~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
一. Stream流
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
1.Stream流的概述和获取方式
① 概述
② 获取方式
2. Stream流的常用API
API代码演示:
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("张无忌");
list.add("周芷若");
Stream<String> filter = list.stream().filter(s -> s.startsWith("张"));
Stream<String> limit = list.stream().limit(3);
Stream<String> skip = list.stream().skip(3);
Stream<String> distinct = list.stream().distinct();
// map加工方法: 第一个参数原材料 -> 第二个参数是加工后的结果。
// 给集合元素的前面都加上一个:黑马的:
list.stream().map(s -> "黑马的:" + s).forEach(a -> System.out.println(a));
Stream<String> concat = Stream.concat(filter, limit);
list.stream().forEach(s -> System.out.println(s));
//与上行一 list.stream().forEach(System.out::println);
long num = list.stream().count();
}
3. Stream流的综合应用
public class Test {
public static double oneallMoeny = 0;
public static double allMoeny = 0;
public static void main(String[] args) {
List<Employee> one = new ArrayList<>();
one.add(new Employee("猪八戒", '男', 30000, 25000, null));
one.add(new Employee("孙悟空", '男', 25000, 1000, "顶撞上司"));
one.add(new Employee("沙僧", '男', 20000, 20000, null));
one.add(new Employee("小白龙", '男', 20000, 25000, null));
List<Employee> two = new ArrayList<>();
two.add(new Employee("武松", '男', 15000, 9000, null));
two.add(new Employee("李逵", '男', 20000, 10000, null));
two.add(new Employee("西门庆", '男', 50000, 100000, "被打"));
two.add(new Employee("潘金莲", '女', 3500, 1000, "被打"));
two.add(new Employee("武大郎", '女', 20000, 0, "下毒"));
//优秀员工
Employee E = one.stream().max((o1, o2) -> Double.compare(o1.getSalary() + o1.getBonus(), o2.getSalary() + o2.getBonus()))
.map(e -> new Employee(e.getName(), e.getSex(), e.getSalary(), e.getBonus(), e.getPunish())).get();
System.out.println(E);
//一部平均工资
one.stream().sorted((o1, o2) -> Double.compare(o1.getSalary() + o1.getBonus(), o2.getSalary() + o2.getBonus()))
.skip(1).limit(one.size() - 2).forEach(e -> {
oneallMoeny += e.getSalary() + e.getBonus();
});
System.out.println("一部平均工资:" + oneallMoeny / (one.size() - 2));
//两个部门平均工资
Stream<Employee> all = Stream.concat(one.stream(), two.stream());
all.sorted((o1, o2) -> Double.compare(o1.getSalary() + o1.getBonus(), o2.getSalary() + o2.getBonus()))
.skip(1).limit((one.size() + two.size()) - 2).forEach(e -> {
allMoeny += e.getSalary() + e.getBonus();
});
//使用BigDecimal计算
BigDecimal big = BigDecimal.valueOf(allMoeny);
BigDecimal num = BigDecimal.valueOf(one.size() + two.size() - 2);
System.out.println("两个部门平均工资:" + big.divide(num,RoundingMode.HALF_UP));
}
}
4. 收集Stream流
public class StreamDemo05 {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("张无忌");
list.add("周芷若");
list.add("赵敏");
list.add("张三丰");
// * 注意注意注意:“流只能使用一次” *
//1. 转List
Stream<String> s1 = list.stream().filter(s -> s.startsWith("张"));
List<String> zhangList = s1.collect(Collectors.toList()); // 可变集合
zhangList.add("java1");
System.out.println(zhangList);
/* List<String> list1 = s1.toList(); // 得到不可变集合
list1.add("java");
System.out.println(list1);*/
//2. 转set集合
Stream<String> s2 = list.stream().filter(s -> s.startsWith("张"));
Set<String> zhangSet = s2.collect(Collectors.toSet());
System.out.println(zhangSet);
//3. 转数组
Stream<String> s3 = list.stream().filter(s -> s.startsWith("张"));
//3.1 转数组默认转成Object
Object[] arrs = s3.toArray();
//3.2 强行转指定类型
String[] arrs1 = s3.toArray(new IntFunction<String[]>() {
@Override
public String[] apply(int value) {
return new String[value];
}
});
String[] arrs2 = s3.toArray(String[]::new);
System.out.println("Arrays数组内容:" + Arrays.toString(arrs2));
}
}
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
二. 异常处理
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
1. 常见运行时异常
public static void main(String[] args) {
/** 2.空指针异常 : NullPointerException。直接输出没有问题。但是调用空指针的变量的功能就会报错!! */
String name = null;
System.out.println(name); // null
