动态内存分配与运算符重载
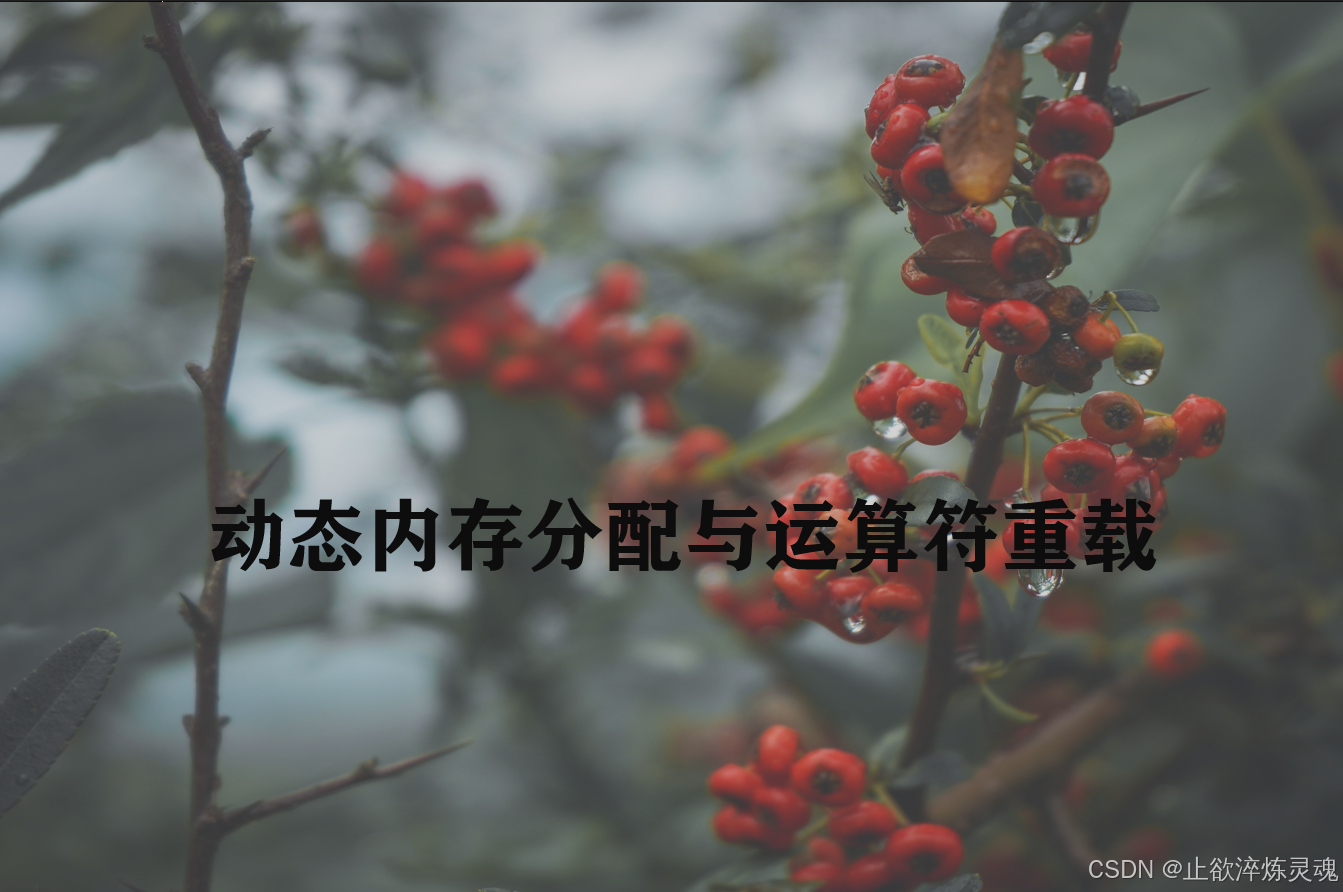
一、动态内存分配
(一)内存的分类
在C/C++中,内存主要分为五个部分,其中我们之前C语言学习过程中的动态内存分配读取的堆区的内存。
- 栈:非静态局部变量/函数参数/返回值等等,栈是向下增长的。
- 内存映射段:是高效的I/O映射方式,用于装载一个共享的动态内存库。用户可使用系统接口创建共享共享内存,做进程间通信。
- 堆:用于程序运行时动态内存分配,堆是向上增长的。
- 数据段:存储全局数据和静态数据
- 代码段:可执行代码、只读常量
(二)动态内存分配函数
(1)new 和delete 的使用
在动态内存分配是,通过控制台可以发现,堆区是向上增长的。同时也发现了new / delete
和 malloc / free
这组函数的区别,前者会申请后完成构造,释放时自动调用析构,而后者只做空间的申请。
class A
{
public:
A(int a = 0)
: _a(a)
{
cout << "A():" << this << endl;
}
~A()
{
cout << "~A():" << this << endl;
}
private:
int _a;
};
int main() {
cout << "内置类型:" << endl;
int* p1 = new int;
int* p2 = new int(1);
int* p3 = new int[3];
delete p1;
delete p2;
delete[] p3;
cout << "自定义类型:" << endl;
A* p4 = new A;
A* p5 = new A(1);
A* p6 = new A[3];
delete p4;
delete p5;
delete[] p6;
return 0;
}
(1)new 的原理
通过汇编代码,可以清楚的发现,new 调用了 operator new 函数和构造函数
(2)delete 的原理
和new 一样,先调用了析构函数,后调用了 operater delete。
2、 operator new与operator delete
需要区分的是,new 和 delete是用户进行动态内存申请和释放的操作符,operator new
和operator delete
是系统提供的全局函数,不是函数重载。new在底层调用operator new来申请空间,delete在底层通过operator delete来释放空间。
(1)operator new 的原理
通过下面代码,我们可以发现,operate new也是通过 malloc 来申请空间,相比于 malloc,不再通过返回值是否为空来判断申请的成功与否,而是抛异常的方式。
而在参数上面二者一致。
void* __CRTDECL operator new(size_t size) _THROW1(_STD bad_alloc)
{
// try to allocate size bytes
void* p;
while ((p = malloc(size)) == 0)
if (_callnewh(size) == 0)
{
// report no memory
// 如果申请内存失败了,这里会抛出bad_alloc 类型异常
static const std::bad_alloc nomem;
_RAISE(nomem);
}
return (p);
}
(2)operator delete 的原理
不难看出,delete 的底层也是采用free 实现,free 本质是一个宏。
void operator delete(void* pUserData)
{
_CrtMemBlockHeader* pHead;
RTCCALLBACK(_RTC_Free_hook, (pUserData, 0));
if (pUserData == NULL)
return;
_mlock(_HEAP_LOCK);
__TRY
pHead = pHdr(pUserData);
_ASSERTE(_BLOCK_TYPE_IS_VALID(pHead->nBlockUse));
_free_dbg(pUserData, pHead->nBlockUse);
__FINALLY
_munlock(_HEAP_LOCK);
__END_TRY_FINALLY
return;
}
#define free(p) _free_dbg(p, _NORMAL_BLOCK)
(三)定位new
如果我们想要将申请空间和构造函数、释放空间和析构函数分开调用,又该怎么办呢。我们知道构造函数不可以显示调用,此时可以用到定位new。
new (place_address) type
或者new (place_address) type(initializer-list)
其中,place_address必须是一个指针,initializer-list是类型的初始化列表
A* object = (A*)malloc(sizeof(A));
new(object)A(1);
object->~A();
free(object);
A* object2 = (A*)operator new(sizeof(A));
new(object2)A;
object2->~A();
free(object2);
在上面的代码中,采用malloc
和operater new
的唯一区别就是前者可以通过检查返回值查看是否开辟成功,而后者通过抛异常的方式检测。
(四) malloc/free和new/delete的区别
- malloc和free是函数,new和delete是操作符
