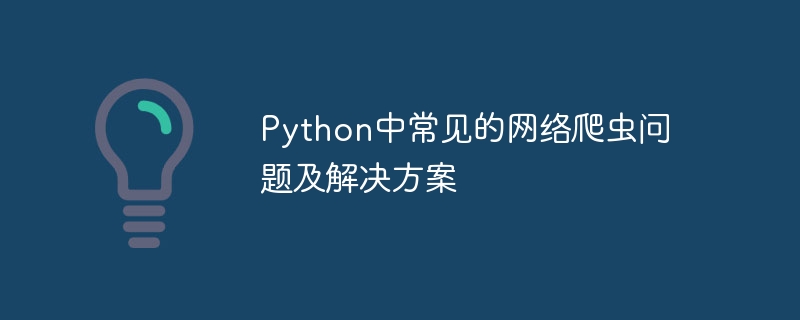
Python中常见的网络爬虫问题及解决方案
概述:
随着互联网的发展,网络爬虫已经成为数据采集和信息分析的重要工具。而Python作为一种简单易用且功能强大的编程语言,被广泛应用于网络爬虫的开发。然而,在实际开发过程中,我们常会遇到一些问题。本文将介绍Python中常见的网络爬虫问题,并提供相应的解决方案,同时附上代码示例。
一、反爬虫策略
反爬虫是指网站为了保护自身利益,采取一系列措施限制爬虫对网站的访问。常见的反爬虫策略包括IP封禁、验证码、登录限制等。以下是一些解决方案:
- 使用代理IP
反爬虫常通过IP地址进行识别和封禁,因此我们可以通过代理服务器获取不同的IP地址来规避反爬虫策略。下面是一个使用代理IP的示例代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
import requests def get_html(url): proxy = { 'http' : 'http://username:password@proxy_ip:proxy_port' , 'https' : 'https://username:password@proxy_ip:proxy_port' } headers = { 'User-Agent' : 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/71.0.3578.98 Safari/537.36' } try : response = requests.get(url, proxies = proxy, headers = headers) if response.status_code = = 200 : return response.text else : return None except requests.exceptions.RequestException as e: return None url = 'http://example.com' html = get_html(url)
|
- 使用随机User-Agent头
反爬虫可能通过检测User-Agent头来识别爬虫访问。我们可以使用随机的User-Agent头来规避该策略。下面是一个使用随机User-Agent头的示例代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
import requests import random def get_html(url): user_agents = [ 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/71.0.3578.98 Safari/537.36' , 'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_14_3) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/71.0.3578.98 Safari/537.36' , 'Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/71.0.3578.98 Safari/537.36' ] headers = { 'User-Agent' : random.choice(user_agents) } try : response = requests.get(url, headers = headers) if response.status_code = = 200 : return response.text else : return None except requests.exceptions.RequestException as e: return None url = 'http://example.com' html = get_html(url)
|
二、页面解析
在爬取数据时,我们常需要对页面进行解析,提取所需的信息。以下是一些常见的页面解析问题及相应的解决方案:
- 静态页面解析
对于静态页面,我们可以使用Python中的一些库,如BeautifulSoup、XPath等,来进行解析。下面是一个使用BeautifulSoup进行解析的示例代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
import requests from bs4 import BeautifulSoup def get_html(url): headers = { 'User-Agent' : 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/71.0.3578.98 Safari/537.36' } try : response = requests.get(url, headers = headers) if response.status_code = = 200 : return response.text else : return None except requests.exceptions.RequestException as e: return None def get_info(html): soup = BeautifulSoup(html, 'html.parser' ) title = soup.title.text return title url = 'http://example.com' html = get_html(url) info = get_info(html)
|
- 动态页面解析
针对使用JavaScript渲染的动态页面,我们可以使用Selenium库来模拟浏览器行为,获取渲染后的页面。下面是一个使用Selenium进行动态页面解析的示例代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
from selenium import webdriver def get_html(url): driver = webdriver.Chrome( 'path/to/chromedriver' ) driver.get(url) html = driver.page_source return html def get_info(html): # 解析获取所需信息 pass url = 'http://example.com' html = get_html(url) info = get_info(html)
|
以上是Python中常见的网络爬虫问题及解决方案的概述。在实际开发过程中,根据不同的场景,可能会遇到更多的问题。希望本文能为读者在网络爬虫开发中提供一些参考和帮助。