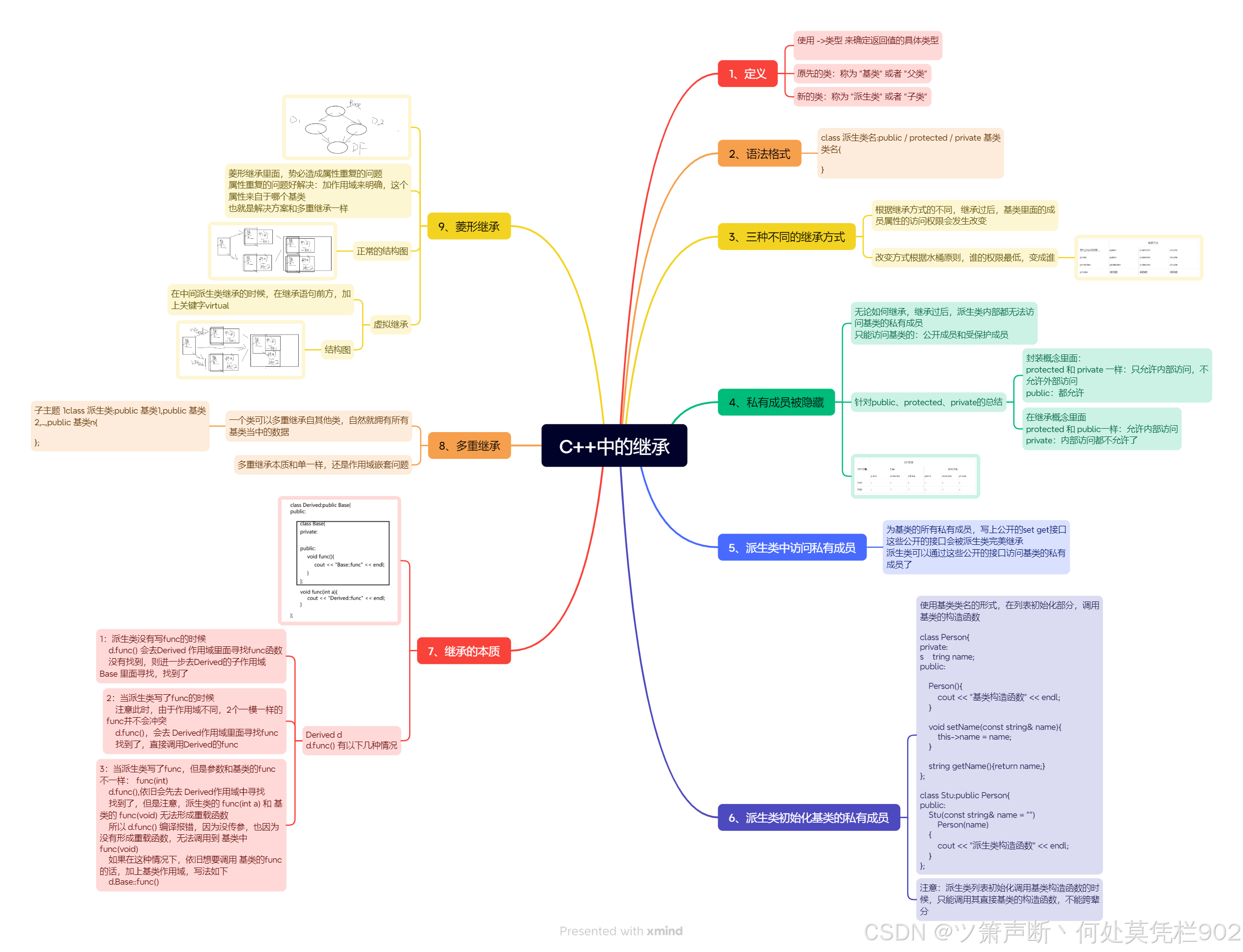
#include <iostream>
using namespace std;
// 武器类(基类)
class Weapon {
protected:
int atk; // 攻击力
public:
// 构造函数
Weapon(int atk = 0) : atk(atk) {}
// 虚拟析构函数
virtual ~Weapon() {}
// set 和 get 接口
void setAtk(int atk) {
this->atk = atk;
}
int getAtk() const {
return atk;
}
};
// 长剑类(继承自武器类)
class Sword : public Weapon {
private:
int hp; // 生命
public:
// 构造函数
Sword(int atk = 0, int hp = 0) : Weapon(atk), hp(hp) {}
// set 和 get 接口
void setHp(int hp) {
this->hp = hp;
}
int getHp() const {
return hp;
}
};
// 短剑类(继承自武器类)
class Blade : public Weapon {
private:
int spd; // 速度
public:
// 构造函数
Blade(int atk = 0, int spd = 0) : Weapon(atk), spd(spd) {}
// set 和 get 接口
void setSpd(int spd) {
this->spd = spd;
}
int getSpd() const {
return spd;
}
};
// 斧头类(继承自武器类)
class Axe : public Weapon {
private:
int def; // 防御
public:
// 构造函数
Axe(int atk = 0, int def = 0) : Weapon(atk), def(def) {}
// set 和 get 接口
void setDef(int def) {
this->def = def;
}
int getDef() const {
return def;
}
};
// 英雄类
class Hero {
private:
int atk; // 攻击力
int def; // 防御力
int spd; // 速度
int hp; // 生命值