- malloc申请的空间不会初始化,new可以初始化
- malloc申请空间时,需要手动计算空间大小并传递,new只需在其后跟上空间的类型,多个对象在[]中指定对象个数
- malloc的返回值为void*, 在使用时必须强转,new不需要
- malloc申请空间失败时,返回的是NULL,因此使用时必须判空,new不需要,但是new需要捕获异常
- 申请自定义类型对象时,malloc/free只会开辟空间,不会调用构造函数与析构函数,而new和delete会调用构造函数与析构函数。
二、操作符重载
我们通过实现一个日期类来说明这部分知识。由于运算符重载并不复杂,挑选有特点的部分讲解。
运算符重载要点
1、可以利用运算符之间的联系来重载其它运算符。
2、对于不改变this 指针的运算符,可以考虑加上const
否则一些const对象在调用时可能会造成权限的放大,导致错误
(一)输入输出重载
输入输出流重载中,只能写成全局函数。因为成员函数中存在隐藏的this 指针,而函数重载规定参数列表中的第一个参数是在运算符右边,如果写成成员函数就要反过来,老别扭了。
ostream& operator<<(ostream& out, const Date& d)
{
out << d._year << "年" << d._month << "月" << d._day << "日" << endl;
return out;
}
istream& operator>>(istream & in, Date & d)
{
while (1) {
cout << "请输入年、月、日" << endl;
in >> d._year >> d._month >> d._day;
if (!d.check_date()) {
cout << "日期不合法,请重新输入!" << endl;
continue;
}
break;
}
return in;
}
(二)其它操作符重载
class Date {
friend ostream& operator<<(ostream& out, const Date& data);
friend istream& operator>>(istream& in, Date& d);
public:
Date(int year = 1, int month = 1, int day = 1);
bool check_date() const;
bool isRunNian() const;
int getMonthDay(int month) const;
void print_date() const;
//比较运算符重载
bool operator<(const Date& d) const;
bool operator<=(const Date& d) const;
bool operator>(const Date& d) const;
bool operator>=(const Date& d) const;
bool operator==(const Date& d) const;
bool operator!=(const Date& d) const;
//加减运算符重载
Date& operator+=(int day);
Date operator+(int day) const;
Date& operator-=(int day);
Date operator-(int day) const;
int operator-(const Date& d) const;
Date& operator++();
Date operator++(int);
Date& operator--();
Date operator--(int);
private:
int _year;
int _month;
int _day;
static int _month_day[13];
};
int Date::_month_day[13] = {0,31,28,31,30,31,30,31,31,30,31,30,31 };
Date::Date(int year, int month, int day)
:_year(year)
, _month(month)
, _day(day)
{
if (!check_date()) {
cout << "日期不合法!" << endl;
}
}
bool Date::check_date() const
{
if (_month > 12 || _month < 1)return false;
else if (_day > getMonthDay(_month) || _day < 1) return false;
else return true;
}
bool Date::isRunNian()const
{
if ((_year % 4 == 0 && _year % 100 != 0) || _year % 400 == 0)
return true;
else return false;
}
int Date::getMonthDay(int month) const
{
if (month == 2) {
if (isRunNian()) return 29;
}
return _month_day[month];
}
void Date::print_date() const
{
cout << _year << '-' << _month << '-' << _day << endl;
}
bool Date::operator<(const Date& d) const
{
