以下是关于 Spring Boot 微服务解决方案的对比,并以 Spring Cloud Alibaba 为例,详细说明其核心组件的使用方式、配置及代码示例:
关于 Spring Cloud Alibaba
致力于提供微服务开发的一站式解决方案!
https://sca.aliyun.com/?spm=7145af80.434205f.0.0.74716242oEhMef
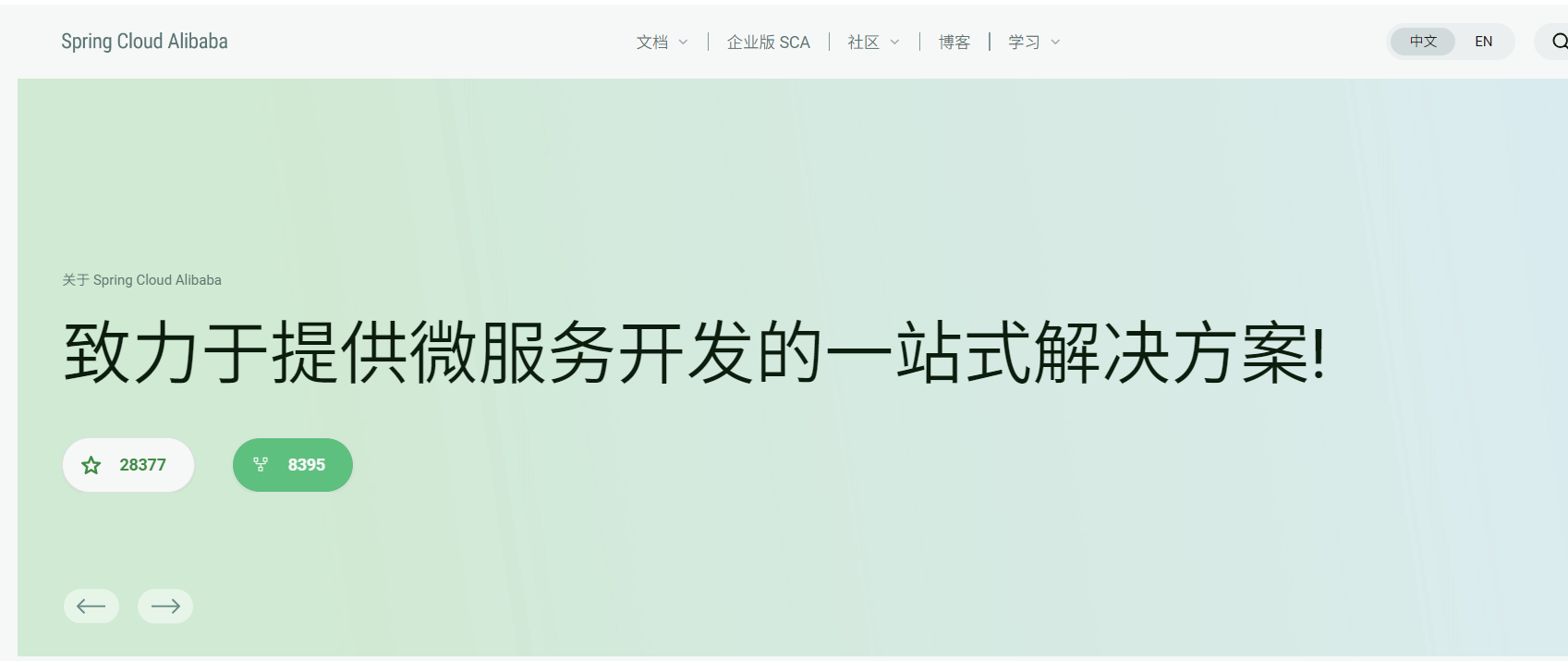
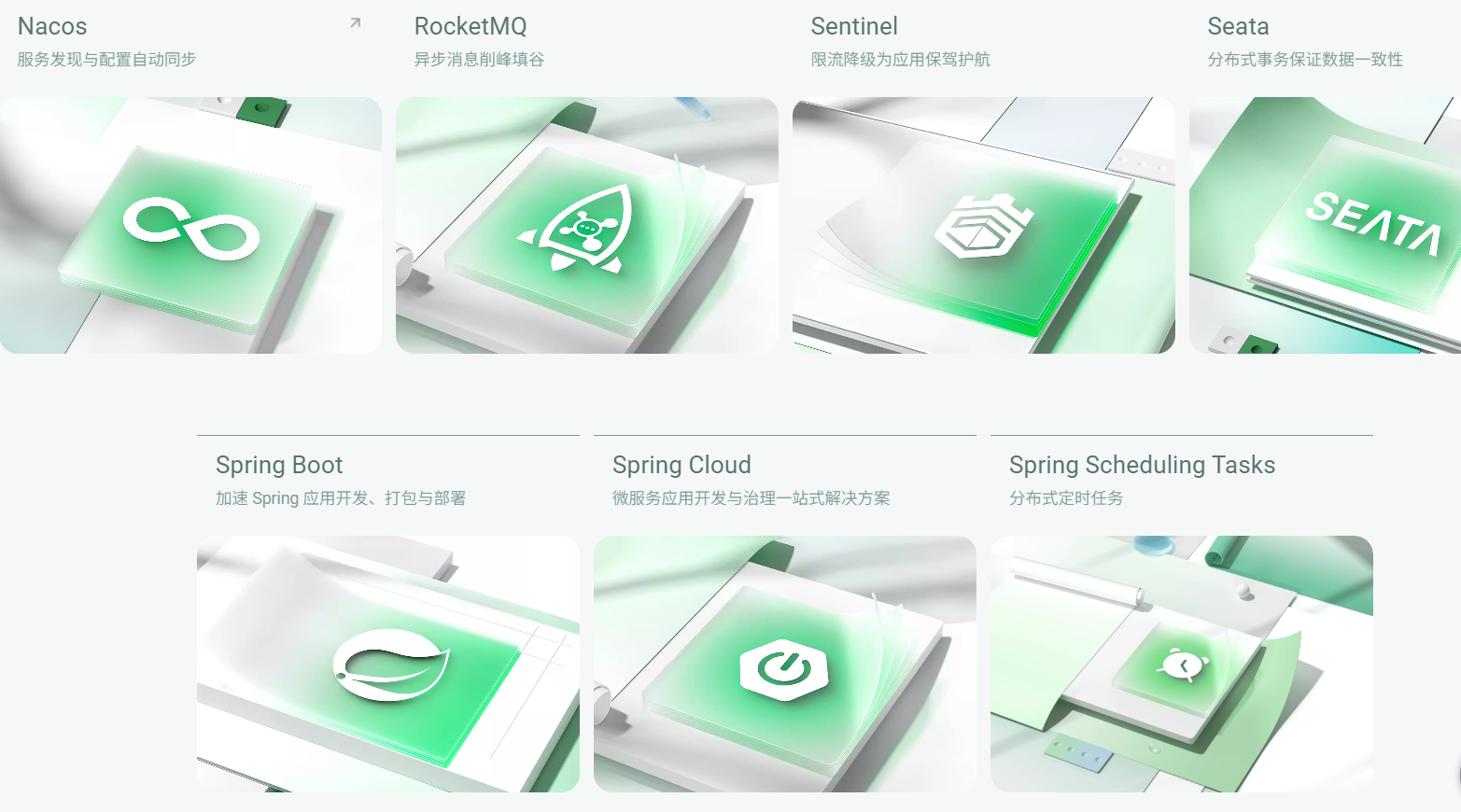
1. 微服务解决方案对比
方案 |
核心组件 |
特点 |
适用场景 |
Spring Cloud Netflix |
Eureka(注册中心)、Hystrix(熔断)、Feign(RPC)、Zuul/Gateway(网关) |
成熟稳定,社区广泛,但部分组件(如 Hystrix)已停止维护 |
传统微服务架构,需兼容旧系统 |
Spring Cloud Alibaba |
Nacos(注册/配置中心)、Sentinel(流控降级)、Dubbo/Feign(RPC)、Gateway(网关) |
阿里巴巴生态,轻量高效,支持云原生,组件活跃维护 |
新项目,需要与阿里云/容器化集成 |
Spring Cloud Consul |
Consul(注册/配置中心)、Resilience4j(熔断)、Spring Cloud Gateway(网关) |
基于 Consul 的强一致性,适合分布式系统 |
需要强一致性分布式环境 |
Istio/Servie Mesh |
Pilot(控制平面)、Envoy(数据平面)、Mixer(策略与遥测) |
服务网格方案,无侵入式治理,支持多语言 |
云原生环境,多语言微服务架构 |
2. Spring Cloud Alibaba 微服务开发详解
2.1 核心组件
组件 |
功能 |
依赖 |
Nacos |
服务注册与发现、动态配置中心 |
spring-cloud-starter-alibaba-nacos-discovery , spring-cloud-starter-alibaba-nacos-config |
Sentinel |
流量控制、熔断降级、系统负载保护 |
spring-cloud-starter-alibaba-sentinel |
Dubbo/Spring MVC |
服务间通信(RPC) |
spring-cloud-starter-dubbo 或 spring-boot-starter-web |
Gateway |
API 网关,路由、鉴权、限流 |
spring-cloud-starter-gateway |
Seata |
分布式事务管理 |
seata-spring-boot-starter |
Sleuth+Zipkin |
分布式链路追踪 |
spring-cloud-starter-sleuth , spring-cloud-sleuth-zipkin |
3. 代码示例:Spring Cloud Alibaba 微服务
3.1 项目依赖(pom.xml)
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>2022.0.3</version>
<type>pom</type>
<scope>import</scope>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-alibaba-dependencies</artifactId>
<version>2022.0.0.0</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-config</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-sentinel</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
</dependency>
<dependency>
<groupId>io.seata</groupId>
<artifactId>seata-spring-boot-starter</artifactId>
<version>1.7.1</version>
</dependency>
</dependencies>
3.2 服务提供者(Provider)
