代码
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>页面标题</title>
<link rel="stylesheet" href="styles.css">
<script src="script.js" defer></script>
<style>
.box {
width: 200px;
height: 200px;
background-color: lightblue;
border: 1px solid #000;
display: flex;
justify-content: center;
align-items: center;
font-size: 24px;
}
</style>
</head>
<body>
<div class="box"></div>
<script>
const box = document.querySelector('.box');
let i = 1;
function mouseMove() {
box.innerHTML = i++;
console.log(i);
}
box.addEventListener('mousemove', mouseMove);
</script>
</body>
</html>
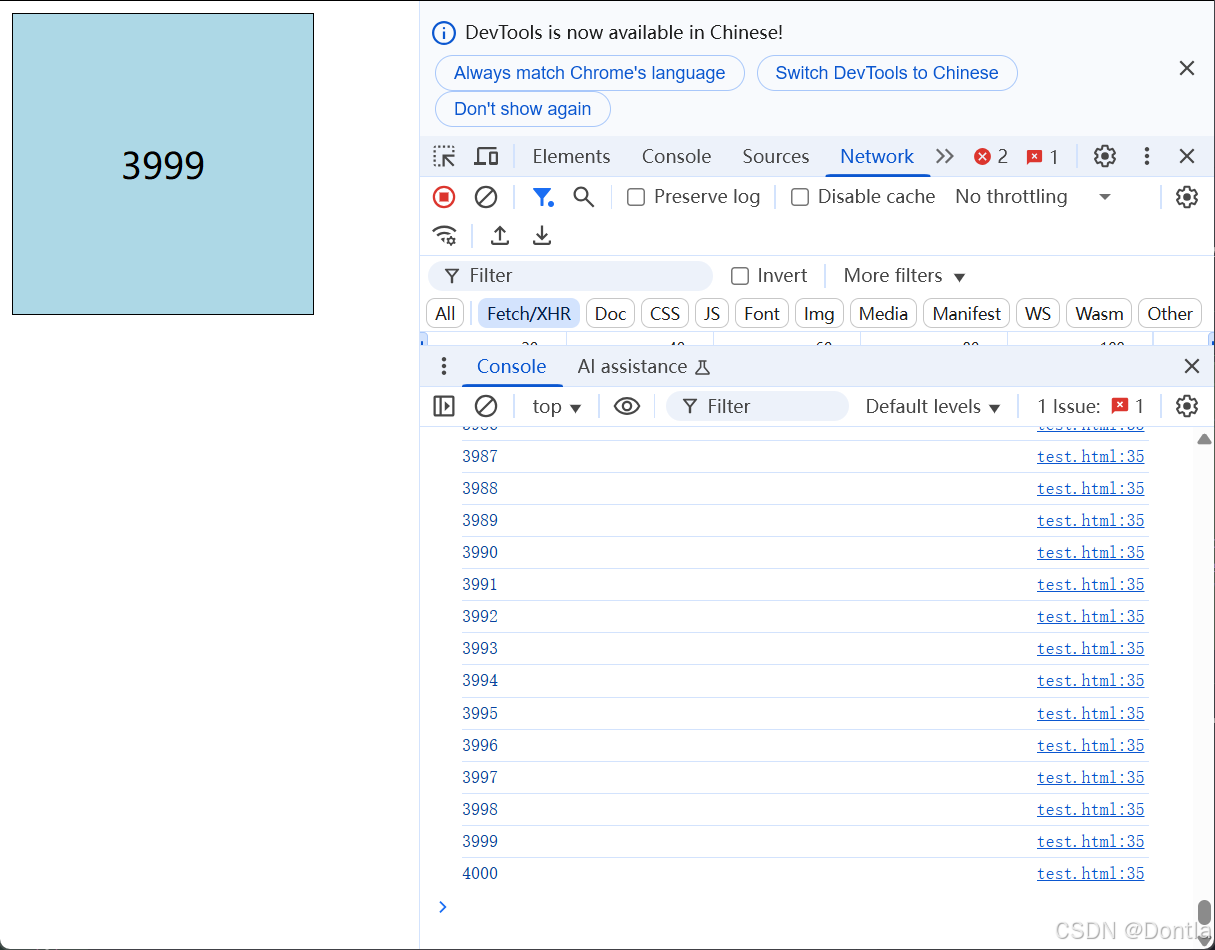
使用lodashjs库debounce函数做防抖处理(只有鼠标移动停止并超过一定时间,才会触发)
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>页面标题</title>
