目录
The After Route Predicate Factory
The Before Route Predicate Factory
The Between Route Predicate Factory
The Header Route Predicate Factory
The Host Route Predicate Factory
The Method Route Predicate Factory
The Path Route Predicate Factory
The RemoteAddr Route Predicate Factory
Route Predicate Factories
Predicate
Predicate是Java 8提供的⼀个函数式编程接⼝, 它接收⼀个参数并返回⼀个布尔值, ⽤于条件过滤, 请求参数的校验.
@FunctionalInterface
public interface Predicate<T> {
/**
* Evaluates this predicate on the given argument.
*
* @param t the input argument
* @return {@code true} if the input argument matches the predicate,
* otherwise {@code false}
*/
boolean test(T t);
}
实现Predicate接口
package predicate;
import java.util.function.Predicate;
/**
* 判断字符串是否为空
* 空 -true
* 非空 -false
*/
public class StringPredicate implements Predicate<String> {
@Override
public boolean test(String s) {
return s == null || s.isEmpty();
}
}
测试运行
package predicate;
import org.junit.Test;
import java.util.function.Predicate;
public class PredicateTest {
@Test
public void test(){
Predicate<String> predicate = new StringPredicate();
System.out.println(predicate.test(""));
System.out.println(predicate.test("aa"));
}
}
运行结果:
Predicate的其它实现方法
匿名内部类
package predicate;
import org.junit.Test;
import java.util.function.Predicate;
public class PredicateTest {
@Test
public void test2(){
Predicate<String> predicate = new Predicate<String>() {
@Override
public boolean test(String s) {
return s == null || s.isEmpty();
}
};
System.out.println(predicate.test(""));
System.out.println(predicate.test("aa"));
}
}
运行结果:
lambda表达式
package predicate;
import org.junit.Test;
import java.util.function.Predicate;
public class PredicateTest {
@Test
public void test3(){
Predicate<String> predicate = s -> s == null || s.isEmpty();
System.out.println(predicate.test(""));
System.out.println(predicate.test("aa"));
}
}
运行结果:
Predicate的其它方法
1.isEqual(Object targetRef) :比较两个对象是否相等,参数可以为Null
2.and(Predicate other): 短路与操作,返回⼀个组成Predicate
3.or(Predicate other) :短路或操作,返回⼀个组成Predicate
4.test(T t) :传⼊⼀个Predicate参数,⽤来做判断
5.negate() : 返回表⽰此Predicate逻辑否定的Predicate
源码详解
当前类的test方法&&other类的test方法
对当前类的test方法进行取非
当前类的test方法 || other类的test方法
判断两个对象是否相等
代码示例
代码示例1
package predicate;
import org.junit.Test;
import java.util.function.Predicate;
public class PredicateTest {
@Test
public void test4(){
Predicate<String> predicate = s -> s == null || s.isEmpty();
System.out.println(predicate.negate().test(""));
System.out.println(predicate.negate().test("aa"));
}
}
运行结果:
代码示例2
package predicate;
import org.junit.Test;
import java.util.function.Predicate;
public class PredicateTest {
@Test
public void test5(){
Predicate<String> predicate = s-> "aa".equals(s);
Predicate<String> predicate2 = s-> "bb".equals(s);
System.out.println(predicate.or(predicate2).test(""));
System.out.println(predicate.or(predicate2).test("aa"));
}
}
运行结果:
代码示例3
package predicate;
import org.junit.Test;
import java.util.function.Predicate;
public class PredicateTest {
@Test
public void test6(){
Predicate<String> predicate = s-> s!=null && !s.isEmpty();
Predicate<String> predicate2 = s-> s!=null && s.chars().allMatch(Character::isDigit);
System.out.println(predicate.and(predicate2).test("aa"));
System.out.println(predicate.and(predicate2).test("123"));
}
}
运行结果:
Route Predicate Factories
Route Predicate Factories (路由断⾔⼯⼚, 也称为路由谓词⼯⼚, 此处谓词表⽰⼀个函数), 在Spring Cloud Gateway中, Predicate提供了路由规则的匹配机制.
我们在配置⽂件中写的断⾔规则只是字符串, 这些字符串会被Route Predicate Factory读取并处理, 转变为路由判断的条件。
这个规则是由org.springframework.cloud.gateway.handler.predicate.PathRoutePredicateF
actory 来实现的.
Spring Cloud Gateway 默认提供了很多Route Predicate Factory, 这些Predicate会分别匹配HTTP请求的不同属性, 并且多个Predicate可以通过and逻辑进⾏组合.
