日期类实现
- C++ 日期类的实现与深度解析
-
- 一、代码结构概览
-
- 1.1 头文件 `Date.h`
- 1.2 源文件 `Date.cpp`
- 二、关键函数实现解析
-
- 2.1 获取某月天数函数 `GetMonthDay`
- 2.2 构造函数 `Date`
- 2.3 日期加减法运算
- 2.4 前置与后置自增/自减操作
- 2.5 日期比较与差值计算
- 三、代码优化与注意事项
-
- 3.1 代码优化
- 3.2 注意事项
- 四、总结
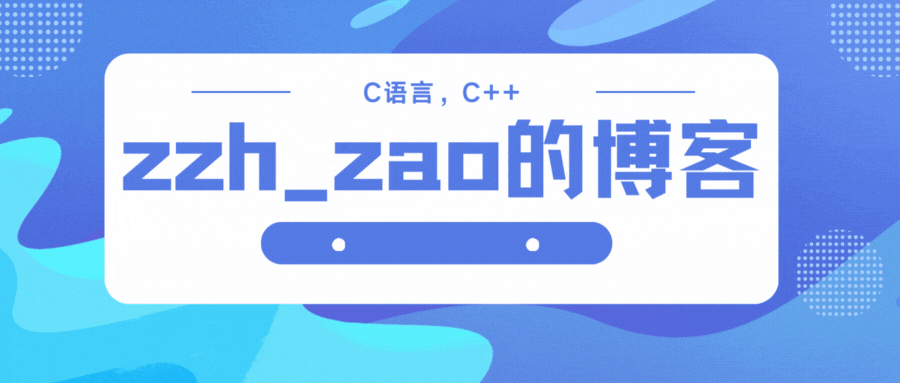
C++ 日期类的实现与深度解析
在C++编程中,自定义数据类型是构建复杂应用的基础。日期作为一个常用的数据类型,涉及到多种操作,如日期的加减、比较、计算间隔天数等。本文是对前几篇C++博客的应用。
一、代码结构概览
我们实现的Date
类包含了日期相关的核心功能,代码分为头文件Date.h
和源文件Date.cpp
两部分。头文件负责类的声明,定义类的成员函数接口和数据成员;源文件则实现这些成员函数,完成具体的业务逻辑。
1.1 头文件 Date.h
// Date.h
#pragma once
#include <iostream>
#include <assert.h>
using namespace std;
class Date
{
public:
// 获取某年某月的天数
int GetMonthDay(int year, int month) const;
// 全缺省的构造函数
Date(int year = 1900, int month = 1, int day = 1);
// 拷贝构造函数
Date(const Date& d);
// 赋值运算符重载
Date& operator=(const Date& d);
// 析构函数
~Date();
// 日期+=天数
Date& operator+=(int day);
// 日期+天数
Date operator+(int day) const;
// 日期-天数
Date operator-(int day) const;
// 日期-=天数
Date& operator-=(int day);
// 前置++
Date& operator++();
// 后置++
Date operator++(int);
// 后置--
Date operator--(int);
// 前置--
Date& operator--();
// >运算符重载
bool operator>(const Date& d) const;
// ==运算符重载
bool operator==(const Date& d) const;
// >=运算符重载
bool operator >= (const Date& d) const;
// <运算符重载
bool operator < (const Date& d) const;
// <=运算符重载
bool operator <= (const Date& d) const;
// !=运算符重载
bool operator != (const Date& d) const;
// 日期-日期 返回天数
int operator-(const Date& d) const;
// 输出日期
void Print() const;
private:
int _year;
int _month;
int _day;
};
头文件中定义了Date
类,包含私有成员变量_year
、_month
、_day
,用于存储日期的年、月、日信息;同时声明了一系列成员函数,涵盖日期计算、比较、赋值等操作。
1.2 源文件 Date.cpp
// Date.cpp
#define _CRT_SECURE_NO_WARNINGS 1
#include "Date.h"
// 实现获取某年某月天数的函数
int Date::GetMonthDay(int year, int month) const
{
assert(month > 0 && month < 13);
static int arr[13] = { 0,31,28,31,30,31,30,31,31,30,31,30,31 };
if (month == 2 && ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0))
return 29;
return arr[month];
}
// 全缺省构造函数,同时检查日期合法性
Date::Date(int year, int month, int day)
{
// 检查日期合法性
if (year < 0 || month < 1 || month > 12 || day < 1 || day > GetMonthDay(year, month))
{
cout << "Invalid date: " << year << "-" << month << "-" << day << endl;
// 使用默认值
_year = 1900;
_month = 1;
_day = 1;
}
else
{
_year = year;
_month = month;
_day = day;
}
}
// 拷贝构造函数
Date::Date(const Date& d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
// 赋值运算符重载
Date& Date::operator=(const Date& d)
{
if (this != &d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
return *this;
}
// 析构函数,无需显式将成员置零
Date::~Date()
{
// 不需要在这里将成员置零
}
// 日期加上指定天数
Date& Date::operator+=(int day)
{
if (day < 0)
