目录
时间对象
实例化
// 时间对象
// 实例化 代码中出现了new,这一操作称为实例化
// 获得当前时间
let date = new Date()
console.log(typeof date);
document.write(date)

时间对象方法
注意:
获得月份 0-11,需要+1获得当前月份
getDay());//0-6 0是周日,6是周六
let date = new Date()
// 获得年份
let year = date.getFullYear()
// console.log(year);
// 获得月份 0-11,需要+1获得当前月份
let month = date.getMonth() + 1
// console.log(month);
//获得几号 1-31
let date1 = date.getDate()
// console.log(date1);
// 时分秒
let hour = date.getHours() //0-23
// console.log(hour);
let min = date.getMinutes() //0-59
// console.log(min);
let sec = date.getSeconds() //0-59
// console.log(sec);
// 星期几
const arr = ['周日', '周一', '周二', '周三', '周四', '周五', '周六']
let day = arr[date.getDay()]
// console.log(date.getDay());//0-6 0是周日,6是周六
时间戳
时间戳 从1970年1月1日0点开始的到现在的毫秒数
// 计算倒计时
// 核心思想:
// 将来时间 有一个时间戳
// 现在时间 有一个时间戳
// 将来时间戳减去现在时间戳,就是剩余的时间戳
// 获取时间戳
// 第一种方法
let date = new Date()
console.log(date.getTime());
// 第二种方法
// 当前
console.log(+new Date());
// 指定时间
console.log(+new Date('2022-8-10 12:30:00'));
// 第三种方法
// 这种只能得到当前时间戳
console.log(Date.now());
数组的map和join方法
map
// map方法会边路数组
// 数组.map(function(item,i){})
// item是数组元素
// i是下标
// map方法返回的是 对原数组处理过的新数组
// 作用:对数组里面的元素进行加工处理
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
// map方法会边路数组
// 数组.map(function(item,i){})
// item是数组元素
// i是下标
// map方法返回的是 对原数组处理过的新数组
// 作用:对数组里面的元素进行加工处理
const arr = ['爱仕达', '撒大大', '甜热热', '尔特']
const newArr = arr.map(function (item, i) {
return item + '大家好'
})
console.log(newArr);
</script>
</body>
</html>
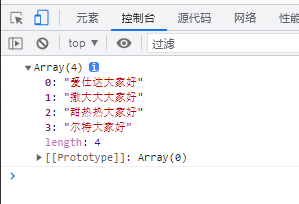
join
// 数组join方法
// 数组名.join([参数])
// 作用:将数据中的元素连接成一个字符串,如果join没有参数,则使用逗号连接
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<ul></ul>
<script>
// 数组join方法
// 数组名.join([参数])
// 作用:将数据中的元素连接成一个字符串,如果join没有参数,则使用逗号连接
const arr = ['爱仕达', '撒大大', '甜热热', '尔特']
const str = arr.join('💎')
document.write(str)
// 需求:根据数据渲染html
// 1.先用map方法对数组里面的元素加工处理
const newArr = arr.map(function (item, i) {
return `<li>${item}</li>`
})
document.querySelector('ul').innerHTML = newArr.join('')
</script>
</body>
</html>
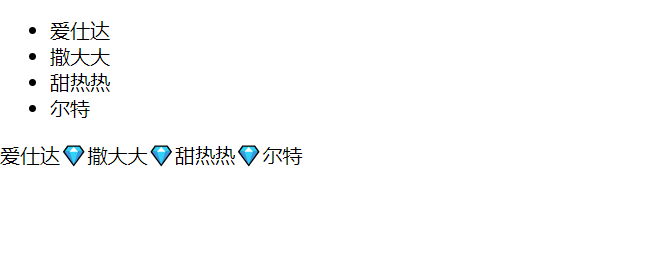
m端事件
// M端事件
// 移动端也有自己独特的地方。比如触屏事件 touch(也称触摸事件),Android 和 IOS 都有。
// 触屏事件 touch(也称触摸事件),Android 和 IOS 都有。
// touch 对象代表一个触摸点。触摸点可能是一根手指,也可能是一根触摸笔。触屏事件可响应用户手指(或触控笔
// )对屏幕或者触控板操作。
// 常见的触屏事件如下:
// touchstart
// 手指触摸到一个DOM元素时触发
// touchmove
// 手指在一个DOM元素上滑动时触发
// touchend
// 手指从一个DOM元素上移开时触发
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.box{
width: 100px;
height: 100px;
background-color: #000;
}
</style>
</head>
<body>
<div class="box"></div>
<script>
const box=document.querySelector('.box')
box.addEventListener('touchstart',function(){
console.log('开始摸');
})
box.addEventListener('touchmove',function(){
console.log('到处摸');
})
box.addEventListener('touchend',function(){
console.log('不摸了');
})
</script>
</body>
</html>
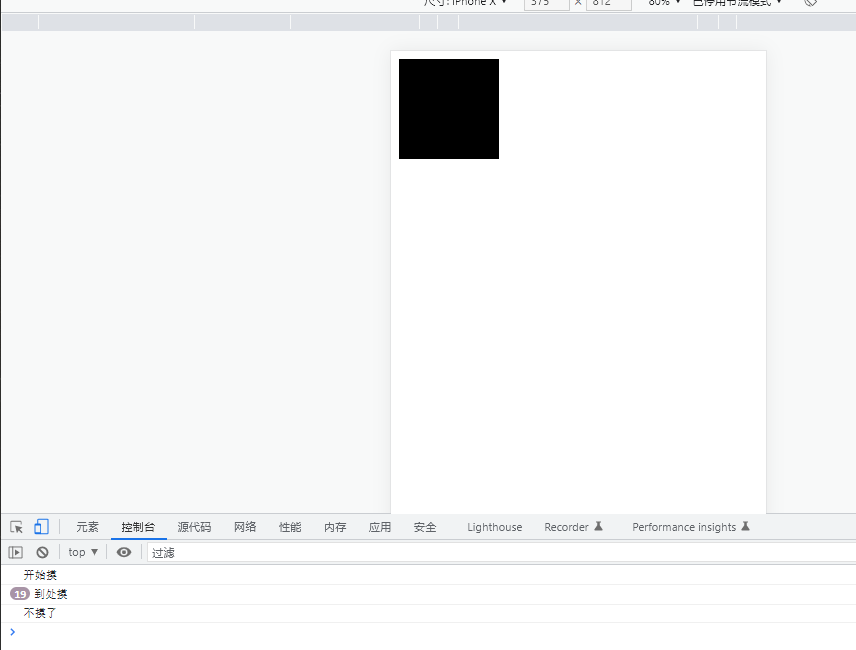
重绘和回流
// 🏆浏览器如何渲染
// 解析(Parser)HTML,生成DOM树(DOM Tree)
// 同时解析(Parser) CSS,生成样式规则 (Style Rules)
// 根据DOM树和样式规则,生成渲染树(Render Tree)
// 进行布局 Layout(回流/重排):根据生成的渲染树,得到节点的几何信息(位置,大小)
// 进行绘制 Painting(重绘): 根据计算和获取的信息进行整个页面的绘制
/ Display: 展示在页面上
// 🏆回流/重排
// 当 Render Tree 中部分或者全部元素的尺寸、结构、布局等发生改变时,浏览器就会重新渲染部分或全部文档的过程称为 回流。
// 🏆重绘
// 由于节点(元素)的样式的改变并不影响它在文档流中的位置和文档布局时(比如:color、background-color、 outline等), 称为重绘。
// 重绘不一定引起回流,而回流一定会引起重绘