<link rel="stylesheet" href="styles.css">
<script src="https://cdn.jsdelivr.net/npm/lodash@4.17.21/lodash.min.js"></script>
<script src="script.js" defer></script>
<style>
.box {
width: 200px;
height: 200px;
background-color: lightblue;
border: 1px solid #000;
display: flex;
justify-content: center;
align-items: center;
font-size: 24px;
}
</style>
</head>
<body>
<div class="box"></div>
<script>
const box = document.querySelector('.box');
let i = 1;
const mouseMove = _.debounce(function() {
box.innerHTML = i++;
}, 300);
box.addEventListener('mousemove', mouseMove);
</script>
</body>
</html>
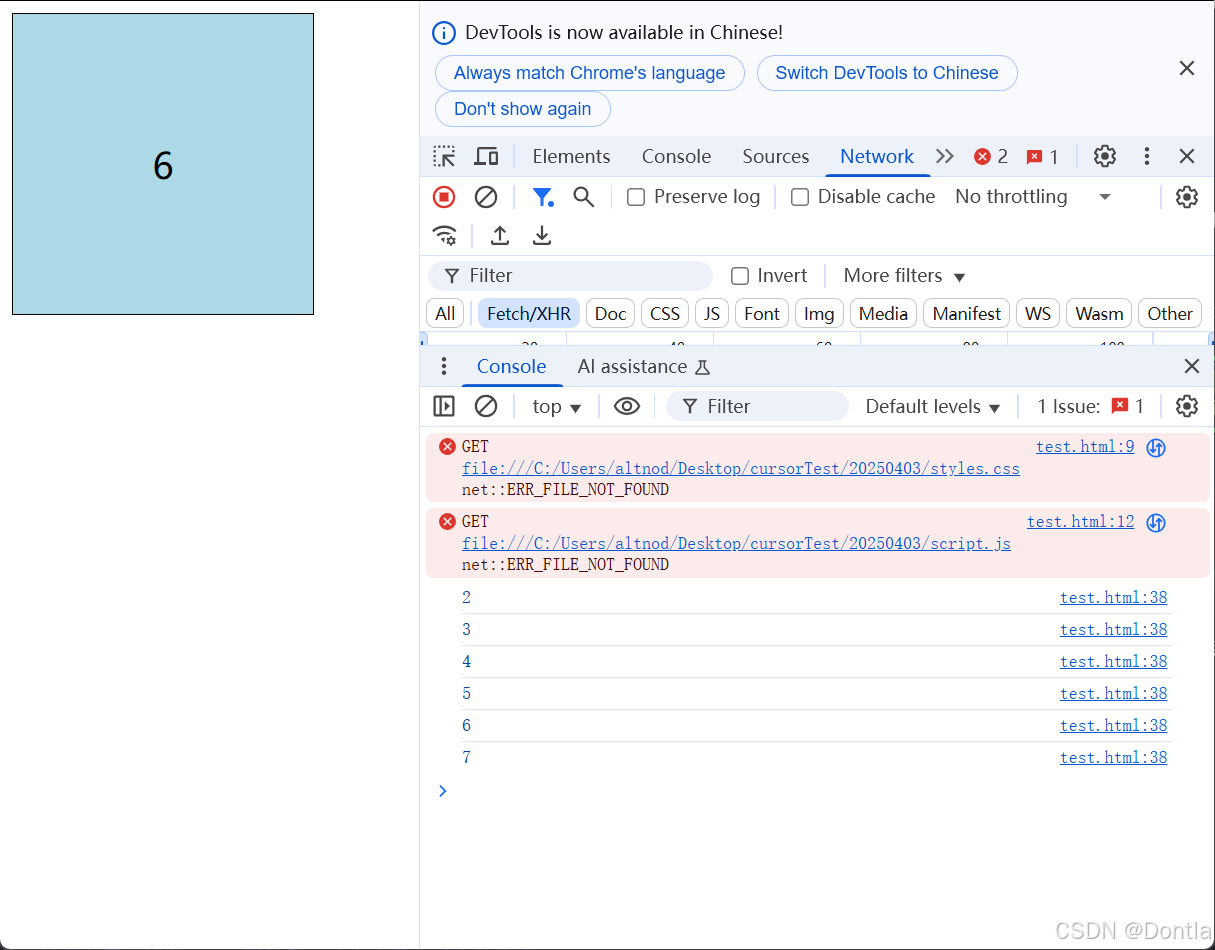
手写防抖函数
// 手写防抖函数
// 核心是利用 setTimeout定时器来实现
// 1.声明定时器变量
// 2.每次鼠标移动(事件触发)的时候都要先判断是否有定时器,如果有先清除以前的定时器
// 3.如果没有定时器,则开启定时器,存入到定时器变量里面