{
return *this -= -day;
}
_day += day;
while (_day > GetMonthDay(_year, _month))
{
_day -= GetMonthDay(_year, _month);
_month++;
if (_month == 13)
{
_month = 1;
_year++;
}
}
return *this;
}
// 日期加上指定天数,返回新的日期对象
Date Date::operator+(int day) const
{
Date tmp = *this;
tmp += day;
return tmp;
}
// 日期减去指定天数
Date& Date::operator-=(int day)
{
if (day < 0)
{
return *this += -day;
}
_day -= day;
while (_day <= 0)
{
_month--;
if (_month == 0)
{
_month = 12;
_year--;
}
_day += GetMonthDay(_year, _month);
}
return *this;
}
// 日期减去指定天数,返回新的日期对象
Date Date::operator-(int day) const
{
Date tmp = *this;
tmp -= day;
return tmp;
}
// 前置++操作
Date& Date::operator++()
{
*this += 1;
return *this;
}
// 后置++操作
Date Date::operator++(int)
{
Date tmp(*this);
*this += 1;
return tmp;
}
// 后置--操作
Date Date::operator--(int)
{
Date tmp(*this);
*this -= 1;
return tmp;
}
// 前置--操作
Date& Date::operator--()
{
*this -= 1;
return *this;
}
// 比较两个日期大小
bool Date::operator>(const Date& d) const
{
if (_year > d._year)
return true;
else if (_year == d._year)
{
if (_month > d._month)
return true;
else if (_month == d._month)
return _day > d._day;
}
return false;
}
// 判断两个日期是否相等
bool Date::operator==(const Date& d) const
{
return _year == d._year && _month == d._month && _day == d._day;
}
// 判断一个日期是否大于等于另一个日期
bool Date::operator >= (const Date& d) const
{
return *this > d || *this == d;
}
// 判断一个日期是否小于另一个日期
bool Date::operator < (const Date& d) const
{
return !(*this >= d);
}
// 判断一个日期是否小于等于另一个日期
bool Date::operator <= (const Date& d) const
{
return !(*this > d);
}
// 判断两个日期是否不相等
bool Date::operator != (const Date& d) const
{
return !(*this == d);
}
// 计算两个日期之间的天数差
int Date::operator-(const Date& d) const
{
Date min = *this;
Date max = d;
int flag = 1;
if (min > max)
{
min = d;
max = *this;
flag = -1;
}
int days = 0;
// 优化算法:逐月计算天数差
while (min < max)
{
// 计算下个月1号的日期
Date nextMonth(min._year, min._month + 1, 1);
if (nextMonth > max)
{
// 如果下个月超过了max,则直接计算当前月剩余天数
days += max._day - min._day;
break;
}
else
{
// 计算当前月的剩余天数
days += GetMonthDay(min._year, min._month) - min._day + 1;
// 跳到下个月1号
min = nextMonth;
}
}
return days * flag;
}
// 输出日期
void Date::Print() const
{
cout << _year << "-" << _month << "-" << _day << endl;
}
源文件中具体实现了头文件声明的各个成员函数,从基础的日期创建、拷贝、赋值,到复杂的日期计算与比较,每个函数各司其职,共同完成日期类的功能。
二、关键函数实现解析
2.1 获取某月天数函数 GetMonthDay
int Date::GetMonthDay(int year, int month) const
{
assert(month > 0 && month < 13);
static int arr[13] = { 0,31,28,31,30,31,30,31,31,30,31,30,31 };
if (month == 2 && ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0))
return 29;
return arr[month];
}
该函数用于获取指定年份和月份的天数。通过一个静态数组arr