3.2.1 配置 application.yml
server:
port: 8080
spring:
application:
name: user-service
cloud:
nacos:
discovery:
server-addr: 127.0.0.1:8848
config:
server-addr: 127.0.0.1:8848
extension-configs:
- file-extension=yaml
group: DEFAULT_GROUP
3.2.2 启动类
@SpringBootApplication
@EnableDiscoveryClient
public class UserServiceApplication {
public static void main(String[] args) {
SpringApplication.run(UserServiceApplication.class, args);
}
}
3.2.3 服务接口
@RestController
@RequestMapping("/users")
public class UserController {
@GetMapping("/{id}")
public User getUser(@PathVariable Long id) {
return new User(id, "John Doe");
}
}
3.3 服务消费者(Consumer)
3.3.1 配置 application.yml
spring:
application:
name: order-service
cloud:
nacos:
discovery:
server-addr: 127.0.0.1:8848
3.3.2 调用服务
@Service
public class OrderService {
@Autowired
private RestTemplate restTemplate;
public Order createOrder(Long userId) {
User user = restTemplate.getForObject(
"http://user-service/users/{userId}", User.class, userId
);
return new Order(user.getName(), "ORDER-123");
}
}
3.4 Nacos 配置中心
3.4.1 配置文件(Nacos 中配置)
user:
service:
timeout: 5000
3.4.2 读取配置
@ConfigurationProperties(prefix = "user.service")
public class UserConfig {
private Integer timeout;
}
3.5 Sentinel 熔断降级
3.5.1 配置限流规则(Nacos 或本地文件)
[
{
"resource": "/users/{id}",
"count": 100,
"grade": 1,
"limitApp": "default",
"strategy": 0,
"controlBehavior": 0,
"statIntervalMs": 1000
}
]
3.5.2 注解限流
@RestController
public class UserController {
@SentinelResource(value = "getUser", blockHandler = "handleBlock")
@GetMapping("/{id}")
public User getUser(@PathVariable Long id) {
}
public User handleBlock(BlockException e) {
return new User(-1L, "服务不可用");
}
}
3.6 API 网关(Spring Cloud Gateway)
3.6.1 配置路由(application.yml)
spring:
cloud:
gateway:
routes:
- id: user_route
uri: lb://user-service
predicates:
- Path=/api/users/**
filters:
- StripPrefix=1
3.6.2 启动类
@SpringBootApplication
@EnableDiscoveryClient
