使用lodashjs库
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>页面标题</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.17.21/lodash.min.js"></script>
<style>
.box {
width: 200px;
height: 200px;
background-color: lightblue;
border: 1px solid #000;
display: flex;
justify-content: center;
align-items: center;
font-size: 24px;
}
</style>
</head>
<body>
<div class="box"></div>
<script>
const box = document.querySelector('.box');
let i = 1;
function mouseMove() {
box.innerHTML = i++;
console.log(i);
}
box.addEventListener('mousemove', _.throttle(mouseMove, 3000));
</script>
</body>
</html>
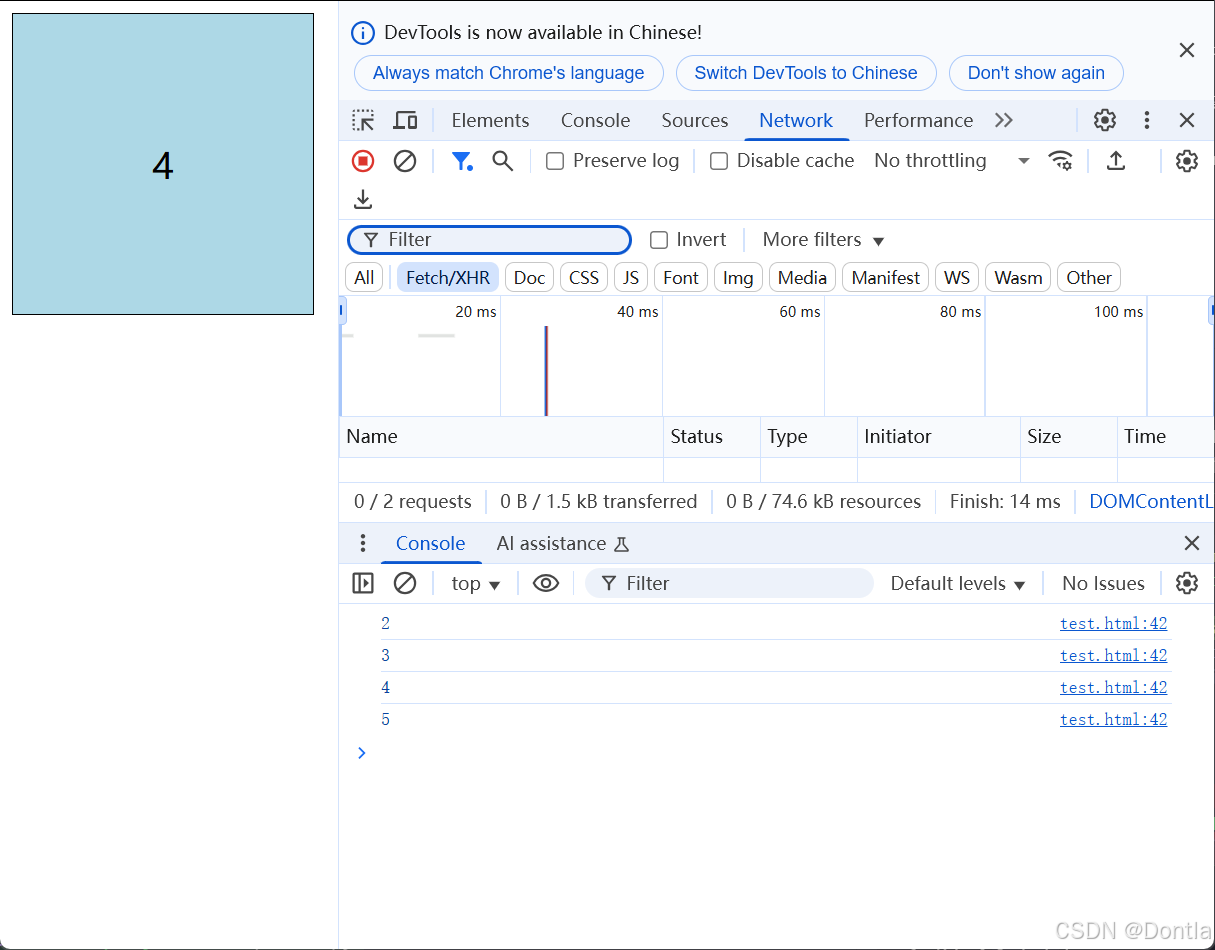
手动实现节流(通过判断之前设定的定时器setTimeout是否存在)
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>页面标题</title>