// 4.定时器里面写函数调用
写法1
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>页面标题</title>
<link rel="stylesheet" href="styles.css">
<script src="script.js" defer></script>
<style>
.box {
width: 200px;
height: 200px;
background-color: lightblue;
border: 1px solid #000;
display: flex;
justify-content: center;
align-items: center;
font-size: 24px;
}
</style>
</head>
<body>
<div class="box"></div>
<script>
const box = document.querySelector('.box');
let i = 1;
let timer;
const mouseMove = function() {
if (timer) {
clearTimeout(timer);
}
timer = setTimeout(() => {
box.innerHTML = i++;
console.log(i);
}, 300);
};
box.addEventListener('mousemove', mouseMove);
</script>
</body>
</html>
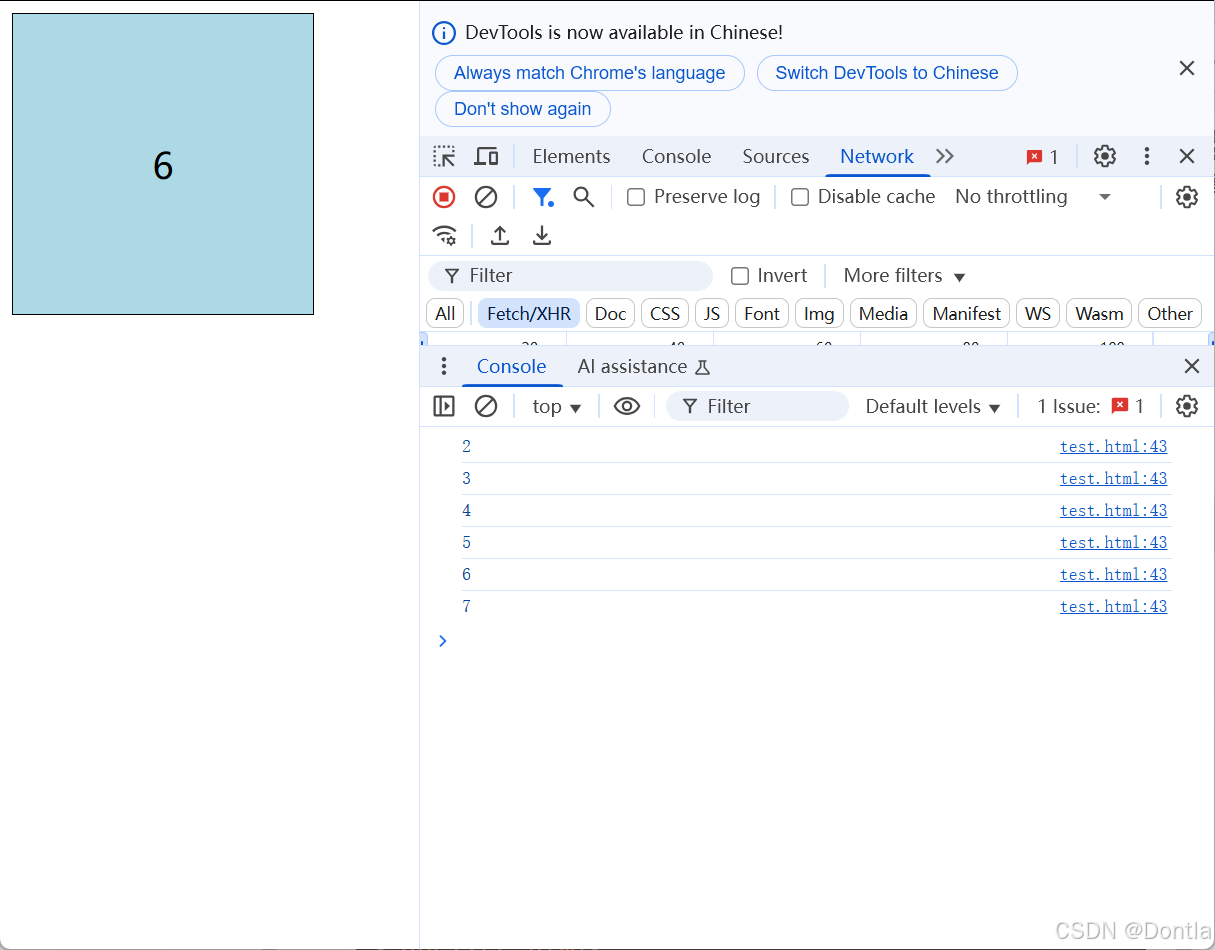