存储常规月份的天数,并根据闰年规则(能被4整除但不能被100整除,或者能被400整除)判断2月的天数。函数声明为const
成员函数,表明不会修改对象的状态,也允许常量对象调用。
2.2 构造函数 Date
Date::Date(int year, int month, int day)
{
// 检查日期合法性
if (year < 0 || month < 1 || month > 12 || day < 1 || day > GetMonthDay(year, month))
{
cout << "Invalid date: " << year << "-" << month << "-" << day << endl;
// 使用默认值
_year = 1900;
_month = 1;
_day = 1;
}
else
{
_year = year;
_month = month;
_day = day;
}
}
构造函数用于初始化Date
对象。在初始化前,对传入的年份、月份和日期进行合法性检查,若日期不合法,则将对象初始化为默认日期1900-01-01
,保证对象的有效性。
2.3 日期加减法运算
在这里为了减少类类型的拷贝,节约资源,通常先实现+= 或 -=;
Date& Date::operator+=(int day)
{
if (day < 0)
{
return *this -= -day;
}
_day += day;
while (_day > GetMonthDay(_year, _month))
{
_day -= GetMonthDay(_year, _month);
_month++;
if (_month == 13)
{
_month = 1;
_year++;
}
}
return *this;
}
Date Date::operator+(int day) const
{
Date tmp = *this;
tmp += day;
return tmp;
}
operator+=
函数实现了日期加上指定天数的功能,通过循环处理跨月、跨年的情况;operator+
函数则基于operator+=
,返回一个新的日期对象,保持原对象不变。类似地,operator-=
和operator-
函数实现了日期减法操作。
2.4 前置与后置自增/自减操作
为区分前置与后置,后置类型要在括号当中加入int形参,与前置构成重载;
Date& Date::operator++()
{
*this += 1;
return *this;
}
Date Date::operator++(int)
{
Date tmp(*this);
*this += 1;
return tmp;
}
前置自增operator++
先对日期进行加1操作,再返回修改后的对象;后置自增operator++(int)
通过创建临时对象保存原始状态,对原对象进行加1操作后,返回临时对象,保证后置自增“先使用,后修改”的语义。自减操作operator--
和operator--(int)
的实现原理与之类似。
2.5 日期比较与差值计算
在自我实现日期比较时,只需实现一个>或<,加上一个=,其余的都可以用这两个来实现。
bool Date::operator>(const Date& d) const
{
if (_year > d._year)
return true;
else if (_year == d._year)
{
if (_month > d._month)
return true;
else if (_month == d._month)
return _day > d._day;
}
return false;
}
int Date::operator-(const Date& d) const
{
Date min = *this;
Date max = d;
int flag = 1;
if (min > max)
{
min = d;
max = *this;
flag = -1;
}
int days = 0;
// 优化算法:逐月计算天数差
while (min < max)
{
// 计算下个月1号的日期
Date nextMonth(min._year, min._month + 1, 1);
if (nextMonth > max)
{
// 如果下个月超过了max,则直接计算当前月剩余天数
days += max._day - min._day;
break;
}
else
{
// 计算当前月的剩余天数
days += GetMonthDay(min._year, min._month) - min._day + 1;
// 跳到下个月1号
min = nextMonth;
}
}
return days * flag;
}
日期比较函数通过依次比较年份、月份和日期,判断两个日期的大小关系;operator-
函数用于计算两个日期之间的天数差,采用逐月计算的优化算法,减少不必要的循环次数,提高计算效率。
三、代码优化与注意事项
3.1 代码优化
- 成员函数添加
const
修饰:将不修改对象状态的成员函数声明为const
,如GetMonthDay
、日期比较函数等,提高代码的安全性和可读性,同时允许常量对象调用这些函数。 - 日期差值计算优化:在计算两个日期差值时,采用逐月计算的方式,避免了每次只增加一天的低效循环,大幅提升计算效率。
3.2 注意事项
- 日期合法性检查:在构造函数和其他涉及日期修改的函数中,要确保对日期的合法性进行严格检查,防止出现无效日期。
- 运算符重载的一致性:在重载日期相关运算符时,要保证逻辑的一致性和正确性,例如
operator+
和operator+=
之间的关系,避免出现逻辑矛盾。 - 避免内存泄漏:虽然
Date
类中没有动态内存分配,但在更复杂的类设计中,析构函数要正确释放资源,防止内存泄漏。
四、总结
通过实现Date
类,运用了C++中类的设计、运算符重载、构造函数、析构函数等核心概念。日期类的实现不仅涉及到基本的数学计算,还需要处理各种边界情况和逻辑判断。