1.标准库中的string类
在 C++ 里,string
类属于标准库的一部分,它在<string>
头文件中定义,用于处理和操作字符串。
1.1string类的常用接口说明
1.1.1. string类对象的常见构造
string() (重点)
|
构造空的string类对象,即空字符串
|
string(const char* s) (重点)
|
用C-string来构造string类对象
|
string(size_t n, char c)
|
string类对象中包含n个字符c
|
string(const string&s) (重点)
|
拷贝构造函数
|
void Teststring()
{
string s1; // 构造空的string类对象s1
string s2("hello bit"); // 用C格式字符串构造string类对象s2
string s3(5,'a');//构造n个字符c的类对象
string s4(s2); // 拷贝构造s3
}
1.1.2. string类对象的容量操作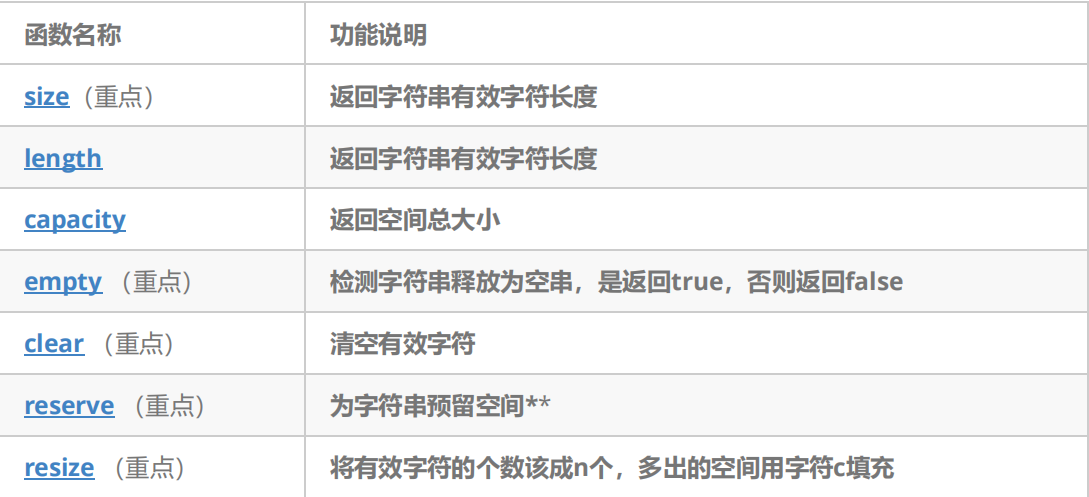
void Teststring1()
{
// 注意:string类对象支持直接用cin和cout进行输入和输出
string s("hello, bit!!!");
cout << s.size() << endl;
cout << s.length() << endl;
cout << s.capacity() << endl;
cout << s << endl;
// 将s中的字符串清空,注意清空时只是将size清0,不改变底层空间的大小
s.clear();
cout << s.size() << endl;
cout << s.capacity() << endl;
// 将s中有效字符个数增加到10个,多出位置用'a'进行填充
// “aaaaaaaaaa”
s.resize(10, 'a');
cout << s.size() << endl;
cout << s.capacity() << endl;
// 将s中有效字符个数增加到15个,多出位置用缺省值'\0'进行填充
// "aaaaaaaaaa\0\0\0\0\0"
// 注意此时s中有效字符个数已经增加到15个
s.resize(15);
cout << s.size() << endl;
cout << s.capacity() << endl;
cout << s << endl;
// 将s中有效字符个数缩小到5个
s.resize(5);
cout << s.size() << endl;
cout << s.capacity() << endl;
cout << s << endl;
}
void Teststring2()
{
string s;
// 测试reserve是否会改变string中有效元素个数
s.reserve(100);
cout << s.size() << endl;
cout << s.capacity() << endl;
// 测试reserve参数小于string的底层空间大小时,是否会将空间缩小
s.reserve(50);
cout << s.size() << endl;
cout << s.capacity() << endl;
}
// 利用reserve提高插入数据的效率,避免增容带来的开销
注意:
1. size()与length()方法底层实现原理完全相同,引入size()的原因是为了与其他容器的接口保持一
致,一般情况下基本都是用size()。
2. clear()只是将string中有效字符清空,不改变底层空间大小。
3. resize(size_t n) 与 resize(size_t n, char c)都是将字符串中有效字符个数改变到n个,不同的是当字符个数增多时:resize(n)用0来填充多出的元素空间,resize(size_t n, char c)用字符c来填充多出的元素空间。注意:resize在改变元素个数时,如果是将元素个数增多,可能会改变底层容量的大
小,如果是将元素个数减少,底层空间总大小不变,但会删除数据。
4. reserve(size_t res_arg=0):为string预留空间,不改变有效元素个数,当reserve的参数小于
string的底层空间总大小时,reserver不会改变容量大小(缩容时,如果有数据就不会改变容量大小,如果clear以后,没有数据就会缩容)。
1.1.3. string类对象的访问及遍历操作
void Teststring4()
{
string s("hello world");
// 3种遍历方式:
// 需要注意的以下三种方式除了遍历string对象,还可以遍历可以是修改string中的字符,
// 1. for+operator[]
for (size_t i = 0; i < s.size(); ++i)