public class GatewayApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayApplication.class, args);
}
}
3.7 分布式事务(Seata)
3.7.1 配置 application.yml
seata:
enabled: true
application-id: ${spring.application.name}
tx-service-group: ${spring.application.name}-group
service:
vgroup-mapping:
${spring.application.name}-group: default
grouplist:
default: 127.0.0.1:8091
3.7.2 事务注解
@Service
public class OrderService {
@GlobalTransactional(name = "createOrder")
public void createOrder() {
userService.createUser();
inventoryService.reduceStock();
}
}
3.8 分布式链路追踪(Sleuth + Zipkin)
3.8.1 配置 application.yml
spring:
zipkin:
base-url: http://zipkin-server:9411
sleuth:
sampler:
probability: 1.0
3.8.2 启动类
@SpringBootApplication
public class TraceApplication {
public static void main(String[] args) {
SpringApplication.run(TraceApplication.class, args);
}
}
4. 组件功能对比表格
组件 |
功能 |
配置方式 |
代码示例 |
Nacos |
服务注册、发现、动态配置 |
application.yml 配置 Nacos 地址 |
@EnableDiscoveryClient , 配置 server-addr |
Sentinel |
流量控制、熔断降级 |
JSON 配置规则或代码动态配置 |
@SentinelResource , 限流规则文件 |
Gateway |
路由、鉴权、限流 |
application.yml 定义路由规则 |
@EnableDiscoveryClient , 路由配置文件 |
Seata |
分布式事务管理 |
配置 seata-config 和事务组 |
@GlobalTransactional , 配置 seata-application.yml |
Sleuth+Zipkin |
链路追踪 |
配置 Zipkin 服务器地址 |
@SpringBootApplication , 配置 spring.zipkin.base-url |
5. 完整项目结构示例
my-microservices/
├── config-center/ # Nacos 配置中心
├── user-service/ # 用户服务(Provider)
├── order-service/ # 订单服务(Consumer)
├── gateway/ # API 网关
├── seata-server/ # 分布式事务服务器
└── zipkin-server/ # 链路追踪服务器
6. 关键配置步骤
6.1 Nacos 服务注册
spring:
cloud:
nacos:
discovery:
server-addr: 127.0.0.1:8848
6.2 Sentinel 熔断配置
@Configuration
public class SentinelConfig {
@Bean
public SentinelGatewayFilterFactory sentinelFilterFactory() {
return new SentinelGatewayFilterFactory();
}
}
6.3 Seata 分布式事务
service {
vgroup_mapping.my_test_tx_group = "default"
default.grouplist = "127.0.0.1:8091"
}
7. 总结表格
组件 |
功能 |
配置方式 |
代码示例 |
Nacos |
