leetcode 355. 设计推特-https://leetcode.cn/problems/design-twitter/
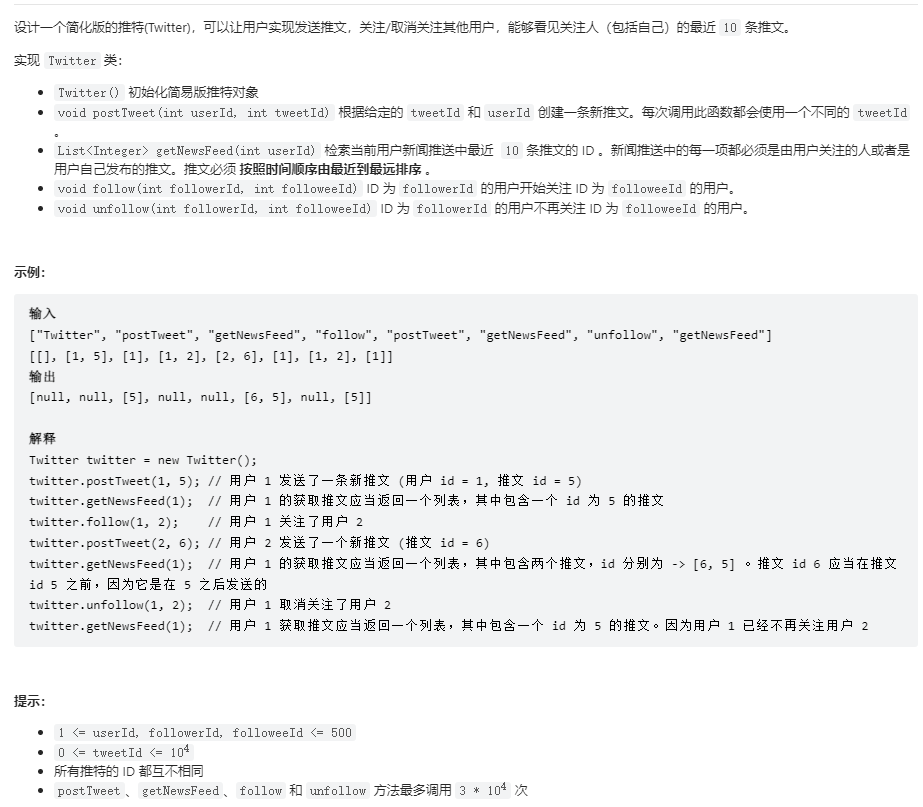
class Twitter {
public Twitter() {
}
public void postTweet(int userId, int tweetId) {
}
public List<Integer> getNewsFeed(int userId) {
}
public void follow(int followerId, int followeeId) {
}
public void unfollow(int followerId, int followeeId) {
}
}
方法一
class Twitter0
{
public static void main(String[] args) {
Twitter0 Twitter0 = new Twitter0();
Twitter0.postTweet(1, 5);
System.out.println(Twitter0.getNewsFeed(1));
Twitter0.follow(1, 2);
Twitter0.postTweet(2, 6);
System.out.println(Twitter0.getNewsFeed(1));
Twitter0.unfollow(1, 2);
System.out.println(Twitter0.getNewsFeed(1));
}
static Map<Integer, MyInfo> peopleMap;
static int time;
public Twitter0() {
peopleMap = new HashMap<>();
time = 0;
}
public void postTweet(int userId, int tweetId) {
TidAndTime tidAndTime = new TidAndTime(tweetId, getNowTime());
if (peopleMap.containsKey(userId)) {
MyInfo myInfo = peopleMap.get(userId);
myInfo.getTidAndTimeList().add(tidAndTime);
}
else {
MyInfo myInfo = new MyInfo();
ArrayList<TidAndTime> tidAndTimeList = new ArrayList<>();
tidAndTimeList.add(tidAndTime);
myInfo.setTidAndTimeList(tidAndTimeList);
peopleMap.put(userId, myInfo);
}
}
public List<Integer> getNewsFeed(int userId) {
List<Integer> rtnList = new ArrayList<>();
if (!peopleMap.containsKey(userId)) {
return rtnList;
}
List<TidAndTime> lastList = new ArrayList<>();
MyInfo myInfo = peopleMap.get(userId);
List<TidAndTime> tidAndTimeList = myInfo.getTidAndTimeList();
lastList.addAll(tidAndTimeList);
List<Integer> focksList = myInfo.getFocksList();
List<TidAndTime> focksTidAndTimeList = getfocksFeed(focksList);
lastList.addAll(focksTidAndTimeList);
boolean change = false;
for (int i = 0, len = lastList.size(); i < len - 1; i++) {
for (int j = 0; j < len - i - 1; j++) {
TidAndTime tidAndTime = lastList.get(j);
TidAndTime tidAndTime2 = lastList.get(j + 1);
if (tidAndTime.getTime() < tidAndTime2.getTime()) {
lastList.set(j, tidAndTime2);
lastList.set(j + 1, tidAndTime);
change = true;
}
}
if (!change) {
break;
}
}
int size = lastList.size();
if (size > 10) {
size = 10;
}
for (int i = 0; i < size; i++) {
rtnList.add(lastList.get(i).getTweetId());
}
return rtnList;
}
public void follow(int followerId, int followeeId) {
if (peopleMap.containsKey(followerId)) {
MyInfo myInfo = peopleMap.get(followerId);
if (!myInfo.getFocksList().contains(followeeId)) {
myInfo.getFocksList().add(followeeId);
}
}
else {
MyInfo myInfo = new MyInfo();
ArrayList<Integer> focksList = new ArrayList<>();
focksList.add(followeeId);
myInfo.setFocksList(focksList);
peopleMap.put(followerId, myInfo);
}
}
public void unfollow(int followerId, int followeeId) {
if (peopleMap.containsKey(followerId)) {
MyInfo myInfo = peopleMap.get(followerId);
List<Integer> focksList = myInfo.getFocksList();
if (focksList.contains(followeeId)) {
List<Integer> newForks = new ArrayList<>();
for (Integer integer : focksList) {
if (!integer.equals(followeeId)) {
newForks.add(integer);
}
}
myInfo.setFocksList(newForks);
}
}
}
public static List<TidAndTime> getfocksFeed(List<Integer> userIdList) {
List<TidAndTime> rtnList = new ArrayList<>();
if (userIdList == null || userIdList.isEmpty()) {
return rtnList;
}
for (Integer userId : userIdList) {
if (!peopleMap.containsKey(userId)) {
continue;
}
MyInfo myInfo = peopleMap.get(userId);
