P2240 【深基12.例1】部分背包问题
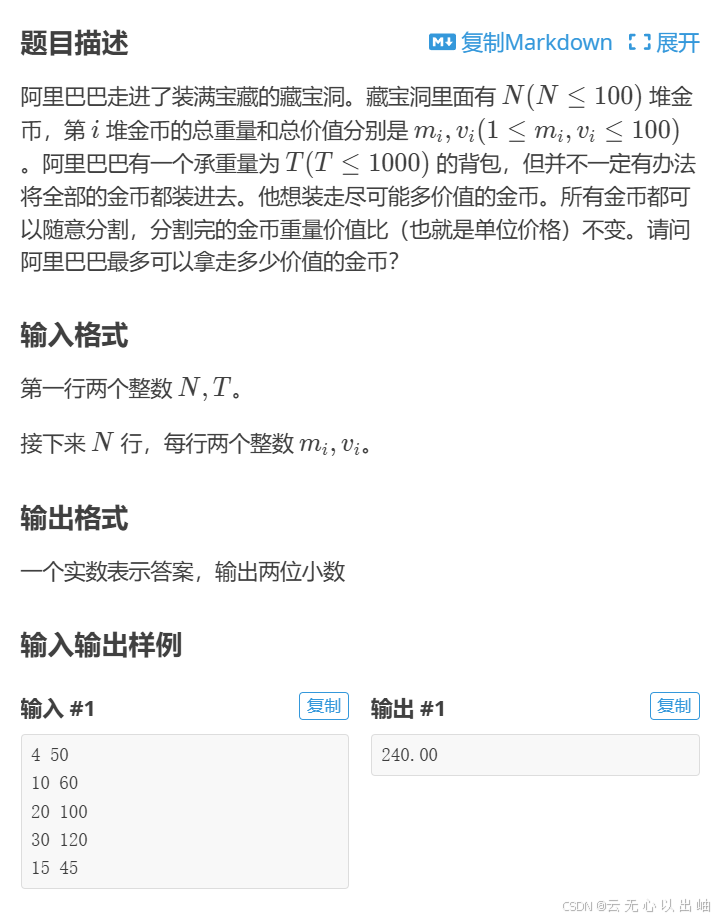
答案
#include <bits/stdc++.h>
using namespace std;
struct Node{//金币结构体
int w,v;
}a[110];
int read(){
int x=0,f=1;
char c=getchar();
while(c<'0'||c>'9'){
if(c=='-')f=-1;
c=getchar();
}
while(c>='0'&&c<='9'){
x=x*10+c-'0';
c=getchar();
}
return x*f;
}
bool cmp(Node aa,Node bb){
return aa.v*bb.w>aa.w*bb.v;
//按性价比从高到低排序,为防止精度问题直接交叉相乘
}
int main(){
int n=read(),m=read();
double ans=0;
for(int i=1;i<=n;i++){
a[i].w=read(),a[i].v=read();
}
sort(a+1,a+n+1,cmp);
for(int i=1;i<=n;i++){
if(a[i].w<=m){
ans+=a[i].v;
m-=a[i].w;
}
else{
ans+=a[i].v*m*1.0/(a[i].w*1.0);
//*1.0强转double
break;
}
}
printf("%.2lf",ans);
return 0;
}
P1223 排队接水
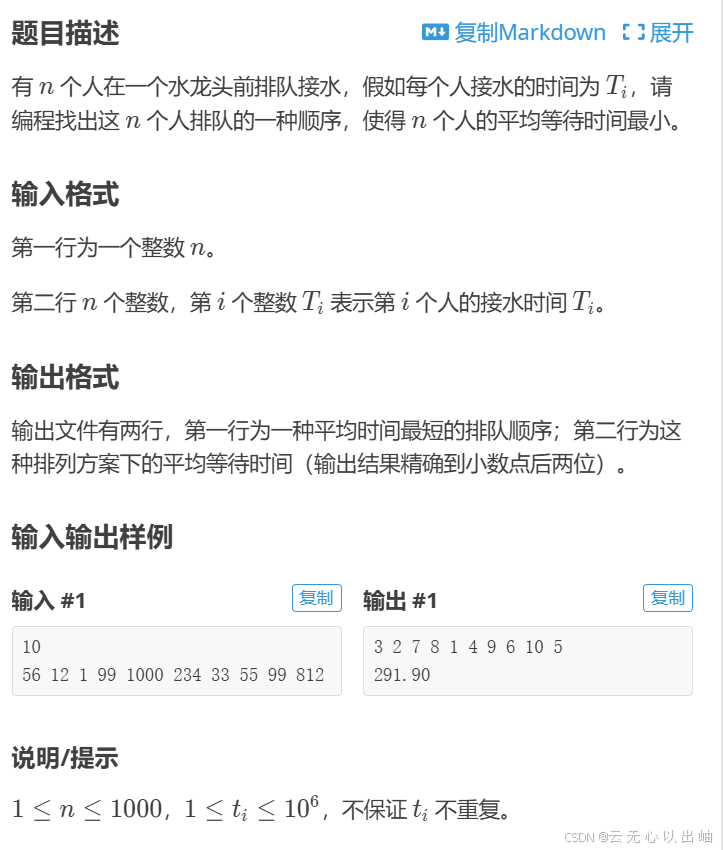
答案
#include <bits/stdc++.h>
using namespace std;
struct Node {
int n, t;
} a[1010];
// 按照接水时间从小到大排序
bool cmp(Node aa, Node bb) {
return aa.t < bb.t;
}
// 读入函数
int read() {
int x = 0, f = 1;
char c = getchar();
while (c < '0' || c > '9') {