<style>
.box {
width: 200px;
height: 200px;
background-color: lightblue;
border: 1px solid #000;
display: flex;
justify-content: center;
align-items: center;
font-size: 24px;
}
</style>
</head>
<body>
<div class="box"></div>
<script>
const box = document.querySelector('.box');
let i = 1;
function mouseMove() {
box.innerHTML = i++;
console.log(i);
}
function throttle(fn, delay) {
let timer = null;
return function () {
console.log('判断定时器是否为空?', timer);
if (!timer) {
console.log('定时器为空,延迟执行');
timer = setTimeout(() => {
fn();
timer = null;
}, delay);
}
console.log('不执行');
}
}
box.addEventListener('mousemove', throttle(mouseMove, 3000));
</script>
</body>
</html>
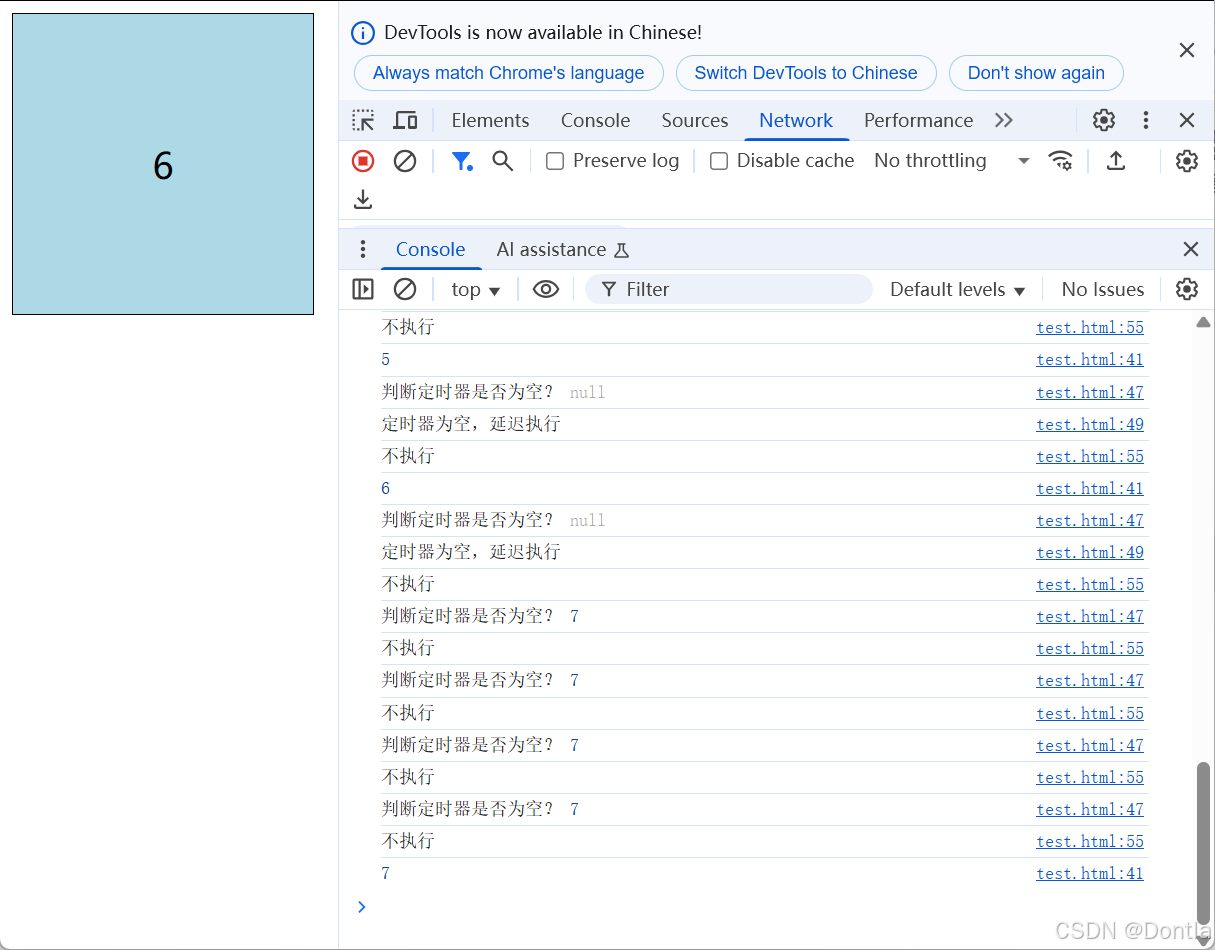