服务端
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading.Tasks;
namespace TeachTcpServer
{
class Program
{
static void Main(string[] args)
{
#region 知识点一 回顾服务端需要做的事情
//1.创建套接字Socket
//2.用Bind方法将套接字和本地地址绑定
//3.用Listen方法监听
//4.用Accept方法等待客户端连接
//5.建立连接、Accept返回新套接字
//6.用Send、Receive相关方法收发数据
//7.用Shutdown方法释放连接
//8.关闭套接字
#endregion
#region 知识点二 实现服务端基本逻辑
//1.创建套接字Socket(Tcp)
Socket socketTcp = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
//2.用Bind方法将套接字和本地地址绑定
try
{
IPEndPoint ipPoint = new IPEndPoint(IPAddress.Parse("127.0.0.1"), 8080);
socketTcp.Bind(ipPoint);
}
catch (Exception e)
{
Console.WriteLine("绑定报错" + e.Message);
return;
}
//3.用Listen方法监听
socketTcp.Listen(1024);
Console.WriteLine("服务端绑定监听结束,等待客户端连入");
//4.用Accept等待客户端连接
//5.建立连接,Accept返回 新的套接字
Socket socketClient= socketTcp.Accept();
//6.用Send和Receive收发数据
//发送
socketClient.Send(Encoding.UTF8.GetBytes("欢迎连入服务端"));
//接收
byte[] result = new byte[1024];
//返回值为接收到的字节数
int receiveNum= socketClient.Receive(result);
Console.WriteLine("接收到了{0}发来的消息{1}",
socketClient.RemoteEndPoint.ToString(),
Encoding.UTF8.GetString(result, 0, receiveNum));
//7.用Shutdown方法释放连接
socketClient.Shutdown(SocketShutdown.Both);
//8.关闭套接字
socketClient.Close();
#endregion
#region 总结
//1.服务端开启的流程每次都是相同的
//2.服务端的Accept、Send、Receive会阻塞主线程,要等到执行完毕才会执行后面的代码
//抛出问题:
//如何让服务器服务n个客户端
#endregion
Console.WriteLine("按任意键退出");
Console.ReadKey();
}
}
}
客户端
using System.Collections;
using System.Collections.Generic;
using System.Net;
using System.Net.Sockets;
using System.Text;
using UnityEngine;
public class Lesson6 : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
#region 知识点一 回顾客户端需要做的事情
//1.创建套接字Socket
//2.用Connect方法与客户端相连
//3.用Send和Recive方法收发数据
//4.用Shutdown方法释放连接
//5.关闭套接字
#endregion
#region 知识点二 实现客户端基本逻辑
//1.创建套接字Socket
Socket socketTcp = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
IPEndPoint ipPoint = new IPEndPoint(IPAddress.Parse("127.0.0.1"), 8080);
//2.用Connect方法与客户端相连
try
{
socketTcp.Connect(ipPoint);
}
catch (SocketException e)
{
if (e.ErrorCode == 10061) print("服务器拒绝连接");
else print("服务器连接失败" + e.ErrorCode);
return;
}
//3.用Send和Recive方法收发数据
//接收数据
byte[] receive = new byte[1024];
int receiveNum=socketTcp.Receive(receive);
print("收到服务端发来的消息" + Encoding.UTF8.GetString(receive, 0, receiveNum));
//发送
socketTcp.Send(Encoding.UTF8.GetBytes("你好,我是客户端"));
//4.用Shutdown方法释放连接
socketTcp.Shutdown(SocketShutdown.Both);
//5.关闭套接字
socketTcp.Close();
#endregion
#region 总结
//1.客户端连接的流程每次都是相同的
//2.客户端的Connect、Send、Receive会阻塞主线程,要执行完毕才能继续执行后面的程序
//问题:
//如何让客户端的Socket不影响主线程,并且可以随时收发消息
#endregion
}
// Update is called once per frame
void Update()
{
}
}
结果
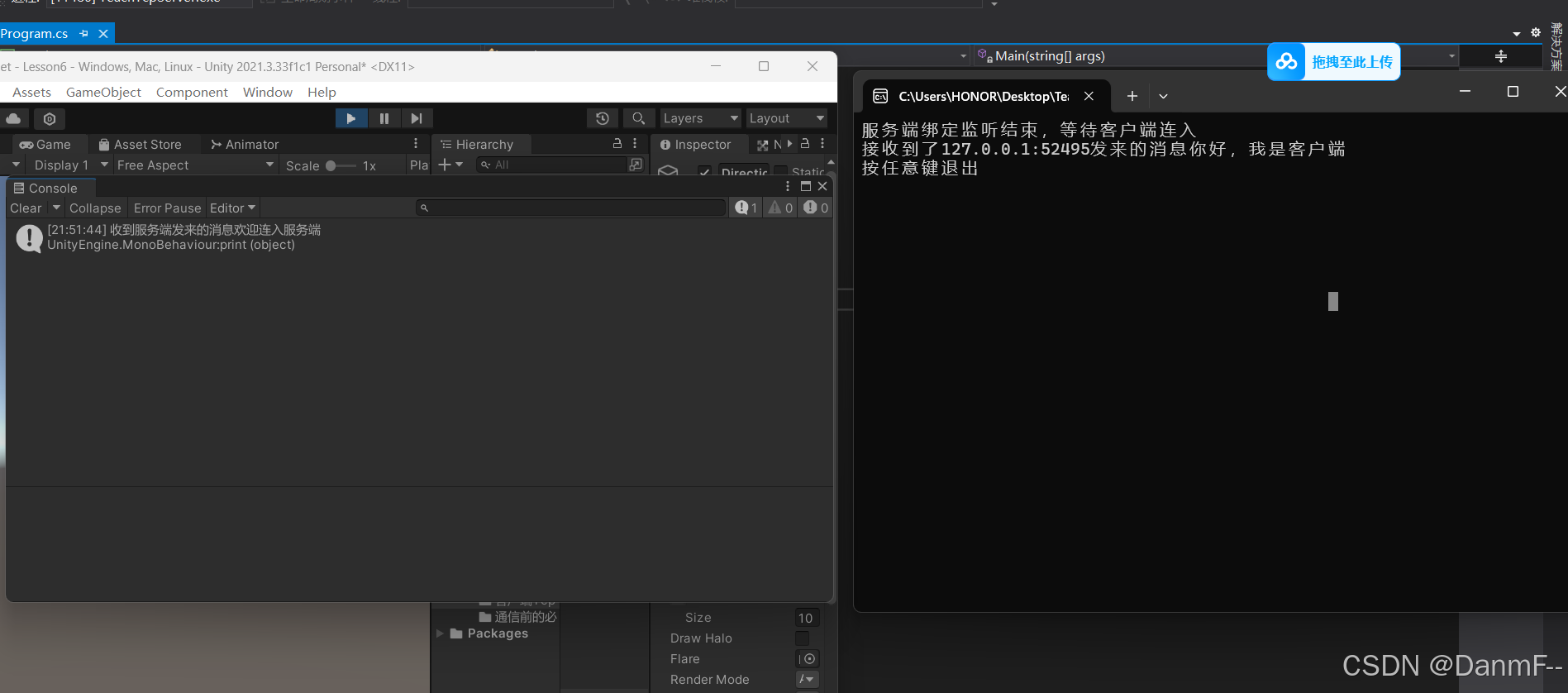