服务注册/发现、配置中心 |
application.yml 配置服务器地址 |
@EnableDiscoveryClient , 动态配置刷新 |
Sentinel |
流量控制、熔断降级 |
JSON 配置或代码动态配置 |
@SentinelResource , 限流规则定义 |
Gateway |
API 网关,路由、鉴权 |
application.yml 定义路由规则 |
@EnableDiscoveryClient , 路由配置文件 |
Seata |
分布式事务管理 |
配置 seata-config 和事务组 |
@GlobalTransactional , 事务组配置 |
Sleuth+Zipkin |
分布式链路追踪 |
配置 Zipkin 服务器地址 |
@SpringBootApplication , 链路日志收集 |
8. 注意事项
- Nacos 集群部署:生产环境需部署 Nacos 集群以保证高可用。
- Sentinel 规则管理:建议通过控制台(
nacos-config
)动态管理规则。
- Seata 性能优化:需配置数据库事务隔离级别(如
READ_COMMITTED
)。
- 链路追踪:Zipkin 需独立部署,支持 HTTP 或 Kafka 模式。
通过以上组件的组合,可以构建一个高可用、可扩展的微服务架构。Spring Cloud Alibaba 的组件设计更贴合云原生需求,适合中大型分布式系统。
番外
以下是关于 微服务服务治理 的详细说明,涵盖核心概念、主流框架/解决方案的对比,以及关键功能的总结表格:
1. 服务治理核心概念
服务治理是微服务架构中管理服务间通信、监控、容错、配置等的核心能力。主要功能包括:
- 服务注册与发现:动态管理服务实例。
- 配置中心:集中管理配置。
- 负载均衡:分发请求流量。
- 熔断降级:防止雪崩效应。
- 服务网关:统一入口,路由与鉴权。
- 分布式事务:保证跨服务事务一致性。
- 链路追踪:监控请求链路性能。
2. 主流服务治理框架/解决方案对比
2.1 Spring Cloud Netflix(已逐步淘汰)
组件 |
功能 |
特点 |
适用场景 |
Eureka |
服务注册与发现 |
成熟稳定,但维护停止,需迁移到其他方案(如Nacos) |
过渡期项目,需兼容旧系统 |
Zuul/Gateway |
API 网关,路由与过滤 |
Zuul 1.x 已停止维护,Spring Cloud Gateway 更推荐 |
需快速搭建网关功能 |
Hystrix |
熔断与降级 |
响应式设计,但代码侵入性强,维护停止 |
需基础熔断功能,但需注意维护状态 |
Feign |
客户端负载均衡与声明式服务调用 |
简单易用,但需与 Ribbon 配合 |
简单 RPC 场景 |
2.2 Spring Cloud Alibaba
组件 |
功能 |
特点 |
适用场景 |
Nacos |
服务注册/发现、动态配置中心 |
阿里巴巴生态,轻量高效,支持云原生,社区活跃 |
新项目,与阿里云/容器化集成 |
Sentinel |
流量控制、熔断、系统负载保护 |
支持实时监控,规则动态调整,支持多语言扩展 |
需高并发场景下的流量控制 |
Dubbo/Spring Cloud |
服务间通信(RPC) |
Dubbo 性能高,Spring Cloud 原生集成 |
复杂服务间通信需求 |
Spring Cloud Gateway |
API 网关,路由、鉴权、限流 |
非侵入式,支持多种过滤器 |
需灵活路由与安全策略 |
2.3 Consul
组件 |
功能 |
特点 |
适用场景 |
Consul |
服务注册/发现、健康检查、Key-Value 配置、多数据中心支持 |
自带配置中心,强一致性,适合分布式系统 |
需强一致性服务发现与配置管理 |
Envoy |
服务网格数据平面,支持流量管理、熔断、链路追踪 |
非侵入式,支持多语言,但需额外部署 |
多语言微服务架构 |
2.4 Istio
组件 |
功能 |
特点 |
适用场景 |
Istio |
服务网格,支持流量管理、熔断、链路追踪、安全策略 |
非侵入式,支持多语言,与 Kubernetes 深度集成 |
云原生环境,多语言微服务架构 |
Pilot |
控制平面,管理服务路由和规则 |
需配合 Envoy 或其他数据平面 |
需复杂流量管理(如金丝雀发布) |
2.5 Apache Dubbo
组件 |
功能 |
特点 |
适用场景 |
Dubbo |
