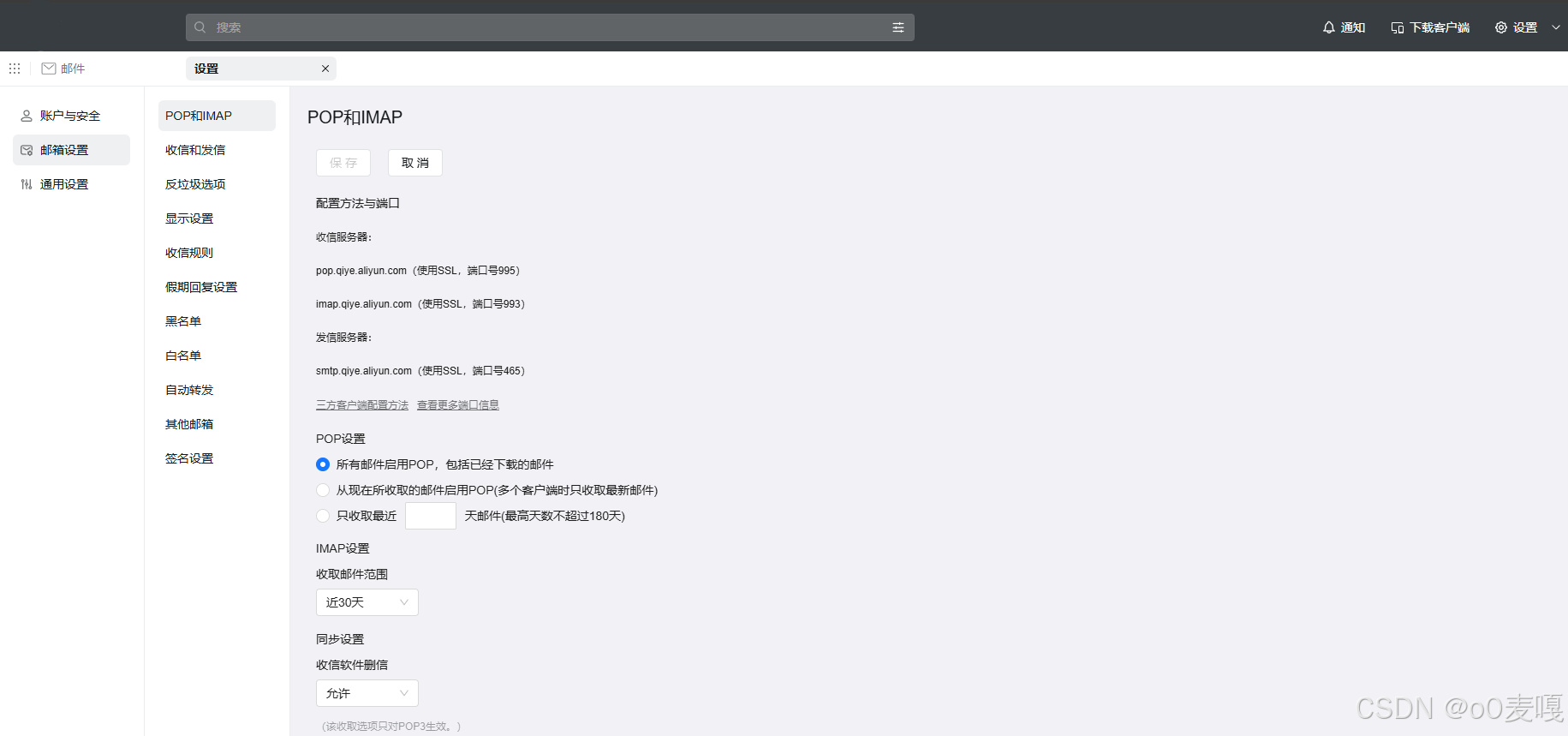
2.引入pom
<dependency>
<groupId>javax.mail</groupId>
<artifactId>mail</artifactId>
<version>1.4.7</version>
</dependency>
3.逻辑
String host = "smtp.qiye.aliyun.com";
String port = "465";
String username = "xxxxxx@dingtalk.com";
String password = "xxxxxx";
String toEmail = "xxxxx@dingtalk.com";
String subject = "邮件标题"
try {
Properties props = new Properties();
props.setProperty("mail.smtp.host", host);
props.setProperty("mail.smtp.port", port);
props.setProperty("mail.smtp.socketFactory.class","javax.net.ssl.SSLSocketFactory");
props.setProperty("mail.smtp.socketFactory.fallback", "false");
props.setProperty("mail.smtp.socketFactory.port", port);
props.setProperty("mail.smtp.auth", "true");
Session session = Session.getDefaultInstance(props, new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
MimeMessage message = new MimeMessage(session);
message.setFrom(new InternetAddress(username));
message.setRecipients(Message.RecipientType.TO, toEmail);
message.setSubject(subject);
MimeMultipart multipart = new MimeMultipart();
BodyPart contentPart = new MimeBodyPart();
contentPart.setContent(content, "text/html;charset=utf-8");
multipart.addBodyPart(contentPart);
MimeBodyPart attachmentBodyPart = new MimeBodyPart();
DataHandler dataHandler = new DataHandler(new FileDataSource("E:\\soft\\test.doc");
attachmentBodyPart.setDataHandler(dataHandler);
attachmentBodyPart.setFileName("test.doc");
multipart.addBodyPart(attachmentBodyPart);
message.setContent(multipart);
Transport.send(message);
} catch (Exception e) {
e.printStackTrace();
}
4.直接添加前端传过来的MultipartFile
.....
MimeMultipart multipart = new MimeMultipart();
BodyPart contentPart = new MimeBodyPart();
contentPart.setContent(content, "text/html;charset=utf-8");
multipart.addBodyPart(contentPart);
MimeBodyPart attachmentBodyPart = new MimeBodyPart();
File file = MultipartFileToFile(multipartFile);
DataSource source = new FileDataSource(file);
attachmentBodyPart.setDataHandler(new DataHandler(source));
attachmentBodyPart.setFileName(file.getName());
multipart.addBodyPart(attachmentBodyPart);
message.setContent(multipart);
Transport.send(message);
.....
5.添加多个附件
MimeMultipart multipart = new MimeMultipart();
BodyPart contentPart = new MimeBodyPart();
contentPart.setContent(content, "text/html;charset=utf-8");
multipart.addBodyPart(contentPart);
for (MultipartFile multipartFile : files){
MimeBodyPart attachmentBodyPart = new MimeBodyPart();
File file = MultipartFileToFile(multipartFile);
DataSource source = new FileDataSource(file);
attachmentBodyPart.setDataHandler(new DataHandler(source));
attachmentBodyPart.setFileName(file.getName());
multipart.addBodyPart(filePart);
}
message.setContent(multipart);
Transport.send(message);
6.给多个邮箱发邮件
String tos = "a1@dingtalk.com,a2@dingtalk.com,a3@dingtalk.com";
String[] toList = to.split(",");
Address[] addresses = new Address[toList.length];
for (int i = 0; i < toList.length; i++) {
addresses[i] = new InternetAddress(toList[i]);
}
message.setRecipients(Message.RecipientType.TO, addresses);