if (_year != d._year)return _year < d._year;
else if (_month != d._month) return _month < d._month;
else return _day < d._day;
}
bool Date::operator<=(const Date& d) const
{
return (*this < d || *this == d);
}
bool Date::operator>(const Date& d) const
{
return !(*this <= d);
}
bool Date::operator>=(const Date& d) const
{
return !(*this < d);
}
bool Date::operator==(const Date& d) const
{
return _year == d._year && _month == d._month && _day == d._day;
}
bool Date::operator!=(const Date& d) const
{
return !(*this == d);
}
Date& Date::operator+=(int day)
{
_day += day;
while (_day > getMonthDay(_month)) {
_day -= getMonthDay(_month);
if (++_month == 13) {
_month = 1;
_year += 1;
}
}
return *this;
}
Date Date::operator+(int day) const
{
Date object(*this);
object += day;
return object;
}
Date& Date::operator-=(int day)
{
_day -= day;
while (_day < 1) {
if (--_month == 0) {
_month = 12;
_year -= 1;
}
_day += getMonthDay(_month);
}
return *this;
}
Date Date::operator-(int day) const
{
Date object(*this);
object -= day;
return object;
}
int Date::operator-(const Date& d) const
{
Date max = *this;
Date min = d;
int flag = 1;
if (max < min) {
max = d;
min = *this;
flag = -1;
}
int n = 0;
while (min != max) {
min += 1;
n++;
}
return n;
}
Date& Date::operator++()
{
return (*this += 1);
}
Date Date::operator++(int)
{
Date temp(*this);
*this += 1;
return temp;
}
Date& Date::operator--()
{
return (*this -= 1);
}
Date Date::operator--(int)
{
Date temp(*this);
*this -= 1;
return temp;
}
void test01()
{
Date object1(2024, 2, 29);
Date object2(2024, 2, 18);
Date object3(2024, 2, 18);
if (object1 > object2) cout << "object1 < object2" << endl;
if (object3 == object2) cout << "object3 == object2" << endl;
}
ostream& operator<<(ostream& out, const Date& d)
{
out << d._year << "年" << d._month << "月" << d._day << "日" << endl;
return out;
}
istream& operator>>(istream & in, Date & d)
{
while (1) {
cout << "请输入年、月、日" << endl;
in >> d._year >> d._month >> d._day;
if (!d.check_date()) {
cout << "日期不合法,请重新输入!" << endl;
continue;
}
break;
}
return in;
}
void test02()
{
Date object1(1940, 10, 5);
Date object2(2024, 8, 27);
Date object3(2024, 9, 19);
Date object4(2004, 7, 19);
object4 += 7367;
object4.print_date();
object3 -= 7367;
object3.print_date();
cout << object3 - object4 << endl;
cout << object2 - object1 << endl;
object1++;
object1.print_date();
object1--;
object1.print_date();
结束语
小伙伴们,文章就先告一段落了,感谢各位长期以来的支持,博主就先撤啦!