服务注册/发现、高性能 RPC、负载均衡、熔断降级 |
性能高,适合 Java 生态,需配合 ZooKeeper/Nacos |
高性能 Java 微服务架构 |
Dubbo 3.x |
支持云原生,集成 Nacos/Sentinel |
兼容 Spring Cloud,支持多语言扩展 |
需高性能与云原生结合 |
2.6 Kubernetes + Istio
组件 |
功能 |
特点 |
适用场景 |
Kubernetes |
容器编排,服务发现,健康检查 |
生态完善,支持自动化部署与扩缩容 |
容器化部署,需与 Istio 集成 |
Istio |
服务网格,流量管理、安全策略、链路追踪 |
非侵入式,支持多语言,需额外资源消耗 |
云原生环境,多语言服务治理 |
2.7 Service Mesh(如 Linkerd)
组件 |
功能 |
特点 |
适用场景 |
Linkerd |
服务网格,轻量级流量控制、熔断、监控 |
性能低开销,支持多语言,社区活跃 |
需轻量级服务治理与多语言支持 |
2.8 HashiCorp Nomad
组件 |
功能 |
特点 |
适用场景 |
Nomad |
作业调度与服务治理,支持服务发现、健康检查 |
与 Consul 集成,适合混合云环境 |
需统一调度与服务治理的混合云场景 |
3. 核心功能对比表格
框架/方案 |
服务注册发现 |
配置中心 |
熔断降级 |
API 网关 |
分布式事务 |
链路追踪 |
社区活跃度 |
适用场景 |
Spring Cloud Netflix |
Eureka |
自定义 |
Hystrix |
Zuul/Gateway |
不直接支持 |
Sleuth+Zipkin |
逐渐衰退 |
过渡期项目,需兼容旧系统 |
Spring Cloud Alibaba |
Nacos |
Nacos |
Sentinel |
Gateway |
Seata |
Sleuth+Zipkin |
活跃 |
新项目,阿里云生态 |
Consul |
Consul |
Consul |
自定义 |
API Gateway |
不直接支持 |
自定义 |
活跃 |
强一致性分布式系统 |
Istio |
自带发现 |
ConfigMap |
自带熔断 |
自带网关 |
自定义 |
Jaeger |
非常活跃 |
云原生,多语言微服务 |
Dubbo |
ZooKeeper/Nacos |
自定义 |
自带熔断 |
自定义 |
Dubbo-TCC |
SkyWalking |
活跃 |
高性能 Java 架构 |
Kubernetes+Istio |
Kubernetes |
ConfigMap |
Istio |
Istio |
自定义 |
Jaeger |
非常活跃 |
容器化部署,云原生 |
**Service Mesh(Linkerd) |
自带发现 |
自定义 |
自带熔断 |
自带网关 |
自定义 |
自定义 |
活跃 |
需轻量级治理与多语言支持 |
4. 功能实现对比
4.1 服务注册发现
- Nacos:轻量、支持集群、多数据中心。
- Consul:强一致性,内置健康检查。
- Kubernetes:基于 DNS 或 CoreDNS,适合容器环境。
4.2 配置中心
- Nacos:动态配置推送,支持多环境。
- Consul:Key-Value 存储,需自定义监听。
- Spring Cloud Config:基于 Git,适合 GitOps。
4.3 熔断降级
- Sentinel:实时统计,支持多维度限流。
- Hystrix:成熟但维护停止。
- Istio:基于 Sidecar 的流量控制。
4.4 分布式事务
- Seata:AT 模式支持自动事务,需数据库支持。
- Saga:基于消息补偿,适合长事务。
- Istio:通过 Sidecar 实现分布式追踪与补偿。
5. 选择建议
需求场景 |
推荐方案 |
原因 |
Java 生态,快速启动 |
Spring Cloud Alibaba (Nacos+Sentinel) |
阿里生态完善,配置简单,社区活跃。 |
云原生,多语言支持 |
Istio + Kubernetes |
非侵入式,与容器生态深度集成。 |
高性能 RPC,Java 为主 |
Dubbo 3.x + Nacos |
性能高,支持云原生,与 Spring Cloud 兼容。 |
混合云环境,强一致性需求 |
Consul + Envoy |
强一致性服务发现,支持多数据中心。 |