if (c == '-') {
f = -1;
}
c = getchar();
}
while (c >= '0' && c <= '9') {
x = x * 10 + c - '0';
c = getchar();
}
return x * f;
}
int main() {
int N = read();
double ans = 0;
// 读取每个人的接水时间
for (int i = 1; i <= N; i++) {
a[i].t = read();
a[i].n = i;
}
// 对接水时间从小到大排序
sort(a + 1, a + N + 1, cmp);
// 输出排队顺序
for (int i = 1; i <= N; i++) {
printf("%d ", a[i].n);
if (i < N) { // 前面的所有人需要等待
ans += a[i].t * (N - i);
}
}
printf("\n");
// 计算平均等待时间并输出
printf("%.2lf\n", ans / N);
return 0;
}
P1803 凌乱的yyy / 线段覆盖
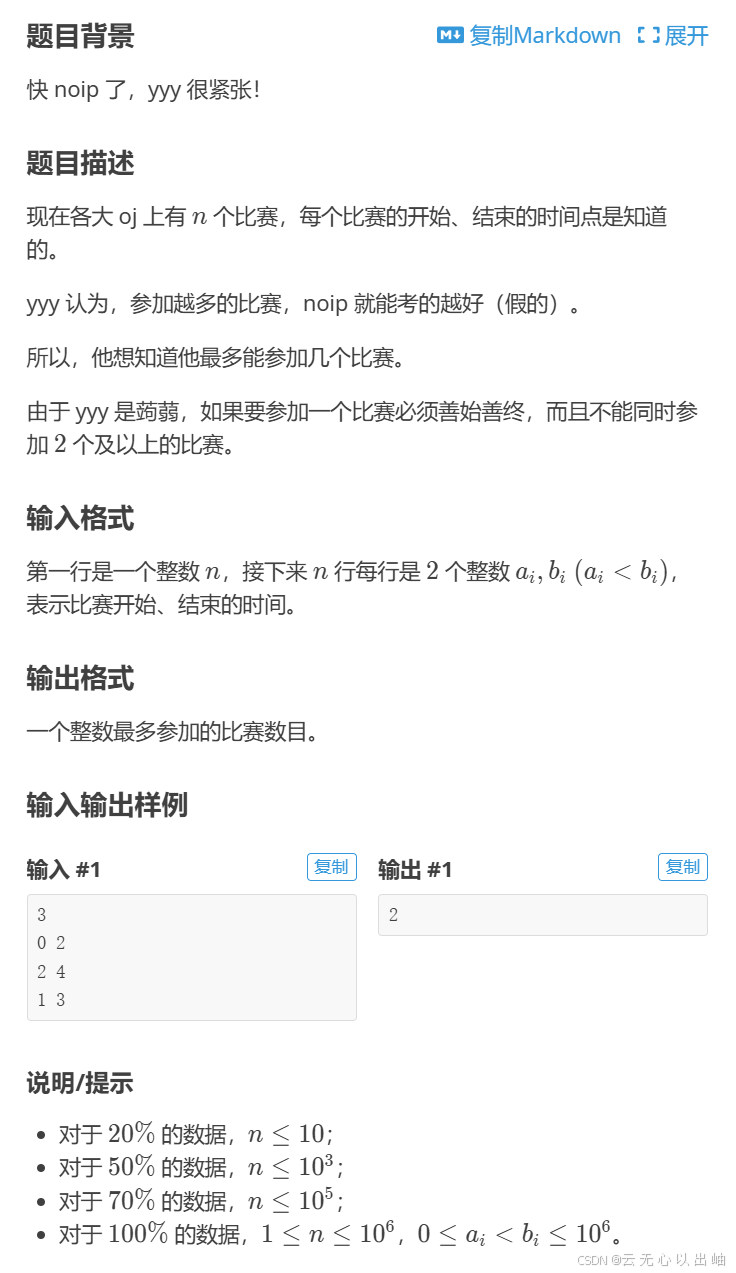
答案
#include <iostream>
#include <cstdio>
#include <algorithm>
using namespace std;
int N;
struct act{
int start;
int end;
}a[1000010];
bool cmp(act a,act b){
return a.end<b.end;
}
int greedy(){
int num=1,i=0;
for(int j=1;j<=N;j++){
if(a[j].start>=a[i].end){
i=j;
num++;
}
}
return num;
}
int main(){
scanf("%d",&N);
for(int i=0;i<N;i++){
scanf("%d%d",&a[i].start,&a[i].end);
}
sort(a,a+N,cmp);
int ans=greedy();
printf("%d\n",ans);
return 0;
}