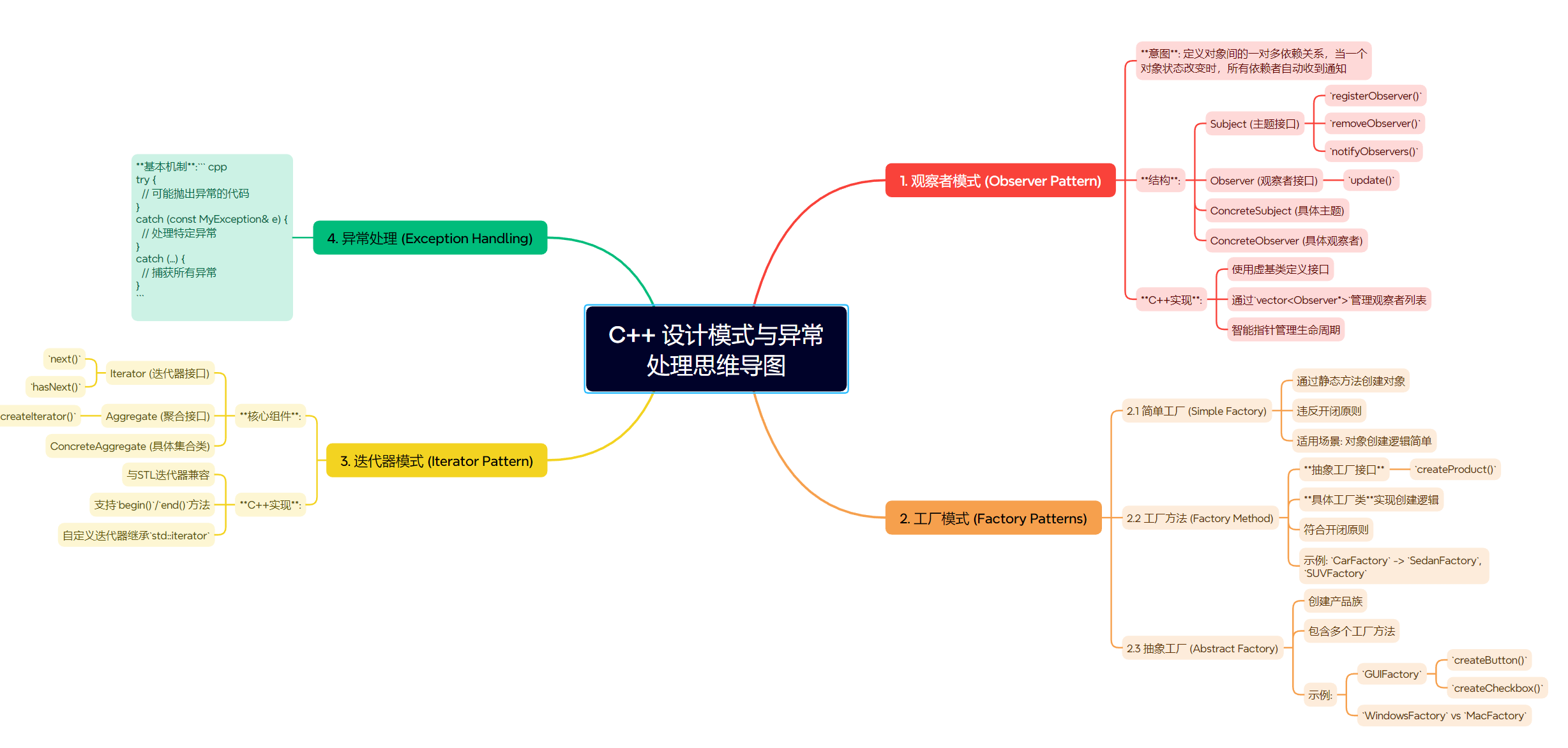
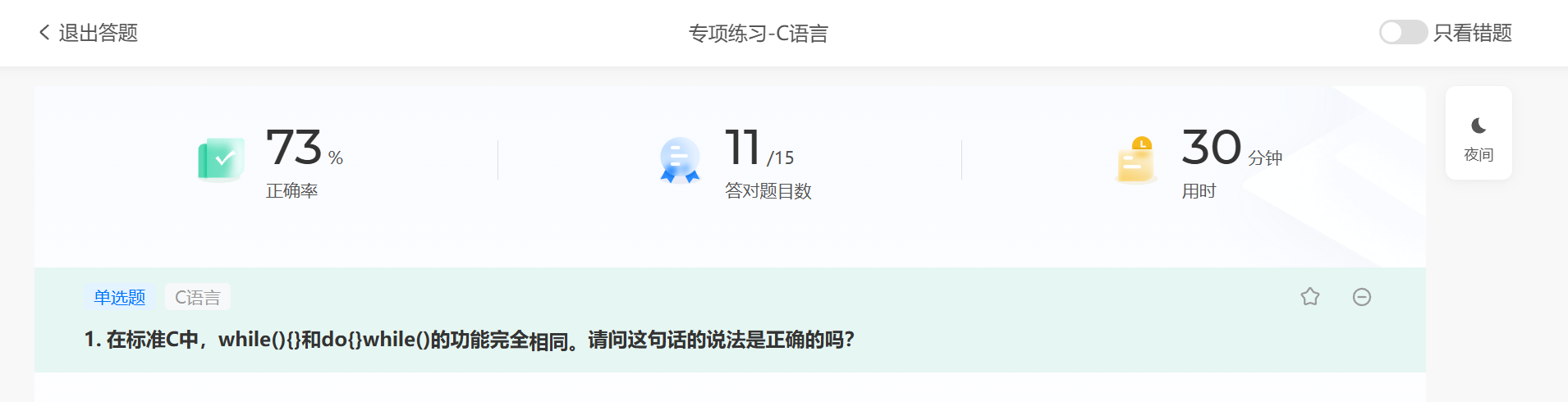
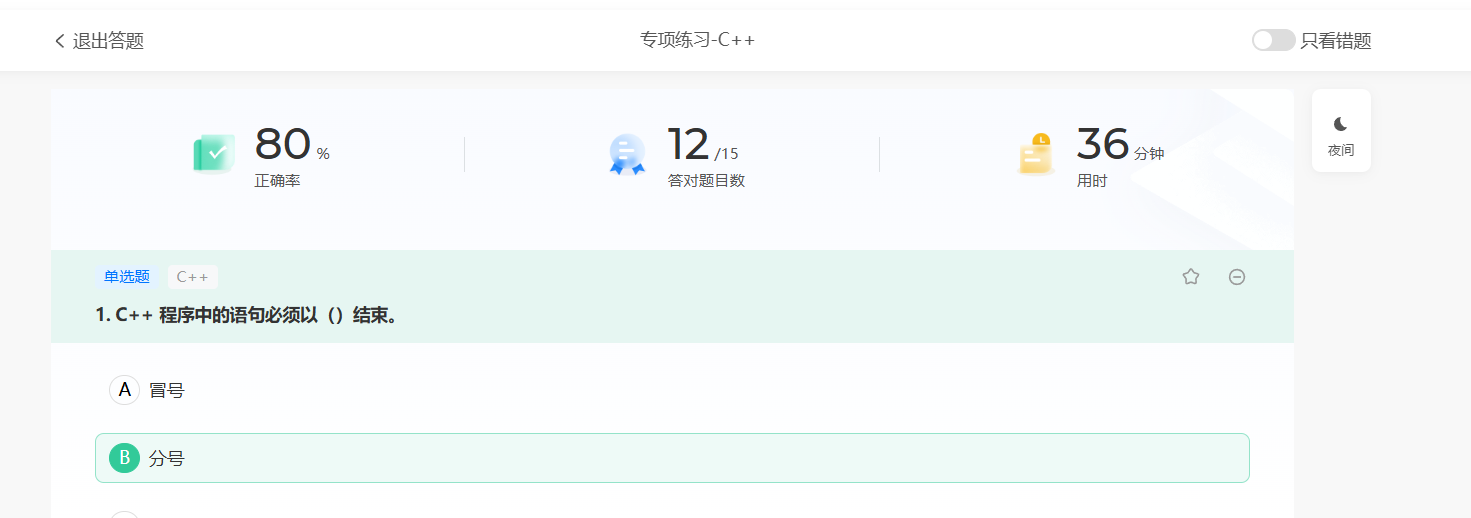
#include <iostream>
#include <cstring>
#include <cstdlib>
#include <unistd.h>
#include <sstream>
#include <vector>
#include <memory>
using namespace std;
class myException:public exception{
private:
public:
const char* what()const noexcept{
return "越界访问";
}
};
template <class T>
class myList{
public:
struct Node{
T val;
Node* next;
Node* prev;
};
class iterator{
private:
Node* ptr;
public:
iterator(Node* ptr=NULL);
T& operator*();
bool operator!=(const iterator& r);
iterator& operator++(int);
iterator& operator++();
};
myList();
void push_back(const T& val);
myList& operator<<(const T& val);
T& operator[](int index);
int size();
iterator begin();
iterator end();
private:
Node* head; //真正的链表(链表头头节点)
Node* tail; // 链表尾节点
int count;
};
//===========================================
//迭代器代码
template <typename T>
myList<T>::iterator::iterator(Node* ptr)
:ptr(ptr){}
template <typename T>
T& myList<T>::iterator::operator*(){
return ptr->val;
}
template <typename T>
bool myList<T>::iterator::operator!=(const iterator& r){
return ptr!=r.ptr;
}
template <typename T>
typename myList<T>::iterator& myList<T>::iterator::operator++(int){
if(ptr==NULL)
{
throw myException();
}
ptr=ptr->next;
return *this;
}
template <typename T>
