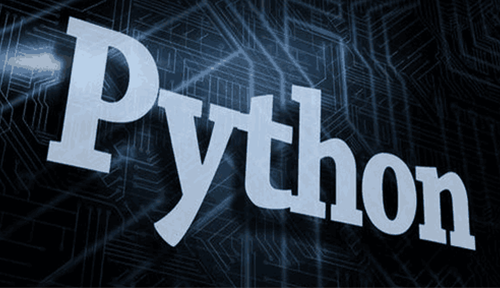
引言
动态钟表是一种直观且实用的UI元素,能够实时显示当前时间。在这篇博客中,我们将使用Python创建一个动态钟表,通过利用Pygame库来实现这一效果。你将看到如何绘制时针、分针和秒针,并使其根据实际时间进行旋转。
准备工作
前置条件
在开始之前,你需要确保你的系统已经安装了Pygame库。如果你还没有安装它,可以使用以下命令进行安装:
pip install pygame
Pygame是一个跨平台的Python模块,用于编写视频游戏。它包括计算机图形和声音库,使得游戏开发更加简单。
代码实现与解析
导入必要的库
我们首先需要导入Pygame库和其他必要的模块:
import pygame
import math
import datetime
初始化Pygame
我们需要初始化Pygame并设置屏幕的基本参数:
pygame.init()
screen = pygame.display.set_mode((600, 600))
pygame.display.set_caption("动态钟表")
clock = pygame.time.Clock()
绘制钟表函数
我们定义几个函数来绘制钟表的各个部分:
def draw_circle(surface, color, center, radius):
pygame.draw.circle(surface, color, center, radius, 2)
def draw_hand(surface, color, center, angle, length, thickness):
end_pos = (center[0] + length * math.cos(angle), center[1] - length * math.sin(angle))
pygame.draw.line(surface, color, center, end_pos, thickness)
def draw_clock(screen):
now = datetime.datetime.now()
center = (300, 300)
radius = 200
# 绘制表盘
draw_circle(screen, (0, 0, 0), center, radius)
# 绘制时针
hour_angle = math.radians((now.hour % 12 + now.minute / 60) * 30 - 90)
draw_hand(screen, (0, 0, 0), center, hour_angle, 100, 8)
# 绘制分针
minute_angle = math.radians(now.minute * 6 - 90)
draw_hand(screen, (0, 0, 0), center, minute_angle, 150, 6)
# 绘制秒针
second_angle = math.radians(now.second * 6 - 90)
draw_hand(screen, (255, 0, 0), center, second_angle, 180, 2)
主循环
我们在主循环中更新钟表并绘制:
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255, 255, 255))
draw_clock(screen)
pygame.display.flip()
clock.tick(30)
pygame.quit()
完整代码
import pygame
import math
import datetime
# 初始化Pygame
pygame.init()
screen = pygame.display.set_mode((600, 600))
pygame.display.set_caption("动态钟表")
clock = pygame.time.Clock()
# 绘制钟表函数
def draw_circle(surface, color, center, radius):
pygame.draw.circle(surface, color, center, radius, 2)
def draw_hand(surface, color, center, angle, length, thickness):
end_pos = (center[0] + length * math.cos(angle), center[1] - length * math.sin(angle))
pygame.draw.line(surface, color, center, end_pos, thickness)
def draw_clock(screen):
now = datetime.datetime.now()
center = (300, 300)
radius = 200
# 绘制表盘
draw_circle(screen, (0, 0, 0), center, radius)
# 绘制时针
hour_angle = math.radians((now.hour % 12 + now.minute / 60) * 30 - 90)
draw_hand(screen, (0, 0, 0), center, hour_angle, 100, 8)
# 绘制分针
minute_angle = math.radians(now.minute * 6 - 90)
draw_hand(screen, (0, 0, 0), center, minute_angle, 150, 6)
# 绘制秒针
second_angle = math.radians(now.second * 6 - 90)
draw_hand(screen, (255, 0, 0), center, second_angle, 180, 2)
# 主循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255, 255, 255))
draw_clock(screen)
pygame.display.flip()
clock.tick(30)
pygame.quit()