1.编写一个程序,读取键盘输入,直到遇到@符号为止,并回显 输入(数字除外),同时将大写字符转换为小写,将小写字符转换为大 写(别忘了cctype函数系列)。
#include<iostream>
#include<cctype>
using namespace std;
int main() {
char ch;
while ((ch = cin.get()) != '@') {
if (isupper(ch)) {//判断是否是大写
ch = tolower(ch);//转换成小写
}
else if (islower(ch)) {//判断是否小写
ch = toupper(ch);//转换成大写
}
else if (isdigit(ch)) {//判断是否为数字
continue;//跳过
}
cout << ch;
}
return 0;
}
2.编写一个程序,最多将10个donation值读入到一个double数组中 (如果您愿意,也可使用模板类array)。程序遇到非数字输入时将结束 输入,并报告这些数字的平均值以及数组中有多少个数字大于平均值。
#include<iostream>
#include<array>
using namespace std;
int main() {
array<double, 10> donation;
double sum = 0, ave = 0, count = 0;
double above_ave = 0;
int i;
for (i = 0; i < 10; i++) {
cout << "Enter donation " << i + 1 << ":";
cin >> donation[i];
if (!cin.fail()) {
sum += donation[i];
}
else {
break;
}
count++;
}
//输出为0时
if (i == 0) {
cout << "No valid donation enterd." << endl;
return 0;
}
ave = sum / count;//求平均值
for (int j = 0; j < count; j++) {
if (donation[j] > ave)
above_ave++;
}
cout << "the Average: " << ave << " and " << above_ave << " number of donation above average";
return 0;
}
3.编写一个菜单驱动程序的雏形。该程序显示一个提供4个选项的 菜单——每个选项用一个字母标记。如果用户使用有效选项之外的字母 进行响应,程序将提示用户输入一个有效的字母,直到用户这样做为 止。然后,该程序使用一条switch语句,根据用户的选择执行一个简单 操作。该程序的运行情况如下:
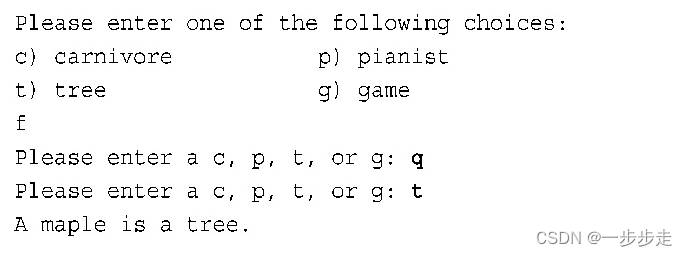
#include<iostream>
using namespace std;
void showmenu();
int main() {
showmenu();
char ch;
while (true) {
cin >> ch;
switch (ch)
{
case 'c':
cout << "Tiger is carnivore.";
break;
case 'p':
cout << "John is a pianist.";
break;
case 't':
cout << "A maple is a tree.";
break;
case 'g':
cout << "Let's play a game!";
break;
default:
cout << "Please enter c,p,t,or g:";
continue;
}
break;
}
}
void showmenu() {
cout << "Please enter one of the following choices:\n";
cout << "c) carnivore(肉食动物)";
cout.width(30);/