一些可能想知道的:
cryptography库:密码学工具包
Fernet 是crytography 里的一个模块,用于对称加密
with open() as file #为了保证无论是否出错都能正确地关闭文件,与try...finally...相同
open() #用于读文件(默认情况下只读 “r”)
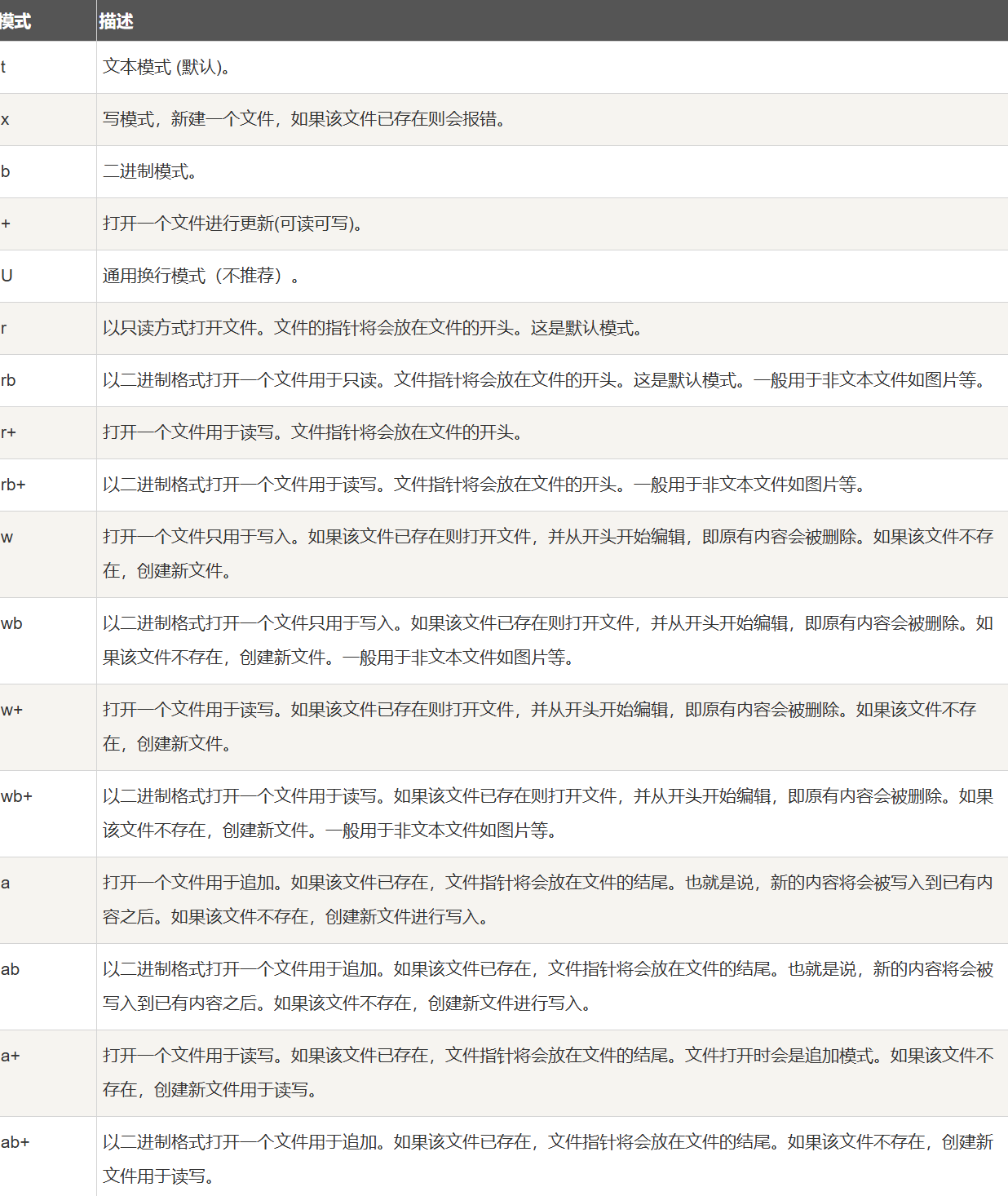
Python 的 f-string(格式化字符串字面量)将变量动态插入到字符串中。
通过 if __name__ == "__main__"
的条件判断,可以确保 main
函数仅在文件被直接运行时执行,而不会在导入时触发。
典:文件加密与解密
#加入模块:Fernet 对称加密
from cryptography.fernet import Fernet
#加密操作
def encrypt_file(file_path,key):
with open(file_path,'rb') as file :
#明文读取
plaintext = file.read()
#密钥及密文生成
cipher_suite = Fernet(key)
ciphertext = cipher_suite.encrypt(plaintext)
#生成加密后的输出路径和文件
output_file = f"{file_path}.encrypted"
with open(output_file,'wb')as file:
file.write(ciphertext)
# 将生成的密钥生成文件为了后续解密
key_file = f"{file_path}.key"
with open(key_file, 'wb') as file:
file.write(key)
print(f"加密完成,加密后的文章保存为:{output_file}")
print(f"密钥已经保存到:{key_file}")
#解密操作
def decrypt_file(file_path,key):
with open(file_path,'rb') as file:
ciphertext = file.read()
cipher_suite = Fernet(key)
plaintext = cipher_suite.decrypt(ciphertext)
output_file = f"{file_path}.decrypted"
with open(output_file,'wb') as file:
file.write(plaintext)
print(f"解密完成,解密后的文件保存为:{output_file}")
def main():
print("欢迎使用文件加密和解密程序!")