轻量级治理,无需 Sidecar |
Spring Cloud Alibaba |
代码侵入式,适合中小型项目。 |
6. 典型场景代码示例(Spring Cloud Alibaba)
6.1 服务注册与发现(Nacos)
配置 application.yml
:
spring:
application:
name: user-service
cloud:
nacos:
discovery:
server-addr: 127.0.0.1:8848
启动类:
@SpringBootApplication
@EnableDiscoveryClient
public class UserServiceApplication {
public static void main(String[] args) {
SpringApplication.run(UserServiceApplication.class, args);
}
}
6.2 熔断降级(Sentinel)
配置限流规则(JSON):
[
{
"resource": "/users/{id}",
"count": 100,
"grade": 1,
"strategy": 0,
"controlBehavior": 0
}
]
代码注解:
@RestController
public class UserController {
@SentinelResource(value = "getUser", blockHandler = "handleBlock")
@GetMapping("/{id}")
public User getUser(@PathVariable Long id) {
}
public User handleBlock(BlockException e) {
return new User(-1L, "服务不可用");
}
}
6.3 API 网关(Spring Cloud Gateway)
路由配置 application.yml
:
spring:
cloud:
gateway:
routes:
- id: user_route
uri: lb://user-service
predicates:
- Path=/api/users/**
filters:
- StripPrefix=1
6.4 分布式事务(Seata)
配置 application.yml
:
seata:
enabled: true
application-id: ${spring.application.name}
tx-service-group: ${spring.application.name}-group
事务注解:
@Service
public class OrderService {
@GlobalTransactional
public void createOrder() {
userService.createUser();
inventoryService.reduceStock();
}
}
7. 总结表格
框架/方案 |
服务治理能力 |
非侵入性 |
多语言支持 |
学习成本 |
适用场景 |
Spring Cloud Alibaba |
完整 |
部分侵入式 |
有限 |
中等 |
新项目,阿里云生态 |
Istio |
完整 |
非侵入式 |
完全 |
高 |
云原生,多语言微服务 |
Consul |
完整 |
部分侵入式 |
有限 |
中等 |
强一致性分布式系统 |
Dubbo |
完整 |
部分侵入式 |
有限 |
中等 |
高性能 Java 架构 |
Kubernetes+Istio |
完整 |
非侵入式 |
完全 |
高 |
容器化部署,云原生 |
**Service Mesh(Linkerd) |
完整 |
非侵入式 |
完全 |
高 |
轻量级治理,多语言支持 |
8. 注意事项
- Spring Cloud Netflix:组件(如 Hystrix)已停止维护,建议迁移到 Resilience4j 或 Sentinel。
- Istio:需部署 Sidecar,资源消耗较高,适合云原生环境。
- Nacos:支持服务发现与配置中心一体化,适合快速搭建。
- Seata:需数据库支持,适合需强事务一致性的场景。
通过以上对比和示例,开发者可根据项目需求选择合适的治理方案。对于新项目,Spring Cloud Alibaba 或 Istio 是主流选择,前者适合 Java 生态,后者适合云原生多语言架构。