cout << s[i];
cout << endl;
// 2.迭代器
string::iterator it = s.begin();
while (it != s.end())
{
cout << *it ;
++it;
}
cout << endl;
//反向迭代器
// string::reverse_iterator rit = s.rbegin();
// C++11之后,直接使用auto定义迭代器,让编译器推到迭代器的类型
auto rit = s.rbegin();
while (rit != s.rend())
{
cout << *rit;
++rit;
}
cout << endl;
// 3.范围for
for (auto ch : s)
cout << ch ;
cout << endl;
}
const迭代器
不允许修改字符串的内容
void Func(const string& s)
{
string::const_iterator it = s.begin();
while (it != s.end())
{
// *it += 2; 错误
cout << *it << " ";
++it;
}
cout << endl;
//string::const_reverse_iterator rit = s.rbegin();
auto rit = s.rbegin();
while (rit != s.rend())
{
// (*rit) += 3; 错误
cout << *rit << " ";
++rit;
}
}
string还有一个at函数,功能和operator[]基本一致,不过两者对越界的处理不一样,at函数采用抛异常,operator[]则是断言。
void Teststring5()
{
string s("hello world");
char x=s.at(0);//读
cout<<x<<endl;
s.at(0)='a';//写
cout<<s<<endl;
}
注意以上的函数在遍历的时候都可以对字符串对象进行修改。
1.1.4. string类对象的修改操作
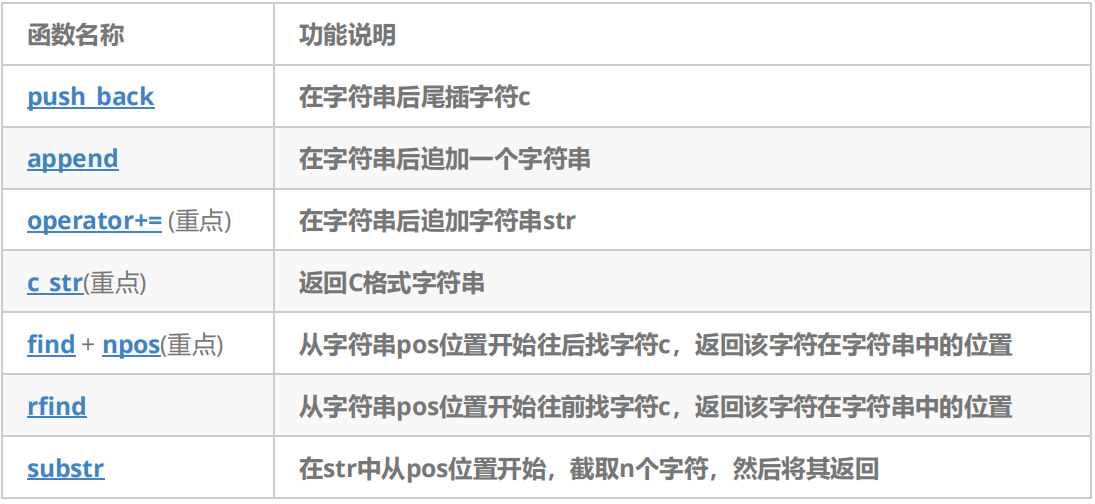
void Teststring6()
{
string str;
//push_back一次只能插入一个字符
str.push_back(' '); // 在str后插入空格
str.append("hello"); // 在str后追加一个字符"hello"
str += 'b'; // 在str后追加一个字符'b'
str += "it"; // 在str后追加一个字符串"it"
cout << str << endl;
//c_str会返回一个指向以空字符结尾的字符数组的常量指针,此字符数组存储着string 对象中的内容。
//和c的一些接口函数配合使用
//以C语言的方式打印字符串(也就是以空字符 '\0' 结尾的字符数组)
cout << str.c_str() << endl;
// 获取file的后缀
string file("string.cpp");
size_t pos = file.rfind('.');
string suffix(file.substr(pos, file.size() - pos));
cout << suffix << endl;
// npos是string里面的一个静态成员变量
// static const size_t npos = -1;
// 取出url中的域名
string url("http://www.cplusplus.com/reference/string/string/find/");
cout << url << endl;
//找不到返回npos
size_t start = url.find("://");
if (start == string::npos)
{
cout << "invalid url" << endl;
return;
}
start += 3;
size_t finish = url.find('/', start);
string address = url.substr(start, finish - start);
cout << address << endl;
// 删除url的协议前缀
pos = url.find("://");
url.erase(0, pos + 3);
cout << url << endl;
}
还有insert(插入),erase(删除),assign(赋值,会覆盖原有的字符串),replace(替换)等函数,用的时候可以查文档进行使用。
字符串匹配函数
读取一行字符串,cin和scanf都有一个特性遇到空格和换行就停止,剩下的放在缓冲区,等待下一次读取,用getline可以读取一行遇到换行符停止。这个函数为非成员函数。
把数据类型转换成字符串
把字符串转化为其他类型