一.概念
1、 继承是Java中实现代码重用的重要手段之一。Java中只支持单根继承,即一个类只能有一个直接父类。Object类是所有Java类的祖先。
目的:减少对代码的书写
2.修改后:
3.使用继承框架
4.案例:
将公共的特性写入父元素
package cn.bdqn.demo03;
//一个类如果没有明确声明其父类是谁,则默认继承Object类
public class Father {
private String name;
public Father() {
super();//调用父类Object类中的无参构造方法
System.out.println("我是Father类中的无参构造方法");
}
public Father(String name) {
super();//调用父类Object类中的无参构造方法
this.name = name;
System.out.println("我是Father类中的有参构造方法");
}
5. 自己特有的属性 类名 方法
package cn.bdqn.demo02;
public class Animal {
// 将demo01包中Dog类和Penguin类中相同的代码提取到这个Animal类中
private String name;
private int health;
private int love;
public Animal() {
}
public Animal(String name, int health, int love) {
this.name = name;
this.health = health;
this.love = love;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getHealth() {
return health;
}
public void setHealth(int health) {
this.health = health;
}
public int getLove() {
return love;
}
public void setLove(int love) {
this.love = love;
}
public void print() {
System.out.println("宠物信息-->昵称:" + this.getName() + ",健康值:"
+ this.getHealth() + ",亲密度:" + this.getLove());
}
package cn.bdqn.demo03;
public class Test {
public static void main(String[] args) {
//创建Son类对象
// Son son1 = new Son();
Son son2 = new Son("张三", 12);
}
小结
super关键字来访问父类的成员
super只能出现在子类的方法和构造方法中
super调用构造方法时,只能是第一句
super不能访问父类的private成员
二.继承条件下的构造方法
2.1子类继承父类的什么?
继承public和protected修饰的属性和方法,不管子类和父类是否在同一个包里
继承默认权限修饰符修饰的属性和方法,但子类和父类必须在同一个包里
图解;
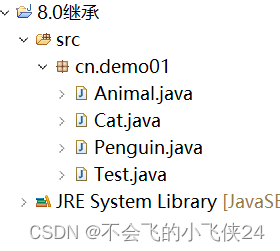
2.1子类可以继承父类的所有资源吗?
三.set和get的引入
使用条件:当修饰类属性为私有时
1.如何生成:
先创建有参 无参
2.创建
选择deselect All 无参
勾选 select All 有参
3.结果
4. set 和 get方法创建
5.完整案例显示;
/父类
package cn.demo01;
public class Animal {
//属性
private String name;
private int health;
private int love;
//无参
public Animal() {
super();
}
//有参
public Animal(String name, int health, int love) {
super();
this.name = name;
this.health = health;
this.love = love;
}
//公共的
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getHealth() {
return health;
}
public void setHealth(int health) {
this.health = health;
}
public int getLove() {
return love;
}
public void setLove(int love) {
this.love = love;
}
public void print() {
System.out.println("宠物信息-->昵称:" + this.getName() + ",健康值:"
+ this.getHealth() + ",亲密度:" + this.getLove());
}
///子类1
package cn.demo01;
//extends 继承属性 用来引出extends的父元素
public class Cat extends Animal {
//cat独有的属性
private String strain;
//引参
public Cat() {
super();//父类Animal的无参
}
//有参
public Cat(String name, int health, int love, String strain) {
super(name, health, love);
this.strain = strain;
}
//构造器
public String getStrain() {
return strain;
}
public void setStrain(String strain) {
this.strain = strain;
}
//定义特有的方法
public void eat(){
System.out.println("狗吃骨头......");
}
}
///子类2
package cn.demo01;
public class Penguin extends Animal {
private String sex;
//无参
public Penguin() {
super();
}
//有参
public Penguin(String name, int health, int love, String sex) {
super(name, health, love);
this.sex = sex;
}
//get set
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
//
public void swimming(){
System.out.println("企鹅会----------");
}
}
package cn.demo01;
public class Test extends Animal {
public static void main(String[] args) {
// 创建Cat对象
Cat c1 = new Cat();//无参
// 父中的继承
c1.setHealth(100);
c1.setLove(20);
c1.setName("xiaobai");
// Cat本类继承
c1.setStrain("wowo");
c1.eat();
//创建对象
Penguin p1 = new Penguin("QQ", 90, 98, "公");
p1.print();
// swimming()是Penguin类中的
p1.swimming();
//
}
四.访问权限修饰符:
类访问权限修饰符
public:公有访问级别
默认修饰符:包级私有,只有在同一个包中的类才能访问默认修饰福修饰的类
类成员访问修饰符:
本类 同包非子类 不同包非子类 同包子类 不同包子类
public √ √ √ √ √
protected √ √ × √ ×
默认修饰符 √ √ × √ ×
private √ × × × ×
static关键字:
static变量:属于类,可以通过类名直接调用
static方法:属于类,可以通过类名直接调用
static代码块:属于类,随着类的加载而运行