Weapon* equippedWeapon; // 当前装备的武器
public:
// 构造函数
Hero(int atk = 0, int def = 0, int spd = 0, int hp = 0)
: atk(atk), def(def), spd(spd), hp(hp), equippedWeapon(nullptr) {}
// 装备武器
void equipWeapon(Weapon* p) {
equippedWeapon = p;
// 根据不同的武器类型进行属性加成
if (dynamic_cast<Sword*>(p)) {
hp += static_cast<Sword*>(p)->getHp();
atk += p->getAtk();
} else if (dynamic_cast<Blade*>(p)) {
spd += static_cast<Blade*>(p)->getSpd();
atk += p->getAtk();
} else if (dynamic_cast<Axe*>(p)) {
def += static_cast<Axe*>(p)->getDef();
atk += p->getAtk();
}
}
// 输出当前英雄的属性
void displayStats() const {
cout << "Hero stats: "
<< "ATK: " << atk << ", "
<< "DEF: " << def << ", "
<< "SPD: " << spd << ", "
<< "HP: " << hp << endl;
}
};
int main() {
// 创建英雄
Hero hero(10, 5, 3, 100);
// 创建武器
Sword sword(5, 20); // 长剑:攻击力5,生命加成20
Blade blade(4, 10); // 短剑:攻击力4,速度加成10
Axe axe(6, 15); // 斧头:攻击力6,防御加成15
// 装备不同的武器并显示英雄状态
hero.equipWeapon(&sword);
hero.displayStats(); // 长剑装备后
hero.equipWeapon(&blade);
hero.displayStats(); // 短剑装备后
hero.equipWeapon(&axe);
hero.displayStats(); // 斧头装备后
return 0;
}
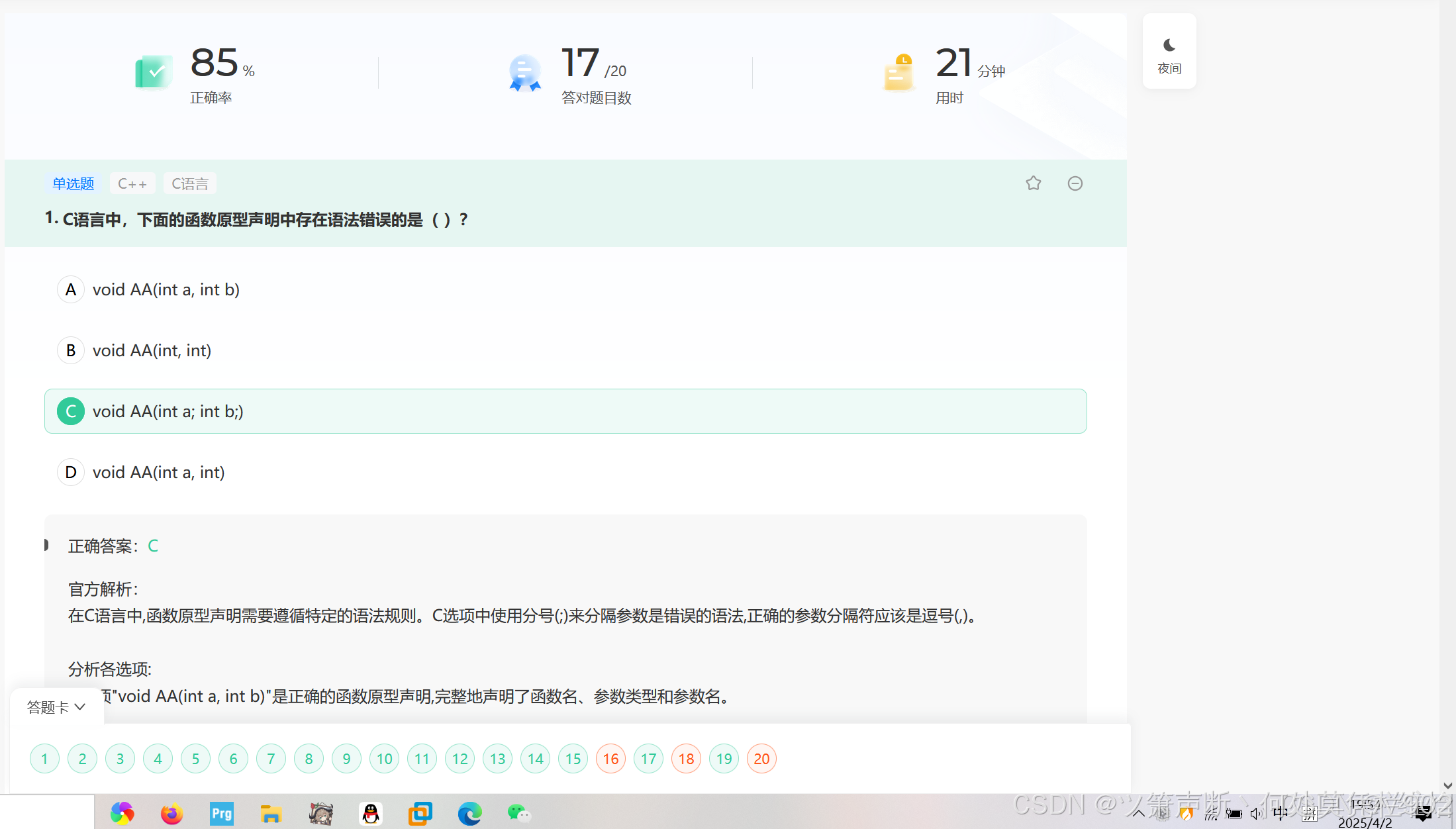