写法2(注意addEventListener监听函数的第二个参数接收的是一个函数,需要构造一个匿名返回函数)
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>页面标题</title>
<style>
.box {
width: 200px;
height: 200px;
background-color: lightblue;
border: 1px solid #000;
display: flex;
justify-content: center;
align-items: center;
font-size: 24px;
}
</style>
</head>
<body>
<div class="box"></div>
<script>
const box = document.querySelector('.box');
let i = 1;
let timer;
function mouseMove() {
box.innerHTML = i;
i++;
console.log(i);
}
function debounce(fn, delay) {
return function () {
console.log("防抖函数被调用");
if (timer) {
clearTimeout(timer);
}
timer = setTimeout(() => {
fn();
}, delay);
};
}
box.addEventListener('mousemove', debounce(mouseMove, 300));
</script>
</body>
</html>
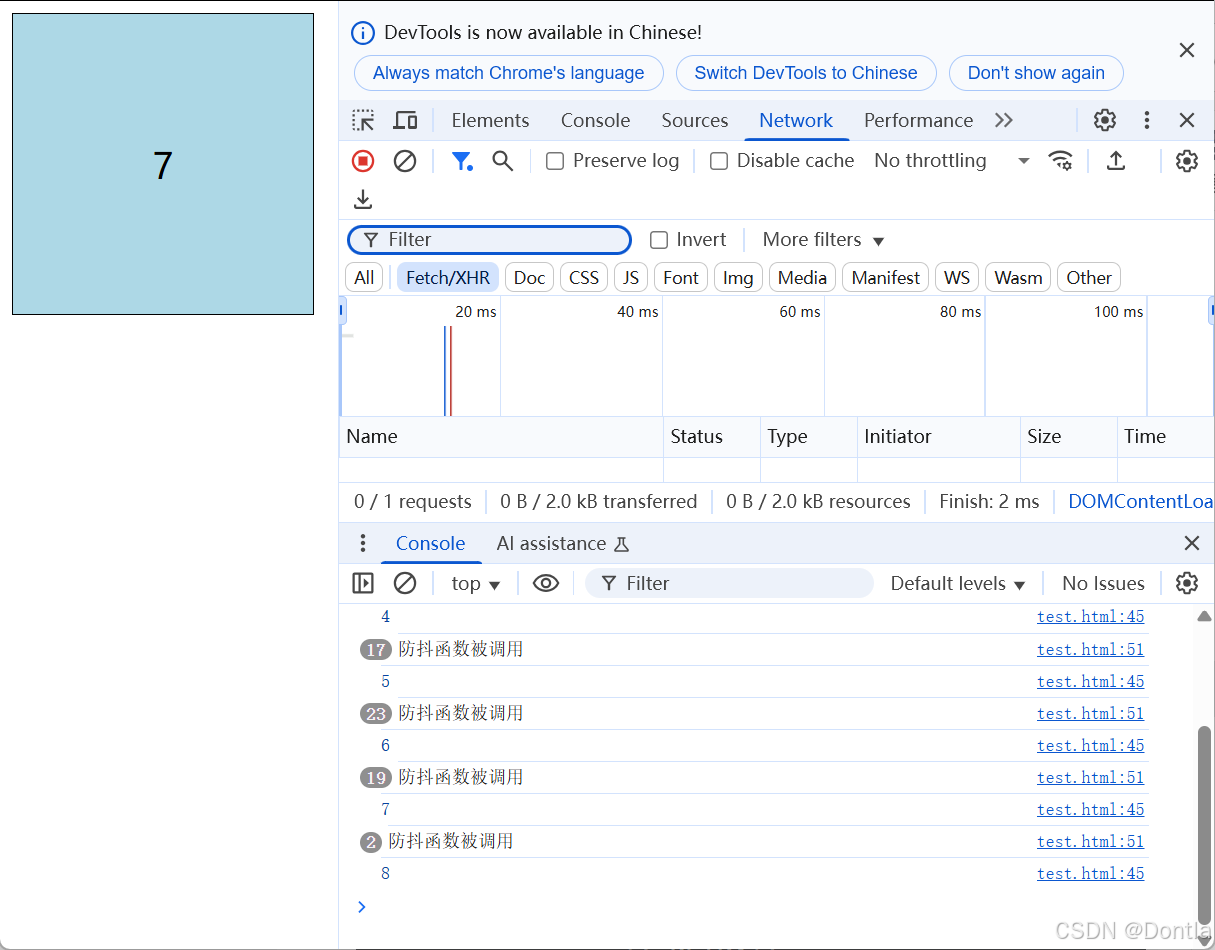