// System.out.println(name.length()); // 运行出错,程序终止
/** 3.类型转换异常:ClassCastException。 */
Object o = 23;
// String s = (String) o; // 运行出错,程序终止
/** 5.数学操作异常:ArithmeticException。 */
//int c = 10 / 0;
/** 6.数字转换异常: NumberFormatException。 */
//String number = "23";
String number = "23aabbc";
Integer it = Integer.valueOf(number); // 运行出错,程序终止
System.out.println(it + 1);
}
2.常见编译时异常
public static void main(String[] args) throws ParseException {
String date = "2015-01-12 10:23:21";
// 创建一个简单日期格式化类:
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
// 解析字符串时间成为日期对象
Date d = sdf.parse(date);
System.out.println(d);
}
3. 编译时异常的处理机制
① 异常处理方式1 —— throws
public class ExceptionDemo01 {
public static void main(String[] args) throws Exception {
System.out.println("程序开始。。。。。");
parseTime("2011-11-11 11:11:11");
System.out.println("程序结束。。。。。");
}
public static void parseTime(String date) throws Exception {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date d = sdf.parse(date);
System.out.println(d);
InputStream is = new FileInputStream("E:/meinv.jpg");
}
}
②. 异常处理方式2 —— try…catch…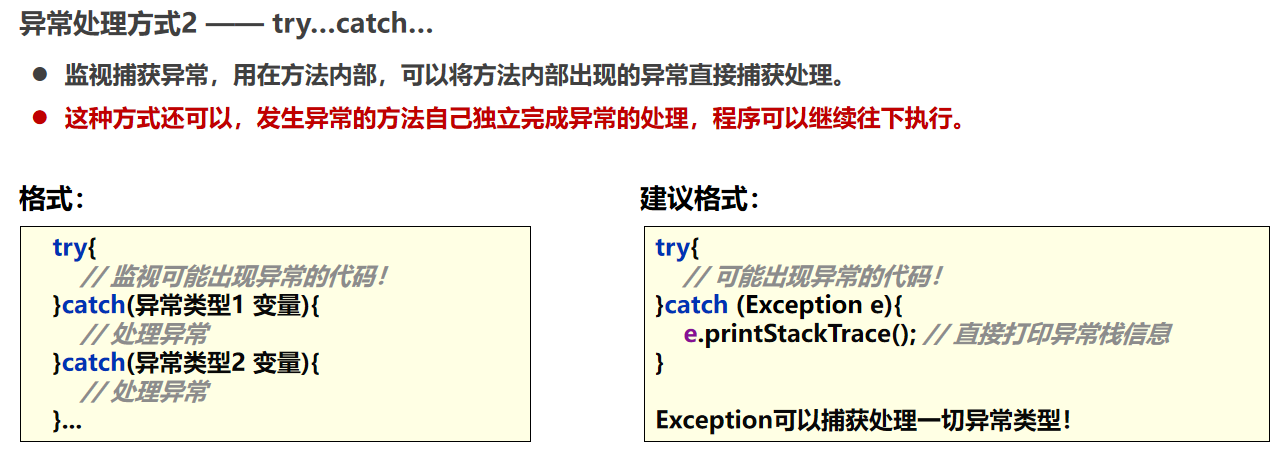
public class ExceptionDemo02 {
public static void main(String[] args) {
System.out.println("程序开始。。。。");
parseTime("2011-11-11 11:11:11");
System.out.println("程序结束。。。。");
}
public static void parseTime(String date) {
try {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy/MM-dd HH:mm:ss");
Date d = sdf.parse(date);
System.out.println(d);
InputStream is = new FileInputStream("E:/meinv.jpg");
} catch (Exception e) {
e.printStackTrace(); // 打印异常栈信息
}
}
}
③. 异常处理方式3 —— 前两者结合
public class ExceptionDemo03 {
public static void main(String[] args) {
System.out.println("程序开始。。。。");
try {
parseTime("2011-11-11 11:11:11");
System.out.println("功能操作成功~~~");
} catch (Exception e) {
e.printStackTrace();
System.out.println("功能操作失败~~~");
}
System.out.println("程序结束。。。。");
}
public static void parseTime(String date) throws Exception {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy、MM-dd HH:mm:ss");
Date d = sdf.parse(date);
System.out.println(d);
InputStream is = new FileInputStream("D:/meinv.jpg");
}
}
4. 运行时异常的处理机制
public class Test {
public static void main(String[] args) {
System.out.println("程序开始。。。。。。。。。。");
try {
chu(10, 0);
} catch (Exception e) {
e.printStackTrace();
}
System.out.println("程序结束。。。。。。。。。。");
}
public static void chu(int a , int b) { // throws RuntimeException{
System.out.println(a);
System.out.println(b);
int c = a / b;
System.out.println(c);
}
}
5. 异常处理使代码更稳健的案例
//需求: 键盘录入一个合理的价格为止(必须是数值,值必须大于0)
public class Test {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
while (true) {
try {
System.out.println("请输入合理的价格:");
String priceStr = sc.nextLine();
double price = Double.parseDouble(priceStr);
if (price > 0) {
System.out.println("对");
break;
} else {
System.out.println("错");
}
} catch (NumberFormatException e) {
e.printStackTrace();
}
}
}
}
6. 自定义异常
//自定义编译时异常
public class Excetption extends Exception{
public Excetption() {
}
public Excetption(String message) {
super(message);
}
}
//自定义运行时异常
public class RunTimeException extends RuntimeException {
public RunTimeException() {
}
public RunTimeException(String message) {
super(message);
}
}
//测试类
public class Test {
public static void main(String[] args) throws Exception{
//编译异常:即使输入合法,也会在编译阶段的时候会报错,目的强烈提醒程序员可能出错,需要在上层继续抛出
checkAge(12);
//运行时异常
checkAge2(23);
}
//运行时异常
public static void checkAge2(int b){
if (b<0 || b>200){
throw new RunTimeException(b+" is cuo");
}else {
System.out.println("年龄合法~");
}
}
//编译时候异常
public static void checkAge(int a) throws Excetption {
if (a<0 || a>200){
throw new Excetption(a+" is cuo");
}else {
System.out.println("年龄合法~");
}
}
}
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
三. 日志框架
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~