rtnList.addAll(myInfo.getTidAndTimeList());
}
return rtnList;
}
public static long getNowTime() {
return time++;
}
class MyInfo
{
private List<TidAndTime> tidAndTimeList;
private List<Integer> focksList;
public MyInfo() {
tidAndTimeList = new ArrayList<>();
focksList = new ArrayList<>();
}
public List<TidAndTime> getTidAndTimeList() {
return tidAndTimeList;
}
public void setTidAndTimeList(List<TidAndTime> tidAndTimeList) {
this.tidAndTimeList = tidAndTimeList;
}
public List<Integer> getFocksList() {
return focksList;
}
public void setFocksList(List<Integer> focksList) {
this.focksList = focksList;
}
}
class TidAndTime
{
private int tweetId;
private long time;
public TidAndTime(int tweetId, long time) {
this.tweetId = tweetId;
this.time = time;
}
public int getTweetId() {
return tweetId;
}
public void setTweetId(int tweetId) {
this.tweetId = tweetId;
}
public long getTime() {
return time;
}
public void setTime(long time) {
this.time = time;
}
}
}
方法2-优先级队列
class Twitter2
{
public static void main(String[] args) {
Twitter2 Twitter2 = new Twitter2();
Twitter2.postTweet(1, 5);
System.out.println(Twitter2.getNewsFeed(1));
Twitter2.follow(1, 2);
Twitter2.postTweet(2, 6);
System.out.println(Twitter2.getNewsFeed(1));
Twitter2.unfollow(1, 2);
System.out.println(Twitter2.getNewsFeed(1));
}
static Map<Integer, MyInfo> peopleMap;
static int time;
public Twitter2() {
peopleMap = new HashMap<>();
time = 0;
}
public void postTweet(int userId, int tweetId) {
TidAndTime tidAndTime = new TidAndTime(tweetId, getNowTime());
MyInfo myInfo = peopleMap.getOrDefault(userId, new MyInfo());
myInfo.getTidAndTimeList().add(tidAndTime);
peopleMap.putIfAbsent(userId, myInfo);
}
public List<Integer> getNewsFeed(int userId) {
List<Integer> rtnList = new ArrayList<>();
if (!peopleMap.containsKey(userId)) {
return rtnList;
}
PriorityQueue<TidAndTime> queue = new PriorityQueue<>((o1, o2) -> -(o1.getTime() - o2.getTime()));
MyInfo myInfo = peopleMap.get(userId);
List<TidAndTime> tidAndTimeList = myInfo.getTidAndTimeList();
for (TidAndTime tidAndTime : tidAndTimeList) {
queue.offer(tidAndTime);
}
Set<Integer> focksSet = myInfo.getFocksSet();
List<TidAndTime> focksTidAndTimeList = getfocksFeed(focksSet);
for (TidAndTime tidAndTime : focksTidAndTimeList) {
queue.offer(tidAndTime);
}
while (!queue.isEmpty() && rtnList.size() < 10) {
rtnList.add(queue.poll().getTweetId());
}
return rtnList;
}
public void follow(int followerId, int followeeId) {
MyInfo myInfo = peopleMap.getOrDefault(followerId, new MyInfo());
myInfo.getFocksSet().add(followeeId);
peopleMap.putIfAbsent(followerId, myInfo);
}
public void unfollow(int followerId, int followeeId) {
MyInfo myInfo = peopleMap.get(followerId);
if (myInfo != null) {
myInfo.getFocksSet().remove(followeeId);
}
}
public static List<TidAndTime> getfocksFeed(Set<Integer> userIdSet) {
List<TidAndTime> rtnList = new ArrayList<>();
if (userIdSet == null || userIdSet.isEmpty()) {
return rtnList;
}
for (Integer userId : userIdSet) {
if (!peopleMap.containsKey(userId)) {
continue;
}
MyInfo myInfo = peopleMap.get(userId);
rtnList.addAll(myInfo.getTidAndTimeList());
}
return rtnList;
}
public static int getNowTime() {
return time++;
}
class MyInfo
{
private List<TidAndTime> tidAndTimeList;
private Set<Integer> focksSet;
public MyInfo() {
tidAndTimeList = new ArrayList<>();
focksSet = new HashSet<>();
}
public List<TidAndTime> getTidAndTimeList() {
return tidAndTimeList;