详细介绍
The After Route Predicate Factory
The
After
route predicate factory takes one parameter, adatetime
(which is a javaZonedDateTime
). This predicate matches requests that happen after the specified datetime. The following example configures an after route predicate:
这个⼯⼚需要⼀个⽇期时间(Java的 ZonedDateTime对象), 匹配指定⽇期之后的请求
The Before Route Predicate Factory
The Before
route predicate factory takes one parameter, a datetime
(which is a java ZonedDateTime
). This predicate matches requests that happen before the specified datetime
. The following example configures a before route predicate:
匹配指定⽇期之前的请求
The Between Route Predicate Factory
The
Between
route predicate factory takes two parameters,datetime1
anddatetime2
which are javaZonedDateTime
objects. This predicate matches requests that happen afterdatetime1
and beforedatetime2
. Thedatetime2
parameter must be afterdatetime1
. The following example configures a between route predicate:
匹配两个指定时间之间的请求datetime2 的参数必须在datetime1 之后
The Cookie Route Predicate Factory
The
Cookie
route predicate factory takes two parameters, the cookiename
and aregexp
(which is a Java regular expression). This predicate matches cookies that have the given name and whose values match the regular expression. The following example configures a cookie route predicate factory:
请求中包含指定Cookie, 且该Cookie值符合指定的正则表达式
The Header Route Predicate Factory
The
Header
route predicate factory takes two parameters, theheader
and aregexp
(which is a Java regular expression). This predicate matches with a header that has the given name whose value matches the regular expression. The following example configures a header route predicate:
请求中包含指定Header, 且该Header值符合指定的正则表达式
The Host Route Predicate Factory
The
Host
route predicate factory takes one parameter: a list of host namepatterns
. The pattern is an Ant-style pattern with.
as the separator. This predicates matches theHost
header that matches the pattern. The following example configures a host route predicate:
请求必须是访问某个host(根据请求中的Host字段进⾏匹配)
The Method Route Predicate Factory
The
Method
Route Predicate Factory takes amethods
argument which is one or more parameters: the HTTP methods to match. The following example configures a method route predicate:
匹配指定的请求⽅式
The Path Route Predicate Factory
The
Path
Route Predicate Factory takes two parameters: a list of SpringPathMatcher
patterns
and an optional flag calledmatchTrailingSlash
(defaults totrue
). The following example configures a path route predicate:
匹配指定规则的路径
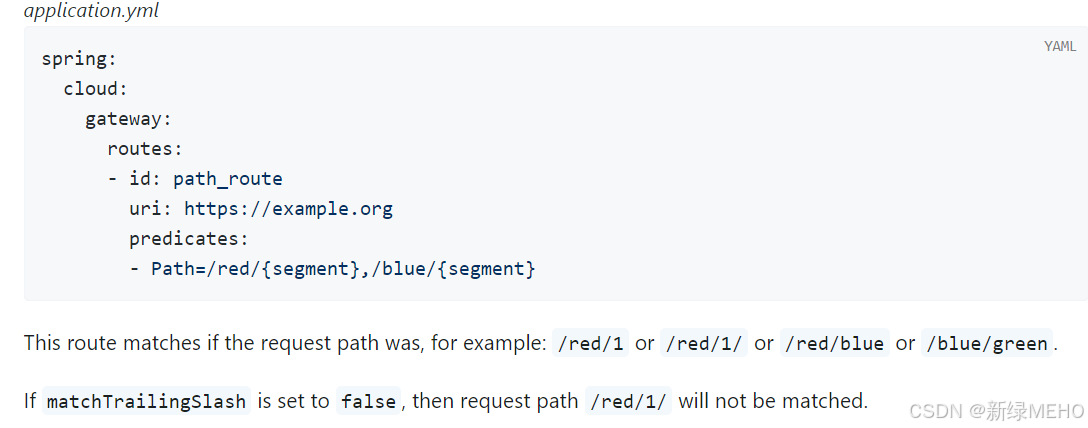
The RemoteAddr Route Predicate Factory
The
RemoteAddr
route predicate factory takes a list (min size 1) ofsources
, which are CIDR-notation (IPv4 or IPv6) strings, such as192.168.0.1/16
(where192.168.0.1
is an IP address and16
is a subnet mask). The following example configures a RemoteAddr route predicate:
请求者的IP必须为指定范围
欢迎大家来访问我的博客主页-----》博客链接