文章目录
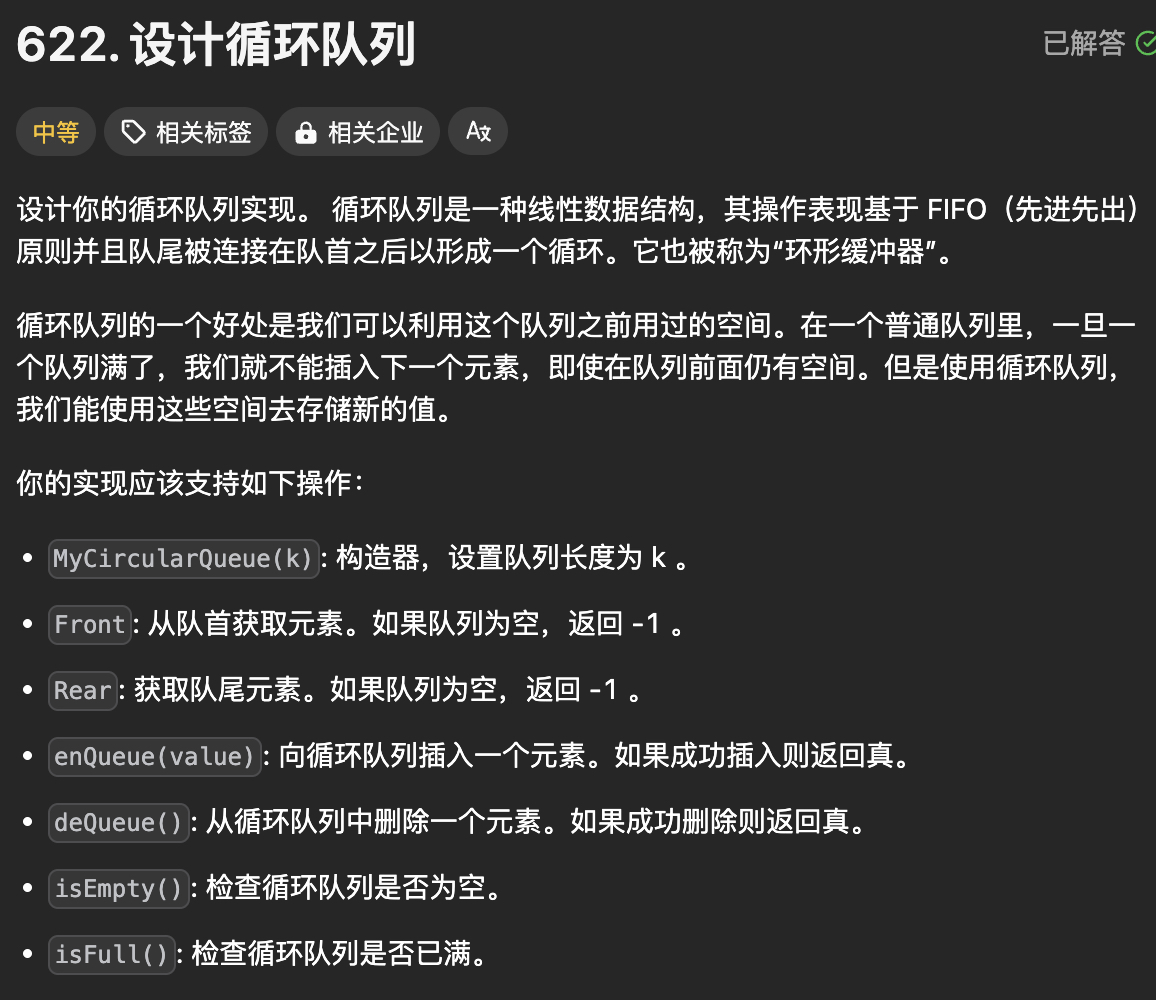
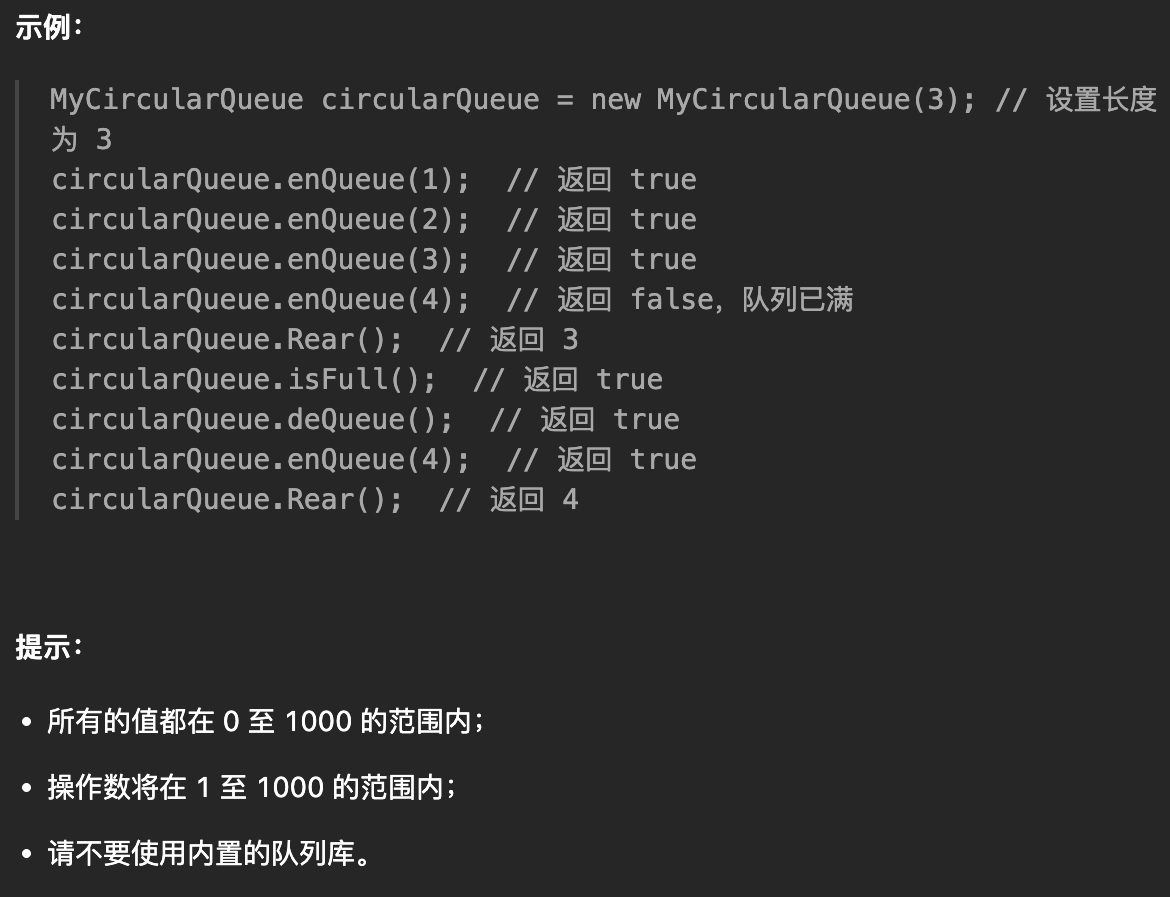
一、数组实现循环队列
1.1 队列
注意:题目要求了 Front() 和 Rear(),当 IsEmpty() 时返回 -1
// go
type MyCircularQueue struct {
l int
r int
len int
cap int
arr []int
}
func Constructor(k int) MyCircularQueue {
return MyCircularQueue {
l: 0,
r: 0,
len: 0,
cap: k,
arr: make([]int, k),
}
}
func (this *MyCircularQueue) EnQueue(value int) bool {
if this.len == this.cap {
return false
}
this.len++
this.arr[this.r]=value
this.r++
if this.r == this.cap {
this.r = 0
}
return true
}
func (this *MyCircularQueue) DeQueue() bool {
if this.len == 0 {
return false
}
this.len--
this.l++
if this.l == this.cap {
this.l = 0
}
return true
}
func (this *MyCircularQueue) Front() int {
if this.IsEmpty() {
return -1
}
return this.arr[this.l]
}
func (this *MyCircularQueue) Rear() int {
if this.IsEmpty() {
return -1
}
i := this.r-1
if i == -1 {
i = this.cap-1
}
return this.arr[i]
}
func (this *MyCircularQueue) IsEmpty() bool {
return this.len == 0
}
func (this *MyCircularQueue) IsFull() bool {
return this.len == this.cap
}
/**
* Your MyCircularQueue object will be instantiated and called as such:
* obj := Constructor(k);
* param_1 := obj.EnQueue(value);
* param_2 := obj.DeQueue();
* param_3 := obj.Front();
* param_4 := obj.Rear();
* param_5 := obj.IsEmpty();
* param_6 := obj.IsFull();
*/
二、多语言解法
C p p / G o / P y t h o n / R u s t / J s / T s Cpp/Go/Python/Rust/Js/Ts Cpp/Go/Python/Rust/Js/Ts
// cpp
// go 同上
# python
// rust
// js
// ts