}
public void setTidAndTimeList(List<TidAndTime> tidAndTimeList) {
this.tidAndTimeList = tidAndTimeList;
}
public Set<Integer> getFocksSet() {
return focksSet;
}
public void setFocksSet(Set<Integer> focksSet) {
this.focksSet = focksSet;
}
}
class TidAndTime
{
private int tweetId;
private int time;
public TidAndTime(int tweetId, int time) {
this.tweetId = tweetId;
this.time = time;
}
public int getTweetId() {
return tweetId;
}
public void setTweetId(int tweetId) {
this.tweetId = tweetId;
}
public int getTime() {
return time;
}
public void setTime(int time) {
this.time = time;
}
}
}
方法三-集合排序,比较器
class Twitter3
{
static Map<Integer, MyInfo> peopleMap;
static int time;
public Twitter3() {
peopleMap = new HashMap<>();
time = 0;
}
public void postTweet(int userId, int tweetId) {
TidAndTime tidAndTime = new TidAndTime(tweetId, getNowTime());
MyInfo myInfo = peopleMap.getOrDefault(userId, new MyInfo());
myInfo.getTidAndTimeList().add(tidAndTime);
peopleMap.putIfAbsent(userId, myInfo);
}
public List<Integer> getNewsFeed(int userId) {
List<Integer> rtnList = new ArrayList<>();
if (!peopleMap.containsKey(userId)) {
return rtnList;
}
List<TidAndTime> lastList = new ArrayList<>();
MyInfo myInfo = peopleMap.get(userId);
List<TidAndTime> tidAndTimeList = myInfo.getTidAndTimeList();
lastList.addAll(tidAndTimeList);
Set<Integer> focksSet = myInfo.getFocksSet();
List<TidAndTime> focksTidAndTimeList = getfocksFeed(focksSet);
lastList.addAll(focksTidAndTimeList);
Collections.sort(lastList, new Comparator<TidAndTime>()
{
@Override
public int compare(TidAndTime o1, TidAndTime o2) {
return -(o1.getTime() - o2.getTime());
}
});
int size = lastList.size();
if (size > 10) {
size = 10;
}
for (int i = 0; i < size; i++) {
rtnList.add(lastList.get(i).getTweetId());
}
return rtnList;
}
public void follow(int followerId, int followeeId) {
MyInfo myInfo = peopleMap.getOrDefault(followerId, new MyInfo());
myInfo.getFocksSet().add(followeeId);
peopleMap.putIfAbsent(followerId, myInfo);
}
public void unfollow(int followerId, int followeeId) {
MyInfo myInfo = peopleMap.get(followerId);
if (myInfo != null) {
myInfo.getFocksSet().remove(followeeId);
}
}
public static List<TidAndTime> getfocksFeed(Set<Integer> userIdSet) {
List<TidAndTime> rtnList = new ArrayList<>();
if (userIdSet == null || userIdSet.isEmpty()) {
return rtnList;
}
for (Integer userId : userIdSet) {
if (!peopleMap.containsKey(userId)) {
continue;
}
MyInfo myInfo = peopleMap.get(userId);
rtnList.addAll(myInfo.getTidAndTimeList());
}
return rtnList;
}
public static int getNowTime() {
return time++;
}
class MyInfo
{
private List<TidAndTime> tidAndTimeList;
private Set<Integer> focksSet;
public MyInfo() {
tidAndTimeList = new ArrayList<>();
focksSet = new HashSet<>();
}
public List<TidAndTime> getTidAndTimeList() {
return tidAndTimeList;
}
public void setTidAndTimeList(List<TidAndTime> tidAndTimeList) {
this.tidAndTimeList = tidAndTimeList;
}
public Set<Integer> getFocksSet() {
return focksSet;
}
public void setFocksSet(Set<Integer> focksSet) {
this.focksSet = focksSet;
}
}
class TidAndTime
{
private int tweetId;
private int time;
public TidAndTime(int tweetId, int time) {
this.tweetId = tweetId;
this.time = time;
}
public int getTweetId() {
return tweetId;
}
public void setTweetId(int tweetId) {
this.tweetId = tweetId;
}
public int getTime() {
return time;
}
public void setTime(int time) {
this.time = time;
}
}
}