print("1.加密文件")
print("2.解密文件")
choice = input("请选择操作(1/2):")
if choice == '1':
file_path = input("请输入要加密的文件路径:")
key = Fernet.generate_key()
encrypt_file(file_path,key)
elif choice =='2':
file_path = input("请输入要解密的文件路径:")
#encode() 用于文件路径处理:若输入的是文件路径,需要字节路径来打开文件
key = input("请输入密钥:")
decrypt_file(file_path,key)
else:
print("无效的选项,请重新运行程序")
if __name__ == "__main__":
main()
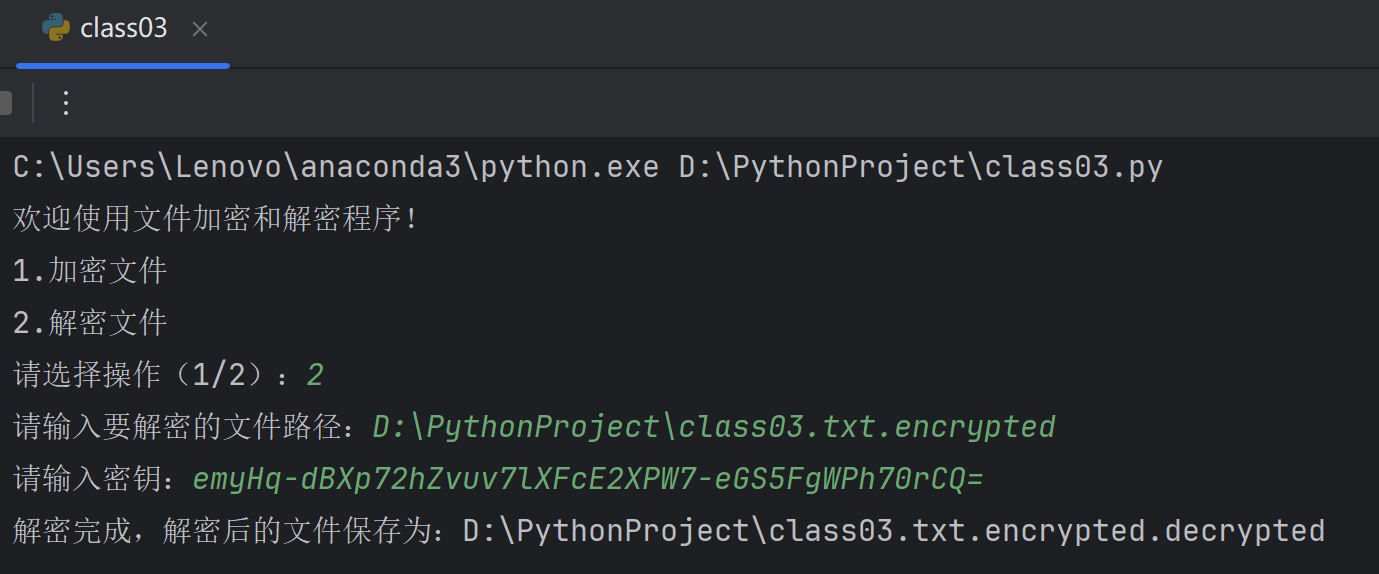
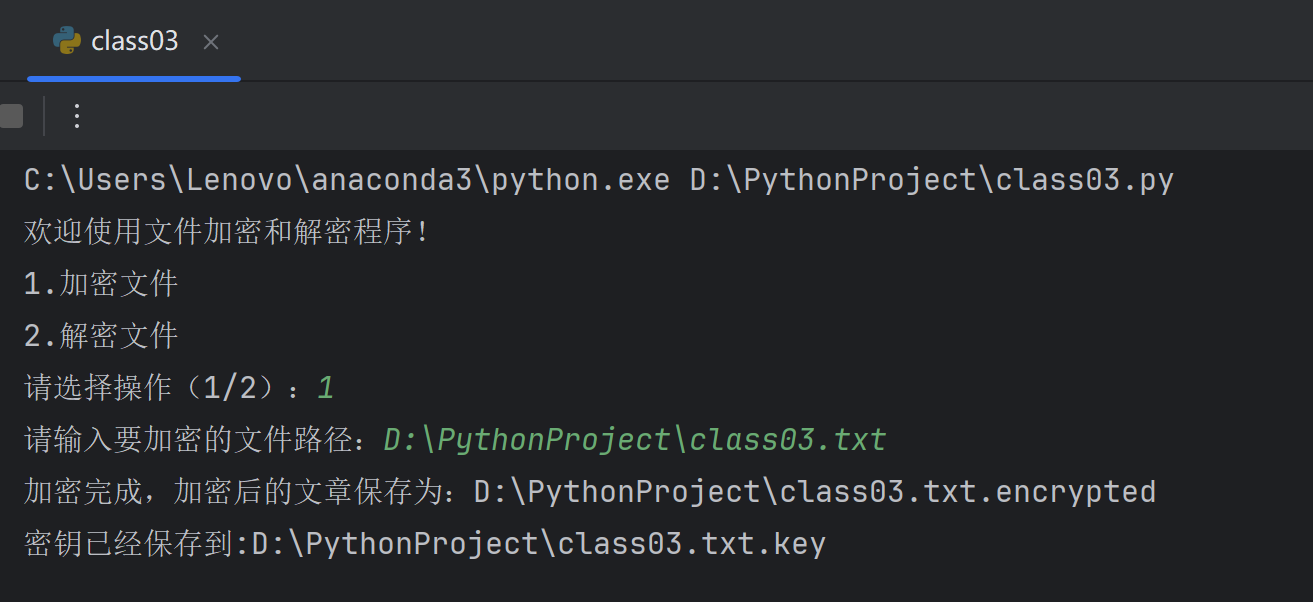