typename myList<T>::iterator& myList<T>::iterator::operator++(){
if(ptr==NULL)
{
throw myException();
}
ptr=ptr->next;
return *this;
}
template <typename T>
typename myList<T>::iterator myList<T>::begin(){
iterator it(head->next);
return it;
}
template <typename T>
typename myList<T>::iterator myList<T>::end(){
iterator it(tail->next);
return it;
}
template <typename T>
myList<T>::myList(){
head = new Node;
head->next = NULL;
head->prev = NULL;
tail = head; // 只有头节点的情况下,尾节点即使头节点
count = 0;
}
template <typename T>
void myList<T>::push_back(const T& val){
Node* newnode = new Node;
newnode->val = val;
newnode->next = NULL;
newnode->prev = tail;
tail->next = newnode;
tail = newnode;
count ++;
}
template <typename T>
myList<T>& myList<T>::operator<<(const T& val){
push_back(val);
// return 0
return *this;
}
template <typename T>
T& myList<T>::operator[](int index){
if(index<0 || index>=count){
throw myException();
}
Node* p = head->next;
for(int i=0;i<index;i++){
p = p->next;
}
return p->val;
}
template <typename T>
int myList<T>::size(){
return count;
}
int main(int argc,const char** argv){
myList<int> l;
l << 1 << 3 << 5 << 7 << 9;
try{
cout << l[5] << endl;
}catch(const myException& e){
cout << "捕获异常:" << e.what() <<endl;
}
try{
myList<int>::iterator it=l.end();
++it;
}catch(const myException& e){
cout << "捕获异常:" << e.what() <<endl;
}
return 0;
}